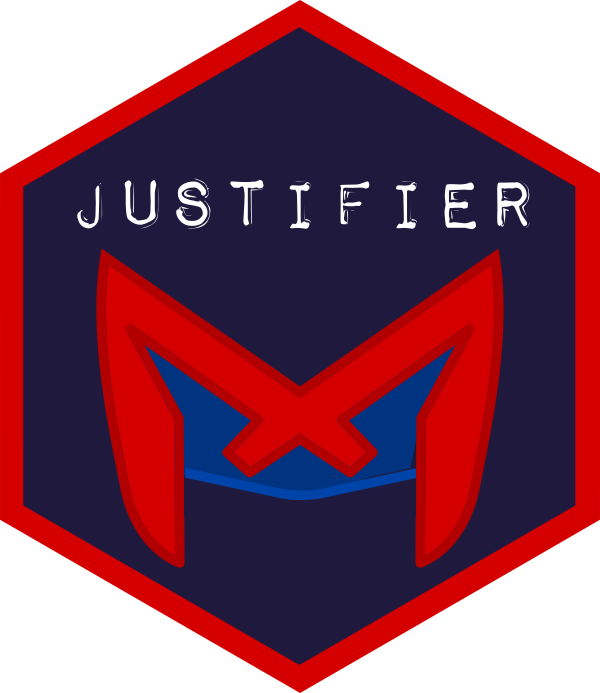
Load Justifications from a file or multiple files
Source:R/load_justifications.R
, R/load_justifications_dir.R
load_justifications.Rd
These function load justifications from the YAML fragments
in one (load_justifications
) or multiple files (load_justifications_dir
).
Usage
load_justifications(
text = NULL,
file = NULL,
delimiterRegEx = "^---$",
justificationContainer = c("justifier", "justification", "decision", "assertion",
"source"),
ignoreOddDelimiters = FALSE,
encoding = "UTF-8",
storeDecisionGraphSvg = TRUE,
silent = TRUE
)
load_justifications_dir(
path,
recursive = TRUE,
extension = "jmd",
regex = NULL,
justificationContainer = c("justifier", "justification", "decision", "assertion",
"source"),
delimiterRegEx = "^---$",
ignoreOddDelimiters = FALSE,
encoding = "UTF-8",
silent = TRUE
)
Arguments
- text, file
As
text
orfile
, you can specify afile
to read with encodingencoding
, which will then be read usingbase::readLines()
. If the argument is namedtext
, whether it is the path to an existing file is checked first, and if it is, that file is read. If the argument is namedfile
, and it does not point to an existing file, an error is produced (useful if calling from other functions). Atext
should be a character vector where every element is a line of the original source (like provided bybase::readLines()
); although if a character vector of one element and including at least one newline character (\\n
) is provided astext
, it is split at the newline characters usingbase::strsplit()
. Basically, this behavior means that the first argument can be either a character vector or the path to a file; and if you're specifying a file and you want to be certain that an error is thrown if it doesn't exist, make sure to name itfile
.- delimiterRegEx
The regular expression used to locate YAML fragments
- justificationContainer
The container of the justifications in the YAML fragments. Because only justifications are read that are stored in this container, the files can contain YAML fragments with other data, too, without interfering with the parsing of the justifications.
- ignoreOddDelimiters
Whether to throw an error (FALSE) or delete the last delimiter (TRUE) if an odd number of delimiters is encountered.
- encoding
The encoding to use when calling
readLines()
. Set to NULL to letreadLines()
guess.- storeDecisionGraphSvg
Whether to also produce (and return) the SVG for the decision graph.
- silent
Whether to be silent (TRUE) or informative (FALSE).
- path
The path containing the files to read.
- recursive
Whether to also process subdirectories (
TRUE
) or not (FALSE
).- extension
The extension of the files to read; files with other extensions will be ignored. Multiple extensions can be separated by a pipe (
|
).- regex
Instead of specifing an extension, it's also possible to specify a regular expression; only files matching this regular expression are read. If specified,
regex
takes precedece overextension
,
Value
An object with the ggplot2::ggplot graph stored
in output$graph
and the overview in output$overview
.
Details
load_justifications_dir
simply identifies all files and then calls
load_justifications
for each of them. load_justifications
loads the
YAML fragments containing the justifications using
yum::load_yaml_fragments()
and then parses the justifications
into a visual representation as a
ggplot2::ggplot graph and Markdown documents with
overviews.
Examples
exampleMinutes <- 'This is an example of minutes that include
a source, an assertion, and a justification. For example, in
the meeting, we can discuss the assertion that sleep deprivation
affects decision making. We could quickly enter this assertion in
a machine-readable way in this manner:
---
assertion:
-
id: assertion_SD_decision
label: Sleep deprivation affects the decision making proces.
source:
id: source_Harrison
---
Because it is important to refer to sources, we cite a source as well.
We have maybe specified that source elsewhere, for example in the
minutes of our last meeting. That specification may have looked
like this:
---
source:
-
id: source_Harrison
label: "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
xdoi: "doi:10.1037/1076-898x.6.3.236"
type: "Journal article"
---
We can now refer to these two specifications later on, for
example to justify decisions we take.
';
justifier::load_justifications(text=exampleMinutes);
#> $raw
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_decision"
#>
#> $assertion$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Harrison"
#>
#>
#>
#> $source
#> $source$id
#> [1] "source_Harrison"
#>
#> $source$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $source$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $source$type
#> [1] "Journal article"
#>
#>
#> attr(,"class")
#> [1] "simplifiedYum" "list"
#>
#> $structured
#> $structured$sources
#> $structured$sources$source_Harrison
#> $structured$sources$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $structured$sources$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $structured$sources$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $structured$sources$source_Harrison$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Harrison$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Harrison$fromDir
#> [1] "."
#>
#>
#>
#> $structured$assertions
#> $structured$assertions$assertion_SD_decision
#> $id
#> [1] "assertion_SD_decision"
#>
#> $label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $source
#> [1] "source_Harrison"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $fromFile
#> [1] "none"
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "assertion"
#>
#>
#> $structured$justifications
#> named list()
#>
#> $structured$decisions
#> named list()
#>
#> $structured$justifier
#> named list()
#>
#>
#> $logging
#> $logging$n_firstSweep
#> sources assertions justifications decisions justifier
#> 1 1 0 0 0
#>
#>
#> $frameworks
#> $frameworks$specs
#> list()
#>
#> $frameworks$loaded
#> list()
#>
#>
#> $fwApplications
#> list()
#>
#> $supplemented
#> $supplemented$sources
#> $supplemented$sources$source_Harrison
#> $supplemented$sources$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$sources$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$sources$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$sources$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Harrison$fromDir
#> [1] "."
#>
#>
#>
#> $supplemented$assertions
#> $supplemented$assertions$assertion_SD_decision
#> $id
#> [1] "assertion_SD_decision"
#>
#> $label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $source
#> $source$source_Harrison
#> $source$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $source$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $source$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $source$source_Harrison$type
#> [1] "Journal article"
#>
#> $source$source_Harrison$fromFile
#> [1] "none"
#>
#> $source$source_Harrison$fromDir
#> [1] "."
#>
#>
#>
#> $fromFile
#> [1] "none"
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "assertion"
#>
#>
#> $supplemented$justifications
#> named list()
#>
#> $supplemented$decisions
#> named list()
#>
#> $supplemented$justifier
#> named list()
#>
#>
#> $decisionTrees
#> named list()
#>
#> $decisionGraphs
#> named list()
#>
#> $decisionGraphsSvg
#> named list()
#>
#> attr(,"class")
#> [1] "justifications"
### To load a directory with justifications
examplePath <-
file.path(system.file(package="justifier"),
'extdata');
justifier::load_justifications_dir(path=examplePath);
#>
#>
#> Not all specifications of decisions, justifications, assertions, and sources successfully passed all verifications for framework '':
#>
#> - In the source with identifier `nice_round_numbers_are_nice`, when applying a verification in framework condition `source_year`, it failed with message: "The year you specify in a source must consist of exactly four digits.".
#>
#>
#> Not all specifications of decisions, justifications, assertions, and sources successfully passed all verifications for framework '':
#>
#> - In the source with identifier `nice_round_numbers_are_nice`, when applying a verification in framework condition `source_year`, it failed with message: "The year you specify in a source must consist of exactly four digits.".
#>
#>
#> All specifications of decisions, justifications, assertions, and sources successfully passed all verifications for framework ''.
#>
#> All specifications of decisions, justifications, assertions, and sources successfully passed all verifications for framework ''.
#> $raw
#> $decision
#> $decision$id
#> [1] "decision_1"
#>
#> $decision$label
#> [1] "Decision 1"
#>
#> $decision$description
#> [1] "Description of decision 1"
#>
#> $decision$justification
#> $decision$justification[[1]]
#> $decision$justification[[1]]$id
#> [1] "justification_1"
#>
#> $decision$justification[[1]]$label
#> [1] "Justification 1"
#>
#> $decision$justification[[1]]$description
#> [1] "Description of justification 1"
#>
#> $decision$justification[[1]]$assertion
#> $decision$justification[[1]]$assertion[[1]]
#> $decision$justification[[1]]$assertion[[1]]$id
#> [1] "assertion_1"
#>
#> $decision$justification[[1]]$assertion[[1]]$label
#> [1] "Assertion 1"
#>
#> $decision$justification[[1]]$assertion[[1]]$description
#> [1] "Description of assertion 1"
#>
#> $decision$justification[[1]]$assertion[[1]]$source
#> $decision$justification[[1]]$assertion[[1]]$source[[1]]
#> $decision$justification[[1]]$assertion[[1]]$source[[1]]$id
#> [1] "source_1"
#>
#> $decision$justification[[1]]$assertion[[1]]$source[[1]]$label
#> [1] "Source 1"
#>
#> $decision$justification[[1]]$assertion[[1]]$source[[1]]$xdoi
#> [1] "doi:10.0001/1000-0001.1.0.1"
#>
#> $decision$justification[[1]]$assertion[[1]]$source[[1]]$type
#> [1] "Journal article"
#>
#>
#> $decision$justification[[1]]$assertion[[1]]$source[[2]]
#> $decision$justification[[1]]$assertion[[1]]$source[[2]]$id
#> [1] "source_2"
#>
#> $decision$justification[[1]]$assertion[[1]]$source[[2]]$label
#> [1] "Source 2"
#>
#> $decision$justification[[1]]$assertion[[1]]$source[[2]]$xdoi
#> [1] "doi:10.0002/2000-0002.2.0.2"
#>
#> $decision$justification[[1]]$assertion[[1]]$source[[2]]$type
#> [1] "Journal article"
#>
#>
#>
#>
#> $decision$justification[[1]]$assertion[[2]]
#> $decision$justification[[1]]$assertion[[2]]$id
#> [1] "assertion_2"
#>
#> $decision$justification[[1]]$assertion[[2]]$label
#> [1] "Assertion 2"
#>
#> $decision$justification[[1]]$assertion[[2]]$description
#> [1] "Description of assertion 2"
#>
#> $decision$justification[[1]]$assertion[[2]]$source
#> $decision$justification[[1]]$assertion[[2]]$source$id
#> [1] "source_3"
#>
#> $decision$justification[[1]]$assertion[[2]]$source$label
#> [1] "Source 3"
#>
#> $decision$justification[[1]]$assertion[[2]]$source$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $decision$justification[[1]]$assertion[[2]]$source$type
#> [1] "Journal article"
#>
#>
#>
#>
#> $decision$justification[[1]]$justification
#> $decision$justification[[1]]$justification$id
#> [1] "justification_1b"
#>
#> $decision$justification[[1]]$justification$label
#> [1] "Justification 1b"
#>
#> $decision$justification[[1]]$justification$description
#> [1] "Description of justification 1b"
#>
#>
#>
#> $decision$justification[[2]]
#> $decision$justification[[2]]$id
#> [1] "justification_2"
#>
#> $decision$justification[[2]]$label
#> [1] "Justification 2"
#>
#> $decision$justification[[2]]$description
#> [1] "Description of justification 2"
#>
#> $decision$justification[[2]]$assertion
#> [1] "assertion_2"
#>
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/complex-example.jmd"
#>
#>
#> $decision
#> $decision$id
#> [1] "decision_2"
#>
#> $decision$label
#> [1] "Decision 2"
#>
#> $decision$description
#> [1] "Description of decision 2"
#>
#> $decision$alternatives
#> $decision$alternatives[[1]]
#> $decision$alternatives[[1]]$value
#> [1] 1
#>
#> $decision$alternatives[[1]]$label
#> [1] "Alternative 1"
#>
#> $decision$alternatives[[1]]$description
#> [1] "Description of alternative 1"
#>
#>
#> $decision$alternatives[[2]]
#> $decision$alternatives[[2]]$value
#> [1] 2
#>
#> $decision$alternatives[[2]]$label
#> [1] "Alternative 2"
#>
#> $decision$alternatives[[2]]$description
#> [1] "Description of alternative 2"
#>
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/complex-example.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "decision1"
#>
#> $justification$`project-id`
#> [1] "wim"
#>
#> $justification$name
#> [1] "last finalization study recruitment"
#>
#> $justification$date
#> [1] "2018-09-12"
#>
#> $justification$actors
#> [1] "Person, First" "Person, Second"
#>
#> $justification$description
#> [1] "We decided to use snowball sampling."
#>
#> $justification$alternatives
#> [1] "only invite people we know" "cast a wide net"
#>
#> $justification$choice
#> [1] 2
#>
#> $justification$justification
#> [1] "more inclusive"
#>
#> $justification$specifications
#> NULL
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/example-minutes.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "decision2"
#>
#> $justification$`project-id`
#> [1] "wim"
#>
#> $justification$name
#> [1] "do list finalization study"
#>
#> $justification$date
#> [1] "2018-09-12"
#>
#> $justification$actors
#> [1] "Kok, Gerjo" "Peters, Louk" "Crutzen, Rik"
#> [4] "Peters, Gjalt-Jorn"
#>
#> $justification$description
#> [1] "We decided to blablabla"
#>
#> $justification$alternatives
#> [1] "no" "yes"
#>
#> $justification$choice
#> [1] 2
#>
#> $justification$justification
#> [1] "more thorough"
#>
#> $justification$specifications
#> NULL
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/example-minutes.jmd"
#>
#>
#> $justifier
#> $justifier$id
#> [1] "justifier_example_study_framework_specification"
#>
#> $justifier$label
#> [1] "Justifier example: Study framework specification"
#>
#> $justifier$description
#> [1] "This framework is an example of how different decisions in the planning of a psychological study could be contextualized."
#>
#> $justifier$context
#> $justifier$context[[1]]
#> $justifier$context[[1]]$id
#> [1] "global_planning"
#>
#> $justifier$context[[1]]$importedContextFramework
#> [1] "some_other_framework_specification"
#>
#> $justifier$context[[1]]$importedContextId
#> [1] "global_planning"
#>
#> $justifier$context[[1]]$sequence
#> [1] 1
#>
#>
#> $justifier$context[[2]]
#> $justifier$context[[2]]$id
#> [1] "design"
#>
#> $justifier$context[[2]]$sequence
#> [1] 2
#>
#> $justifier$context[[2]]$label
#> [1] "Decisions related to design"
#>
#>
#> $justifier$context[[3]]
#> $justifier$context[[3]]$id
#> [1] "sample_size"
#>
#> $justifier$context[[3]]$sequence
#> [1] 3
#>
#>
#> $justifier$context[[4]]
#> $justifier$context[[4]]$id
#> [1] "operationalizations"
#>
#> $justifier$context[[4]]$sequence
#> [1] 4
#>
#>
#>
#> $justifier$condition
#> $justifier$condition[[1]]
#> $justifier$condition[[1]]$id
#> [1] "global_method_specification"
#>
#> $justifier$condition[[1]]$contextId
#> [1] "global_planning"
#>
#> $justifier$condition[[1]]$type
#> [1] "global_method_specification"
#>
#> $justifier$condition[[1]]$element
#> [1] "decision"
#>
#> $justifier$condition[[1]]$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $justifier$condition[[1]]$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#> $justifier$condition[[2]]
#> $justifier$condition[[2]]$id
#> [1] "study_sample_size"
#>
#> $justifier$condition[[2]]$contextId
#> [1] "sample_size"
#>
#> $justifier$condition[[2]]$type
#> [1] "study_sample_size"
#>
#> $justifier$condition[[2]]$element
#> [1] "decision"
#>
#> $justifier$condition[[2]]$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#> $justifier$condition[[3]]
#> $justifier$condition[[3]]$id
#> [1] "included_variables"
#>
#> $justifier$condition[[3]]$contextid
#> [1] "design"
#>
#> $justifier$condition[[3]]$type
#> [1] "included_variables"
#>
#> $justifier$condition[[3]]$element
#> [1] "decision"
#>
#>
#> $justifier$condition[[4]]
#> $justifier$condition[[4]]$id
#> [1] "source_types"
#>
#> $justifier$condition[[4]]$element
#> [1] "source"
#>
#> $justifier$condition[[4]]$field
#> [1] "evidence_type"
#>
#> $justifier$condition[[4]]$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $justifier$condition[[4]]$scores
#> [1] 1 2 3 4 5 6
#>
#> $justifier$condition[[4]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#>
#> $justifier$condition[[5]]
#> $justifier$condition[[5]]$id
#> [1] "source_year"
#>
#> $justifier$condition[[5]]$element
#> [1] "source"
#>
#> $justifier$condition[[5]]$field
#> [1] "year"
#>
#> $justifier$condition[[5]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $justifier$condition[[5]]$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#>
#>
#> $justifier$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/justifier-example-study-framework-specification.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Diekelmann"
#>
#> $source$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $source$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Gais"
#>
#> $source$label
#> [1] "Gais (2003) Sleep after learning aids memory recall."
#>
#> $source$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Menz"
#>
#> $source$label
#> [1] "Menz et al (2013) The role of sleep and sleep deprivation in consolidating fear memories."
#>
#> $source$xdoi
#> [1] "doi:10.1016/j.neuroimage.2013.03.001"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Holland"
#>
#> $source$label
#> [1] "Holland & Lewis (2007) Emotional memory: selective enhancement by sleep."
#>
#> $source$xdoi
#> [1] "doi:10.1016/j.cub.2006.12.033"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Graves"
#>
#> $source$label
#> [1] "Graves (2003) Sleep deprivation selectively impairs memory consolidation for contextual fear conditioning."
#>
#> $source$xdoi
#> [1] "doi:10.1101/lm.48803"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Frey"
#>
#> $source$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $source$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Xie"
#>
#> $source$label
#> [1] "Zie et al (2013) ). Sleep drives metabolite clearance from the adult brain."
#>
#> $source$xdoi
#> [1] "doi:10.1126/science.1241224"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Brokaw"
#>
#> $source$label
#> [1] "Brokaw et al (2016) Resting state EEG correlates of memory consolidation."
#>
#> $source$xdoi
#> [1] "doi:10.1016/j.nlm.2016.01.008"
#>
#> $source$pp
#> [1] "17-25"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Marrosu"
#>
#> $source$label
#> [1] "Marrosu et al (1995) Microdialysis measurement of cortical and hippocampal acetylcholine release during sleep-wake cycle in freely moving cats."
#>
#> $source$xdoi
#> [1] "No DOI found yet, located in: Brain Research, vol 671, pp 329–332."
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Lange"
#>
#> $source$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $source$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Burgess"
#>
#> $source$label
#> [1] "Burgess et al (2002) ). Bright light, dark and melatonin can promote circadian adaptation in night shift workers."
#>
#> $source$xdoi
#> [1] "doi:10.1053/smrv.2001.0215"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Plog"
#>
#> $source$label
#> [1] "Plog & Nedergaard (2018) The glymphatic system in central nervous system health and disease: past, present, and future"
#>
#> $source$xdoi
#> [1] "doi:10.1146/annurev-pathol-051217-111018"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Killgore13"
#>
#> $source$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $source$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Harrison"
#>
#> $source$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $source$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Doran"
#>
#> $source$label
#> [1] "Doran et al (2001) Sustained attention performance during sleep deprivation: evidence of state instability."
#>
#> $source$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Killgore07"
#>
#> $source$label
#> [1] "Killgore et al (2007) The trait of Introversion–Extraversion predicts vulnerability to sleep deprivation."
#>
#> $source$xdoi
#> [1] "doi:10.1111/j.1365-2869.2007.00611.x"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_VanDongen"
#>
#> $source$label
#> [1] "Van Dongen et al (2003) Sleep debt: Theoretical and empirical issues."
#>
#> $source$comment
#> [1] "No DOI found yet; located in Sleep and Biological Rhythms, vol 1, pp 5–13"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Kuypers"
#>
#> $source$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $source$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Williamson"
#>
#> $source$label
#> [1] "Williamson & Feyer (2000) Moderate sleep deprivation produces impairments in cognitive and motor performance equivalent to legally prescribed levels of alcohol intoxication."
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Peeke"
#>
#> $source$label
#> [1] "Peeke et al (1980) Combined effects of alcohol and sleep deprivation in normal young adults."
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Croes"
#>
#> $source$label
#> [1] "Croes et al (2017) Langdurige klachten na ecstasygebruik."
#>
#> $source$xdoi
#> [1] "url:www.trimbos.nl/docs/2c1748e6-93d5-481b-8fad-013f91a9e1df.pdf"
#>
#> $source$type
#> [1] "Report"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Berger"
#>
#> $source$label
#> [1] "Energy conservation and sleep."
#>
#> $source$xdoi
#> [1] "doi:10.1016/0166-4328(95)00002-b"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Fairclough"
#>
#> $source$label
#> [1] "Fairclough & Graham (1999) Impairment of driving performance caused by sleep deprivation or alcohol: A comparative Study. "
#>
#> $source$xdoi
#> [1] "doi:10.1518/001872099779577336"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Krueger"
#>
#> $source$label
#> [1] "Kreueger et al (2006) The role of cytokines in physiological sleep regulation"
#>
#> $source$xdoi
#> [1] "doi:10.1111/j.1749-6632.2001.tb05826.x"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Jessen"
#>
#> $source$label
#> [1] "Jessen et al (2015) The Glymphatic System: A Beginner’s Guide."
#>
#> $source$xdoi
#> [1] "doi:10.1007/s11064-015-1581-6"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Lim"
#>
#> $source$label
#> [1] "Lim & Dinges (2010) A meta-analysis of the impact of short-term sleep deprivation on cognitive variables."
#>
#> $source$xdoi
#> [1] "doi:10.1037/a0018883"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Tononi"
#>
#> $source$label
#> [1] "Tononi & Cirelli (2006) ). Sleep function and synaptic homeostasis."
#>
#> $source$xdoi
#> [1] "doi:10.1016/j.smrv.2005.05.002"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Beebe"
#>
#> $source$label
#> [1] "Beebe et al (2010). Attention, learning, and arousal of experimentally sleep-restricted adolescents in a simulated classroom"
#>
#> $source$xdoi
#> [1] "doi:10.1016/j.jadohealth.2010.03.005"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_memory_1"
#>
#> $assertion$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Diekelmann"
#>
#> $assertion$source$comment
#> [1] "test of a comment"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_memory_2"
#>
#> $assertion$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Gais"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_memory_3"
#>
#> $assertion$label
#> [1] "Sleep promotes the consolidation of fear memory in humans."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Menz"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_memory_4"
#>
#> $assertion$label
#> [1] "Sleep promotes the consolidation of emotional memory in humans."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Holland"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_memory_5"
#>
#> $assertion$label
#> [1] "Sleep promotes the consolidation of memory in animals after training for a specific task."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Graves"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_memory_6"
#>
#> $assertion$label
#> [1] "Memory retention is already noticable after only several minutes of sleep."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Diekelmann"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_cytokines"
#>
#> $assertion$label
#> [1] "Infection, stress and tissue damage triggers the release of inflammatory cytokines."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Frey"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_cytokines_function"
#>
#> $assertion$label
#> [1] "When pro-inflammatory cytokines are injected, these cytokines enhance sleep."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Krueger"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_glympathic_system"
#>
#> $assertion$label
#> [1] "Sleep promotes the glympathic system."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Xie"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_cytokines"
#>
#> $assertion$label
#> [1] "Sleep deprivation is associated with an increase of pro-inflammatory cytokines, which creates a disbalance of inflammatory cytokines and this induces inflammation."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Frey"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_resting_1"
#>
#> $assertion$label
#> [1] "Resting enhances the consolidation of memory."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Brokaw"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_resting_2"
#>
#> $assertion$label
#> [1] "The neurotransmitter ACh is released during quiet rest, just like during sleep."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Marrosu"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_nocturnal_1"
#>
#> $assertion$label
#> [1] "Cells like leukocytes show a specific diurnal or nocturnal rhythm."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Lange"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_nocturnal_2"
#>
#> $assertion$label
#> [1] "During nocturnal sleep cell counts of toxic cells are suppressed."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Lange"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_sleep_debt"
#>
#> $assertion$label
#> [1] "Sleep deprivation results in a sleep debt, which has unhealthy consequences."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Burgess"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_glympathic_system_2"
#>
#> $assertion$label
#> [1] "Failure of the glympathic system is associated with Alzheimer's disease."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Plog"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_glympathic_system_1"
#>
#> $assertion$label
#> [1] "The glympathic system is a recently discovered waste clearance pathway that removes metabolites and toxic proteins from the brain."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Jessen"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_performance"
#>
#> $assertion$label
#> [1] "(Cognitive) performance deteriorates after 16 hours of wakefulness."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Killgore13"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_sensory_perception"
#>
#> $assertion$label
#> [1] "Sleep loss causes a reduction in visual cortex activity and the reduction is most prominent when an individual experiences an attentional lapse."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Killgore13"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_inattentive_behaviours"
#>
#> $assertion$label
#> [1] "sleep deprived individuals showed significantly more inattentive behaviours compared to non-sleep deprived individuals while watching a movie."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Beebe"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_decision"
#>
#> $assertion$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Harrison"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_state_instability"
#>
#> $assertion$label
#> [1] "After sleep deprivation performance becomes instable, which is called state instability."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Doran"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_emotion_1"
#>
#> $assertion$label
#> [1] "Sleep loss enhances the strenth of reactions to negative, but not to positive or neutral stimuli."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Killgore13"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_emotion_2"
#>
#> $assertion$label
#> [1] "Humor is negatively evaluated and emotional expression is lost in the voice after sleep deprivation."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Killgore13"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_individual_differences_1"
#>
#> $assertion$label
#> [1] "The personality trait extraversion is more related to attentional lapses and more extensive declines in speed response during a task after one night of sleep deprivation."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Killgore07"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_individual_differences_2"
#>
#> $assertion$label
#> [1] "There is a genetic predisposition for vulnerability to sleep loss."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_VanDongen"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_MDMA_impulse_daytime"
#>
#> $assertion$label
#> [1] "When MDMA is taken during daytime, impulse control is enhanced."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Kuypers"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_MDMA_impulse_nighttime"
#>
#> $assertion$label
#> [1] "When MDMA is taken during nighttime, impulse control is not enhanced."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Kuypers"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_MDMA_PMF_daytime"
#>
#> $assertion$label
#> [1] "When MDMA is taken during daytime psychomotor functioning is enhanced."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Kuypers"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_MDMA_PMF_nighttime"
#>
#> $assertion$label
#> [1] "When MDMA is taken during nighttime psychomotor functioning is impaired."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Kuypers"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_alcohol"
#>
#> $assertion$label
#> [1] "Sleep deprivation causes cognitive and motor performance impairment similar to alcohol intoxication."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Williamson"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_interaction_SD_alcohol_1"
#>
#> $assertion$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived react slower than people who are either are under the influence of alcohol or sleep deprived alone."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Peeke"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_interaction_SD_alcohol_2"
#>
#> $assertion$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived show worse driving performance than people who either are under the influence of alcohol or sleep deprived alone."
#>
#> $assertion$comment
#> [1] "this source was 'Howard'. But the one that was named 'Howard', I renamed to 'Harrison'. Smth wrong here?"
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Harrison"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_MDMA_cortisol"
#>
#> $assertion$label
#> [1] "Cortisol levels rise after MDMA is taken."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Croes"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_resting_3"
#>
#> $assertion$label
#> [1] "Important functions of sleep, like recovery of neural function and synaptic downscaling to save energy and experience benefits for learning and memory, only occur together with slow wave sleep and therefore not while resting."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Tononi"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_attention"
#>
#> $assertion$label
#> [1] "The most simple form of attention, that is being able to detect a stimulus, is most strongly affected by sleep deprivation."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Lim"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_01"
#>
#> $justification$label
#> [1] "Sleep prevents us from getting sick and (therefore) sleep deprivation causes an impaired immune system. Sleep prevents a disbalance of inflammatory cytokines and therefore health is maintained."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_cytokines" "assertion_cytokines_function"
#> [3] "assertion_SD_cytokines"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_02"
#>
#> $justification$label
#> [1] "Sleep is important for our memory and (therefore) sleep deprivation causes memory impairment."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_sleep_memory_1" "assertion_sleep_memory_2"
#> [3] "assertion_sleep_memory_3" "assertion_sleep_memory_4"
#> [5] "assertion_sleep_memory_5" "assertion_sleep_memory_6"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_03"
#>
#> $justification$label
#> [1] "Sleep protects us from toxicants and (therefore) sleep deprivation is associated with neurological diseases."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_glympathic_system_1" "assertion_glympathic_system_2"
#> [3] "assertion_sleep_glympathic_system"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_04"
#>
#> $justification$label
#> [1] "Resting is not as beneficial as sleep, but is preferred over total sleep deprivation as resting state resembles a sleep state."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_resting_1" "assertion_resting_2" "assertion_resting_3"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_05"
#>
#> $justification$label
#> [1] "Nocturnal sleep is preferred over diurnal sleep, but diurnal sleep is preferred over total sleep deprivation."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_nocturnal_1" "assertion_nocturnal_2" "assertion_sleep_debt"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_06"
#>
#> $justification$label
#> [1] "Sleep deprivation causes impaired cognitive performance."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_SD_performance" "assertion_attention"
#> [3] "assertion_inattentive_behaviours"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_07"
#>
#> $justification$label
#> [1] "Sleep deprivation causes impaired decision making."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_SD_decision"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_08"
#>
#> $justification$label
#> [1] "Sleep deprivation causes state instability."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_state_instability"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_09"
#>
#> $justification$label
#> [1] "Sleep deprivation causes impaired sensory perception."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_SD_sensory_perception"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_10"
#>
#> $justification$label
#> [1] "Sleep deprivation affects emotional perception and experience."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_SD_emotion_1" "assertion_SD_emotion_2"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_11"
#>
#> $justification$label
#> [1] "There are individual differences in the effect sleep deprivation has on people."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_individual_differences_1" "assertion_individual_differences_2"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_12"
#>
#> $justification$label
#> [1] "There is an interaction between sleep deprivation and MDMA."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_MDMA_PMF_nighttime" "assertion_MDMA_PMF_daytime"
#> [3] "assertion_MDMA_impulse_nighttime" "assertion_MDMA_impulse_daytime"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $justification
#> $justification$id
#> [1] "justification_13"
#>
#> $justification$label
#> [1] "There is an interaction between sleep deprivation and alcohol."
#>
#> $justification$assertion
#> $justification$assertion$id
#> [1] "assertion_SD_alcohol" "assertion_interaction_SD_alcohol_1"
#> [3] "assertion_interaction_SD_alcohol_2"
#>
#>
#> $justification$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $decision
#> $decision$id
#> [1] "decision_to_select_behavior_1"
#>
#> $decision$label
#> [1] "People should do so and so because of justifications 04,05,07,10 etc."
#>
#> $decision$justification
#> $decision$justification$id
#> [1] "justification_04" "justification_05" "justification_07" "justification_10"
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $decision
#> $decision$id
#> [1] "decision_to_select_behavior_2"
#>
#> $decision$label
#> [1] "People should do so and so because of"
#>
#> $decision$justification
#> $decision$justification$id
#> [1] "justification_01" "justification_02"
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/pp19.1-target-behavior-selection.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "assertion_SD_decision"
#>
#> $assertion$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "source_Harrison"
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/simple-example.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "source_Harrison"
#>
#> $source$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $source$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $source$type
#> [1] "Journal article"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/simple-example.jmd"
#>
#>
#> $justifier
#> $justifier$scope
#> [1] "global"
#>
#> $justifier$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $justifier$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/study-example.jmd"
#>
#>
#> $justifier
#> $justifier$scope
#> [1] "local"
#>
#> $justifier$date
#> [1] "2019-03-06"
#>
#> $justifier$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/study-example.jmd"
#>
#>
#> $decision
#> $decision$type
#> [1] "research_question"
#>
#> $decision$id
#> [1] "research_question"
#>
#> $decision$value
#> [1] "What are the most important determinants of getting one's ecstasy tested?"
#>
#> $decision$label
#> [1] "The answer to this research question is required to develop and effective interventions to promote ecstasy pill testing."
#>
#> $decision$description
#> [1] "To minimize the likelihood of incidents and accidental intoxication, testing is a required step."
#>
#> $decision$justification
#> $decision$justification[[1]]
#> $decision$justification[[1]]$id
#> [1] "without_testing_users_may_ingest_contaminants"
#>
#> $decision$justification[[1]]$label
#> [1] "Some XTC pills are contaminated, and one needs to test them to be aware of the pill contents."
#>
#> $decision$justification[[1]]$assertion
#> $decision$justification[[1]]$assertion[[1]]
#> $decision$justification[[1]]$assertion[[1]]$id
#> [1] "some_pills_are_contaminated"
#>
#> $decision$justification[[1]]$assertion[[1]]$source
#> [1] "vogels_2009"
#>
#> $decision$justification[[1]]$assertion[[1]]$label
#> [1] "Not all produced XTC pills contain only MDMA as active ingredient."
#>
#> $decision$justification[[1]]$assertion[[1]]$evidence_type
#> [1] "empirical study"
#>
#>
#>
#>
#> $decision$justification[[2]]
#> $decision$justification[[2]]$id
#> [1] "proper_dosing_requires_knowing_dose"
#>
#> $decision$justification[[2]]$label
#> [1] "Proper dosing (~ 1-1.5 mg of MDMA per kg of body weight) becomes hard if the dose in a pill is unknown."
#>
#> $decision$justification[[2]]$assertion
#> $decision$justification[[2]]$assertion$id
#> [1] "dosing_requires_pill_content"
#>
#> $decision$justification[[2]]$assertion$label
#> [1] "Determining the dose of MDMA one uses requires knowing both one's body weight and the pill dose."
#>
#> $decision$justification[[2]]$assertion$source
#> $decision$justification[[2]]$assertion$source$id
#> [1] "brunt_2012"
#>
#> $decision$justification[[2]]$assertion$source$label
#> [1] "Brunt, Koeter, Niesink & van den Brink (2012)"
#>
#> $decision$justification[[2]]$assertion$source$evidence_type
#> [1] "empirical study"
#>
#>
#>
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/study-example.jmd"
#>
#>
#> $decision
#> $decision$type
#> [1] "global_study_method"
#>
#> $decision$id
#> [1] "global_study_method"
#>
#> $decision$value
#> [1] "quantitative"
#>
#> $decision$label
#> [1] "We will conduct a quantitative study."
#>
#> $decision$description
#> [1] "Here, a decision can be explained more in detail (e.g. describing how the justifications relate to each other)."
#>
#> $decision$justification
#> $decision$justification[[1]]
#> $decision$justification[[1]]$id
#> [1] "enough_known_about_testing_determinants"
#>
#> $decision$justification[[1]]$label
#> [1] "We have enough information available to develop a questionnaire that is likely to measure the most important determinants and sub-determinants of XTC testing."
#>
#> $decision$justification[[1]]$assertion
#> $decision$justification[[1]]$assertion[[1]]
#> $decision$justification[[1]]$assertion[[1]]$id
#> [1] "testing_xtc_is_reasoned"
#>
#> $decision$justification[[1]]$assertion[[1]]$source
#> [1] "phd_peters_2008"
#>
#> $decision$justification[[1]]$assertion[[1]]$label
#> [1] "Previous research indicates that getting one's ecstasy tested (or not) is largely a reasoned behavior"
#>
#>
#> $decision$justification[[1]]$assertion[[2]]
#> $decision$justification[[1]]$assertion[[2]]$id
#> [1] "there_is_qualitative_research_about_testing"
#>
#> $decision$justification[[1]]$assertion[[2]]$source
#> [1] "phd_peters_2008"
#>
#> $decision$justification[[1]]$assertion[[2]]$label
#> [1] "There exists qualitative research about why people get their ecstasy tested"
#>
#>
#>
#>
#> $decision$justification[[2]]
#> $decision$justification[[2]]$id
#> [1] "limited_time"
#>
#> $decision$justification[[2]]$label
#> [1] "We have to finish this study before the end of 2019."
#>
#> $decision$justification[[2]]$assertion
#> $decision$justification[[2]]$assertion[[1]]
#> $decision$justification[[2]]$assertion[[1]]$id
#> [1] "project_deadline"
#>
#> $decision$justification[[2]]$assertion[[1]]$label
#> [1] "The deadline for this project is december 2019."
#>
#> $decision$justification[[2]]$assertion[[1]]$source
#> $decision$justification[[2]]$assertion[[1]]$source$id
#> [1] "project_proposal"
#>
#> $decision$justification[[2]]$assertion[[1]]$source$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $decision$justification[[2]]$assertion[[1]]$source$year
#> [1] 2017
#>
#>
#>
#>
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/study-example.jmd"
#>
#>
#> $decision
#> $decision$id
#> [1] "study_sample_size"
#>
#> $decision$type
#> [1] "study_sample_size"
#>
#> $decision$value
#> [1] 400
#>
#> $decision$label
#> [1] "We aim to recruit around 400 participants."
#>
#> $decision$justification
#> $decision$justification[[1]]
#> $decision$justification[[1]]$id
#> [1] "aipe_for_correlations"
#>
#> $decision$justification[[1]]$label
#> [1] "To estimate a correlation accurately, you need ~ 400 participants."
#>
#> $decision$justification[[1]]$assertion
#> $decision$justification[[1]]$assertion[[1]]
#> $decision$justification[[1]]$assertion[[1]]$id
#> [1] "nice_round_number"
#>
#> $decision$justification[[1]]$assertion[[1]]$label
#> [1] "We want to recruit a nice round number of participants."
#>
#> $decision$justification[[1]]$assertion[[1]]$source
#> $decision$justification[[1]]$assertion[[1]]$source$id
#> [1] "nice_round_numbers_are_nice"
#>
#> $decision$justification[[1]]$assertion[[1]]$source$year
#> [1] 219
#>
#> $decision$justification[[1]]$assertion[[1]]$source$label
#> [1] "In our meeting of 2019-07-05, we agreed that all team members like nice round numbers."
#>
#> $decision$justification[[1]]$assertion[[1]]$source$evidence_type
#> [1] "team opinion"
#>
#>
#>
#> $decision$justification[[1]]$assertion[[2]]
#> $decision$justification[[1]]$assertion[[2]]$id
#> [1] "exact_aipe_for_our_study"
#>
#> $decision$justification[[1]]$assertion[[2]]$label
#> [1] "Table 1 shows that 383 participants are sufficient even for correlations as low as .05."
#>
#> $decision$justification[[1]]$assertion[[2]]$source
#> $decision$justification[[1]]$assertion[[2]]$source$id
#> [1] "moinester_gottfried_2014"
#>
#> $decision$justification[[1]]$assertion[[2]]$source$year
#> [1] 2014
#>
#> $decision$justification[[1]]$assertion[[2]]$source$label
#> [1] "Moinester, M., & Gottfried, R. (2014). Sample size estimation for correlations with pre-specified confidence interval. The Quantitative Methods of Psychology, 10(2), 124–130."
#>
#> $decision$justification[[1]]$assertion[[2]]$source$evidence_type
#> [1] "theory"
#>
#>
#>
#>
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/study-example.jmd"
#>
#>
#> $decision
#> $decision$id
#> [1] "preregistration"
#>
#> $decision$type
#> [1] "preregistration"
#>
#> $decision$value
#> [1] FALSE
#>
#> $decision$label
#> [1] "We will not preregister this study."
#>
#> $decision$justification
#> $decision$justification[[1]]
#> $decision$justification[[1]]$id
#> [1] "low_risk_of_bias"
#>
#> $decision$justification[[1]]$label
#> [1] "Because we do not test a specific hypothesis but simply want to know how relevant the different determinants are in this population, the need to preregister is less pressing."
#>
#> $decision$justification[[1]]$assertion
#> $decision$justification[[1]]$assertion[[1]]
#> $decision$justification[[1]]$assertion[[1]]$id
#> [1] "prereg_to_decrease_bias"
#>
#> $decision$justification[[1]]$assertion[[1]]$label
#> [1] "Preregistration_decreases_bias"
#>
#> $decision$justification[[1]]$assertion[[1]]$source
#> $decision$justification[[1]]$assertion[[1]]$source$id
#> [1] "example_source"
#>
#> $decision$justification[[1]]$assertion[[1]]$source$label
#> [1] "Not online now, but a study making this point would be nice here"
#>
#> $decision$justification[[1]]$assertion[[1]]$source$evidence_type
#> [1] "empirical study"
#>
#>
#>
#>
#>
#> $decision$justification[[2]]
#> $decision$justification[[2]]$id
#> [1] "no_time_for_prereg"
#>
#> $decision$justification[[2]]$label
#> [1] "We have no time to complete a preregistration form."
#>
#> $decision$justification[[2]]$assertion
#> $decision$justification[[2]]$assertion$id
#> [1] "project_deadline"
#>
#>
#>
#>
#> $decision$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/study-example.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "phd_peters_xtc_determinants"
#>
#> $source$label
#> [1] "Peters, G. J. Y. (2008) Determinants of ecstasy use and harm reduction strategies: informing evidence-based intervention development. https://phdthesis.nl"
#>
#> $source$xdoi
#> [1] "isbn:9781409240716"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/xtc-non-users.jmd"
#>
#>
#> $source
#> $source$id
#> [1] "phd_vervaeke_xtc_social"
#>
#> $source$label
#> [1] "Vervaeke, H. K. E. (2009) Initiation and continuation: social context and behavioural aspects of ecstasy use"
#>
#> $source$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/xtc-non-users.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "tryout_determinants_ranking_intention"
#>
#> $assertion$label
#> [1] "Intention to try out XTC is an extremely strong predictor of trying out XTC (Cohen's d > 1, OR > 7)"
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "src:phd_peters_determinant_xtc_use"
#>
#> $assertion$source$pp
#> [1] 91
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/xtc-non-users.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "tryout_determinants_ranking_attitude"
#>
#> $assertion$label
#> [1] "Attitude is the strongest predictor of intention to try out XTC (r = .67)"
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "src:phd_peters_determinant_xtc_use"
#>
#> $assertion$source$pp
#> [1] 91
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/xtc-non-users.jmd"
#>
#>
#> $assertion
#> $assertion$id
#> [1] "notry_determinants_ranking_othersubstance"
#>
#> $assertion$label
#> [1] "Already using some other substance is the strongest 'deterrent' of trying out ecstasy"
#>
#> $assertion$source
#> $assertion$source$id
#> [1] "src:phd_vervaeke_xtc_social"
#>
#> $assertion$source$pp
#> [1] 36
#>
#>
#> $assertion$fromFile
#> [1] "/tmp/RtmpQqVTea/temp_libpath1a0c4f1b2af41/justifier/extdata/xtc-non-users.jmd"
#>
#>
#> attr(,"class")
#> [1] "simplifiedYum" "list"
#>
#> $structured
#> $structured$sources
#> $structured$sources$source_Diekelmann
#> $structured$sources$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $structured$sources$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $structured$sources$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $structured$sources$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $structured$sources$source_Diekelmann$fromDir
#> [1] "."
#>
#> $structured$sources$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Gais
#> $structured$sources$source_Gais$id
#> [1] "source_Gais"
#>
#> $structured$sources$source_Gais$label
#> [1] "Gais (2003) Sleep after learning aids memory recall."
#>
#> $structured$sources$source_Gais$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $structured$sources$source_Gais$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Gais$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Gais$fromDir
#> [1] "."
#>
#> $structured$sources$source_Gais$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Gais$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Menz
#> $structured$sources$source_Menz$id
#> [1] "source_Menz"
#>
#> $structured$sources$source_Menz$label
#> [1] "Menz et al (2013) The role of sleep and sleep deprivation in consolidating fear memories."
#>
#> $structured$sources$source_Menz$xdoi
#> [1] "doi:10.1016/j.neuroimage.2013.03.001"
#>
#> $structured$sources$source_Menz$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Menz$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Menz$fromDir
#> [1] "."
#>
#> $structured$sources$source_Menz$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Menz$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Holland
#> $structured$sources$source_Holland$id
#> [1] "source_Holland"
#>
#> $structured$sources$source_Holland$label
#> [1] "Holland & Lewis (2007) Emotional memory: selective enhancement by sleep."
#>
#> $structured$sources$source_Holland$xdoi
#> [1] "doi:10.1016/j.cub.2006.12.033"
#>
#> $structured$sources$source_Holland$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Holland$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Holland$fromDir
#> [1] "."
#>
#> $structured$sources$source_Holland$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Holland$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Graves
#> $structured$sources$source_Graves$id
#> [1] "source_Graves"
#>
#> $structured$sources$source_Graves$label
#> [1] "Graves (2003) Sleep deprivation selectively impairs memory consolidation for contextual fear conditioning."
#>
#> $structured$sources$source_Graves$xdoi
#> [1] "doi:10.1101/lm.48803"
#>
#> $structured$sources$source_Graves$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Graves$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Graves$fromDir
#> [1] "."
#>
#> $structured$sources$source_Graves$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Graves$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Frey
#> $structured$sources$source_Frey$id
#> [1] "source_Frey"
#>
#> $structured$sources$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $structured$sources$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $structured$sources$source_Frey$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Frey$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Frey$fromDir
#> [1] "."
#>
#> $structured$sources$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Xie
#> $structured$sources$source_Xie$id
#> [1] "source_Xie"
#>
#> $structured$sources$source_Xie$label
#> [1] "Zie et al (2013) ). Sleep drives metabolite clearance from the adult brain."
#>
#> $structured$sources$source_Xie$xdoi
#> [1] "doi:10.1126/science.1241224"
#>
#> $structured$sources$source_Xie$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Xie$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Xie$fromDir
#> [1] "."
#>
#> $structured$sources$source_Xie$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Xie$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Brokaw
#> $structured$sources$source_Brokaw$id
#> [1] "source_Brokaw"
#>
#> $structured$sources$source_Brokaw$label
#> [1] "Brokaw et al (2016) Resting state EEG correlates of memory consolidation."
#>
#> $structured$sources$source_Brokaw$xdoi
#> [1] "doi:10.1016/j.nlm.2016.01.008"
#>
#> $structured$sources$source_Brokaw$pp
#> [1] "17-25"
#>
#> $structured$sources$source_Brokaw$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Brokaw$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Brokaw$fromDir
#> [1] "."
#>
#> $structured$sources$source_Brokaw$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Brokaw$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Marrosu
#> $structured$sources$source_Marrosu$id
#> [1] "source_Marrosu"
#>
#> $structured$sources$source_Marrosu$label
#> [1] "Marrosu et al (1995) Microdialysis measurement of cortical and hippocampal acetylcholine release during sleep-wake cycle in freely moving cats."
#>
#> $structured$sources$source_Marrosu$xdoi
#> [1] "No DOI found yet, located in: Brain Research, vol 671, pp 329–332."
#>
#> $structured$sources$source_Marrosu$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Marrosu$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Marrosu$fromDir
#> [1] "."
#>
#> $structured$sources$source_Marrosu$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Marrosu$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Lange
#> $structured$sources$source_Lange$id
#> [1] "source_Lange"
#>
#> $structured$sources$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $structured$sources$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $structured$sources$source_Lange$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Lange$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Lange$fromDir
#> [1] "."
#>
#> $structured$sources$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Burgess
#> $structured$sources$source_Burgess$id
#> [1] "source_Burgess"
#>
#> $structured$sources$source_Burgess$label
#> [1] "Burgess et al (2002) ). Bright light, dark and melatonin can promote circadian adaptation in night shift workers."
#>
#> $structured$sources$source_Burgess$xdoi
#> [1] "doi:10.1053/smrv.2001.0215"
#>
#> $structured$sources$source_Burgess$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Burgess$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Burgess$fromDir
#> [1] "."
#>
#> $structured$sources$source_Burgess$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Burgess$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Plog
#> $structured$sources$source_Plog$id
#> [1] "source_Plog"
#>
#> $structured$sources$source_Plog$label
#> [1] "Plog & Nedergaard (2018) The glymphatic system in central nervous system health and disease: past, present, and future"
#>
#> $structured$sources$source_Plog$xdoi
#> [1] "doi:10.1146/annurev-pathol-051217-111018"
#>
#> $structured$sources$source_Plog$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Plog$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Plog$fromDir
#> [1] "."
#>
#> $structured$sources$source_Plog$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Plog$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Killgore13
#> $structured$sources$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $structured$sources$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $structured$sources$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $structured$sources$source_Killgore13$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Killgore13$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Killgore13$fromDir
#> [1] "."
#>
#> $structured$sources$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Harrison
#> $structured$sources$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $structured$sources$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $structured$sources$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $structured$sources$source_Harrison$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Harrison$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Harrison$fromDir
#> [1] "."
#>
#> $structured$sources$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Doran
#> $structured$sources$source_Doran$id
#> [1] "source_Doran"
#>
#> $structured$sources$source_Doran$label
#> [1] "Doran et al (2001) Sustained attention performance during sleep deprivation: evidence of state instability."
#>
#> $structured$sources$source_Doran$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $structured$sources$source_Doran$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Doran$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Doran$fromDir
#> [1] "."
#>
#> $structured$sources$source_Doran$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Doran$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Killgore07
#> $structured$sources$source_Killgore07$id
#> [1] "source_Killgore07"
#>
#> $structured$sources$source_Killgore07$label
#> [1] "Killgore et al (2007) The trait of Introversion–Extraversion predicts vulnerability to sleep deprivation."
#>
#> $structured$sources$source_Killgore07$xdoi
#> [1] "doi:10.1111/j.1365-2869.2007.00611.x"
#>
#> $structured$sources$source_Killgore07$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Killgore07$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Killgore07$fromDir
#> [1] "."
#>
#> $structured$sources$source_Killgore07$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Killgore07$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_VanDongen
#> $structured$sources$source_VanDongen$id
#> [1] "source_VanDongen"
#>
#> $structured$sources$source_VanDongen$label
#> [1] "Van Dongen et al (2003) Sleep debt: Theoretical and empirical issues."
#>
#> $structured$sources$source_VanDongen$comment
#> [1] "No DOI found yet; located in Sleep and Biological Rhythms, vol 1, pp 5–13"
#>
#> $structured$sources$source_VanDongen$type
#> [1] "Journal article"
#>
#> $structured$sources$source_VanDongen$fromFile
#> [1] "none"
#>
#> $structured$sources$source_VanDongen$fromDir
#> [1] "."
#>
#> $structured$sources$source_VanDongen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_VanDongen$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Kuypers
#> $structured$sources$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $structured$sources$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $structured$sources$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $structured$sources$source_Kuypers$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Kuypers$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Kuypers$fromDir
#> [1] "."
#>
#> $structured$sources$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Williamson
#> $structured$sources$source_Williamson$id
#> [1] "source_Williamson"
#>
#> $structured$sources$source_Williamson$label
#> [1] "Williamson & Feyer (2000) Moderate sleep deprivation produces impairments in cognitive and motor performance equivalent to legally prescribed levels of alcohol intoxication."
#>
#> $structured$sources$source_Williamson$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Williamson$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Williamson$fromDir
#> [1] "."
#>
#> $structured$sources$source_Williamson$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Williamson$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Peeke
#> $structured$sources$source_Peeke$id
#> [1] "source_Peeke"
#>
#> $structured$sources$source_Peeke$label
#> [1] "Peeke et al (1980) Combined effects of alcohol and sleep deprivation in normal young adults."
#>
#> $structured$sources$source_Peeke$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Peeke$fromDir
#> [1] "."
#>
#> $structured$sources$source_Peeke$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Peeke$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Croes
#> $structured$sources$source_Croes$id
#> [1] "source_Croes"
#>
#> $structured$sources$source_Croes$label
#> [1] "Croes et al (2017) Langdurige klachten na ecstasygebruik."
#>
#> $structured$sources$source_Croes$xdoi
#> [1] "url:www.trimbos.nl/docs/2c1748e6-93d5-481b-8fad-013f91a9e1df.pdf"
#>
#> $structured$sources$source_Croes$type
#> [1] "Report"
#>
#> $structured$sources$source_Croes$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Croes$fromDir
#> [1] "."
#>
#> $structured$sources$source_Croes$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Croes$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Berger
#> $structured$sources$source_Berger$id
#> [1] "source_Berger"
#>
#> $structured$sources$source_Berger$label
#> [1] "Energy conservation and sleep."
#>
#> $structured$sources$source_Berger$xdoi
#> [1] "doi:10.1016/0166-4328(95)00002-b"
#>
#> $structured$sources$source_Berger$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Berger$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Berger$fromDir
#> [1] "."
#>
#> $structured$sources$source_Berger$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Berger$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Fairclough
#> $structured$sources$source_Fairclough$id
#> [1] "source_Fairclough"
#>
#> $structured$sources$source_Fairclough$label
#> [1] "Fairclough & Graham (1999) Impairment of driving performance caused by sleep deprivation or alcohol: A comparative Study. "
#>
#> $structured$sources$source_Fairclough$xdoi
#> [1] "doi:10.1518/001872099779577336"
#>
#> $structured$sources$source_Fairclough$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Fairclough$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Fairclough$fromDir
#> [1] "."
#>
#> $structured$sources$source_Fairclough$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Fairclough$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Krueger
#> $structured$sources$source_Krueger$id
#> [1] "source_Krueger"
#>
#> $structured$sources$source_Krueger$label
#> [1] "Kreueger et al (2006) The role of cytokines in physiological sleep regulation"
#>
#> $structured$sources$source_Krueger$xdoi
#> [1] "doi:10.1111/j.1749-6632.2001.tb05826.x"
#>
#> $structured$sources$source_Krueger$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Krueger$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Krueger$fromDir
#> [1] "."
#>
#> $structured$sources$source_Krueger$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Krueger$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Jessen
#> $structured$sources$source_Jessen$id
#> [1] "source_Jessen"
#>
#> $structured$sources$source_Jessen$label
#> [1] "Jessen et al (2015) The Glymphatic System: A Beginner’s Guide."
#>
#> $structured$sources$source_Jessen$xdoi
#> [1] "doi:10.1007/s11064-015-1581-6"
#>
#> $structured$sources$source_Jessen$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Jessen$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Jessen$fromDir
#> [1] "."
#>
#> $structured$sources$source_Jessen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Jessen$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Lim
#> $structured$sources$source_Lim$id
#> [1] "source_Lim"
#>
#> $structured$sources$source_Lim$label
#> [1] "Lim & Dinges (2010) A meta-analysis of the impact of short-term sleep deprivation on cognitive variables."
#>
#> $structured$sources$source_Lim$xdoi
#> [1] "doi:10.1037/a0018883"
#>
#> $structured$sources$source_Lim$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Lim$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Lim$fromDir
#> [1] "."
#>
#> $structured$sources$source_Lim$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Lim$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Tononi
#> $structured$sources$source_Tononi$id
#> [1] "source_Tononi"
#>
#> $structured$sources$source_Tononi$label
#> [1] "Tononi & Cirelli (2006) ). Sleep function and synaptic homeostasis."
#>
#> $structured$sources$source_Tononi$xdoi
#> [1] "doi:10.1016/j.smrv.2005.05.002"
#>
#> $structured$sources$source_Tononi$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Tononi$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Tononi$fromDir
#> [1] "."
#>
#> $structured$sources$source_Tononi$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Tononi$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Beebe
#> $structured$sources$source_Beebe$id
#> [1] "source_Beebe"
#>
#> $structured$sources$source_Beebe$label
#> [1] "Beebe et al (2010). Attention, learning, and arousal of experimentally sleep-restricted adolescents in a simulated classroom"
#>
#> $structured$sources$source_Beebe$xdoi
#> [1] "doi:10.1016/j.jadohealth.2010.03.005"
#>
#> $structured$sources$source_Beebe$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Beebe$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Beebe$fromDir
#> [1] "."
#>
#> $structured$sources$source_Beebe$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Beebe$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_Harrison
#> $structured$sources$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $structured$sources$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $structured$sources$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $structured$sources$source_Harrison$type
#> [1] "Journal article"
#>
#> $structured$sources$source_Harrison$fromFile
#> [1] "none"
#>
#> $structured$sources$source_Harrison$fromDir
#> [1] "."
#>
#> $structured$sources$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$phd_peters_xtc_determinants
#> $structured$sources$phd_peters_xtc_determinants$id
#> [1] "phd_peters_xtc_determinants"
#>
#> $structured$sources$phd_peters_xtc_determinants$label
#> [1] "Peters, G. J. Y. (2008) Determinants of ecstasy use and harm reduction strategies: informing evidence-based intervention development. https://phdthesis.nl"
#>
#> $structured$sources$phd_peters_xtc_determinants$xdoi
#> [1] "isbn:9781409240716"
#>
#> $structured$sources$phd_peters_xtc_determinants$fromFile
#> [1] "none"
#>
#> $structured$sources$phd_peters_xtc_determinants$fromDir
#> [1] "."
#>
#> $structured$sources$phd_peters_xtc_determinants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$phd_peters_xtc_determinants$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$phd_vervaeke_xtc_social
#> $structured$sources$phd_vervaeke_xtc_social$id
#> [1] "phd_vervaeke_xtc_social"
#>
#> $structured$sources$phd_vervaeke_xtc_social$label
#> [1] "Vervaeke, H. K. E. (2009) Initiation and continuation: social context and behavioural aspects of ecstasy use"
#>
#> $structured$sources$phd_vervaeke_xtc_social$fromFile
#> [1] "none"
#>
#> $structured$sources$phd_vervaeke_xtc_social$fromDir
#> [1] "."
#>
#> $structured$sources$phd_vervaeke_xtc_social$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$phd_vervaeke_xtc_social$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$`src:phd_peters_determinant_xtc_use`
#> $structured$sources$`src:phd_peters_determinant_xtc_use`$id
#> [1] "src:phd_peters_determinant_xtc_use"
#>
#> $structured$sources$`src:phd_peters_determinant_xtc_use`$pp
#> [1] 91
#>
#> $structured$sources$`src:phd_peters_determinant_xtc_use`$fromFile
#> [1] "none"
#>
#> $structured$sources$`src:phd_peters_determinant_xtc_use`$fromDir
#> [1] "."
#>
#> $structured$sources$`src:phd_peters_determinant_xtc_use`$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$`src:phd_peters_determinant_xtc_use`$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$`src:phd_vervaeke_xtc_social`
#> $structured$sources$`src:phd_vervaeke_xtc_social`$id
#> [1] "src:phd_vervaeke_xtc_social"
#>
#> $structured$sources$`src:phd_vervaeke_xtc_social`$pp
#> [1] 36
#>
#> $structured$sources$`src:phd_vervaeke_xtc_social`$fromFile
#> [1] "none"
#>
#> $structured$sources$`src:phd_vervaeke_xtc_social`$fromDir
#> [1] "."
#>
#> $structured$sources$`src:phd_vervaeke_xtc_social`$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$`src:phd_vervaeke_xtc_social`$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_1
#> $structured$sources$source_1$id
#> [1] "source_1"
#>
#> $structured$sources$source_1$label
#> [1] "Source 1"
#>
#> $structured$sources$source_1$xdoi
#> [1] "doi:10.0001/1000-0001.1.0.1"
#>
#> $structured$sources$source_1$type
#> [1] "Journal article"
#>
#> $structured$sources$source_1$fromFile
#> [1] "none"
#>
#> $structured$sources$source_1$fromDir
#> [1] "."
#>
#> $structured$sources$source_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_2
#> $structured$sources$source_2$id
#> [1] "source_2"
#>
#> $structured$sources$source_2$label
#> [1] "Source 2"
#>
#> $structured$sources$source_2$xdoi
#> [1] "doi:10.0002/2000-0002.2.0.2"
#>
#> $structured$sources$source_2$type
#> [1] "Journal article"
#>
#> $structured$sources$source_2$fromFile
#> [1] "none"
#>
#> $structured$sources$source_2$fromDir
#> [1] "."
#>
#> $structured$sources$source_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$source_3
#> $structured$sources$source_3$id
#> [1] "source_3"
#>
#> $structured$sources$source_3$label
#> [1] "Source 3"
#>
#> $structured$sources$source_3$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $structured$sources$source_3$type
#> [1] "Journal article"
#>
#> $structured$sources$source_3$fromFile
#> [1] "none"
#>
#> $structured$sources$source_3$fromDir
#> [1] "."
#>
#> $structured$sources$source_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$source_3$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$brunt_2012
#> $structured$sources$brunt_2012$id
#> [1] "brunt_2012"
#>
#> $structured$sources$brunt_2012$label
#> [1] "Brunt, Koeter, Niesink & van den Brink (2012)"
#>
#> $structured$sources$brunt_2012$evidence_type
#> [1] "empirical study"
#>
#> $structured$sources$brunt_2012$fromFile
#> [1] "none"
#>
#> $structured$sources$brunt_2012$fromDir
#> [1] "."
#>
#> $structured$sources$brunt_2012$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$brunt_2012$date
#> [1] "2019-03-06"
#>
#> $structured$sources$brunt_2012$scores
#> $structured$sources$brunt_2012$scores$evidence_type
#> [1] 5
#>
#>
#>
#> $structured$sources$project_proposal
#> $structured$sources$project_proposal$id
#> [1] "project_proposal"
#>
#> $structured$sources$project_proposal$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $structured$sources$project_proposal$year
#> [1] 2017
#>
#> $structured$sources$project_proposal$fromFile
#> [1] "none"
#>
#> $structured$sources$project_proposal$fromDir
#> [1] "."
#>
#> $structured$sources$project_proposal$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$project_proposal$date
#> [1] "2019-03-06"
#>
#>
#> $structured$sources$nice_round_numbers_are_nice
#> $structured$sources$nice_round_numbers_are_nice$id
#> [1] "nice_round_numbers_are_nice"
#>
#> $structured$sources$nice_round_numbers_are_nice$year
#> [1] 219
#>
#> $structured$sources$nice_round_numbers_are_nice$label
#> [1] "In our meeting of 2019-07-05, we agreed that all team members like nice round numbers."
#>
#> $structured$sources$nice_round_numbers_are_nice$evidence_type
#> [1] "team opinion"
#>
#> $structured$sources$nice_round_numbers_are_nice$fromFile
#> [1] "none"
#>
#> $structured$sources$nice_round_numbers_are_nice$fromDir
#> [1] "."
#>
#> $structured$sources$nice_round_numbers_are_nice$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$nice_round_numbers_are_nice$date
#> [1] "2019-03-06"
#>
#> $structured$sources$nice_round_numbers_are_nice$scores
#> $structured$sources$nice_round_numbers_are_nice$scores$evidence_type
#> [1] 1
#>
#>
#>
#> $structured$sources$moinester_gottfried_2014
#> $structured$sources$moinester_gottfried_2014$id
#> [1] "moinester_gottfried_2014"
#>
#> $structured$sources$moinester_gottfried_2014$year
#> [1] 2014
#>
#> $structured$sources$moinester_gottfried_2014$label
#> [1] "Moinester, M., & Gottfried, R. (2014). Sample size estimation for correlations with pre-specified confidence interval. The Quantitative Methods of Psychology, 10(2), 124–130."
#>
#> $structured$sources$moinester_gottfried_2014$evidence_type
#> [1] "theory"
#>
#> $structured$sources$moinester_gottfried_2014$fromFile
#> [1] "none"
#>
#> $structured$sources$moinester_gottfried_2014$fromDir
#> [1] "."
#>
#> $structured$sources$moinester_gottfried_2014$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$moinester_gottfried_2014$date
#> [1] "2019-03-06"
#>
#> $structured$sources$moinester_gottfried_2014$scores
#> $structured$sources$moinester_gottfried_2014$scores$evidence_type
#> [1] 4
#>
#>
#>
#> $structured$sources$example_source
#> $structured$sources$example_source$id
#> [1] "example_source"
#>
#> $structured$sources$example_source$label
#> [1] "Not online now, but a study making this point would be nice here"
#>
#> $structured$sources$example_source$evidence_type
#> [1] "empirical study"
#>
#> $structured$sources$example_source$fromFile
#> [1] "none"
#>
#> $structured$sources$example_source$fromDir
#> [1] "."
#>
#> $structured$sources$example_source$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$sources$example_source$date
#> [1] "2019-03-06"
#>
#> $structured$sources$example_source$scores
#> $structured$sources$example_source$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $structured$assertions
#> $structured$assertions$assertion_sleep_memory_1
#> $structured$assertions$assertion_sleep_memory_1$id
#> [1] "assertion_sleep_memory_1"
#>
#> $structured$assertions$assertion_sleep_memory_1$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $structured$assertions$assertion_sleep_memory_1$source
#> [1] "source_Diekelmann"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_memory_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_memory_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_memory_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_memory_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_sleep_memory_2
#> $structured$assertions$assertion_sleep_memory_2$id
#> [1] "assertion_sleep_memory_2"
#>
#> $structured$assertions$assertion_sleep_memory_2$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $structured$assertions$assertion_sleep_memory_2$source
#> [1] "source_Gais"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_memory_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_memory_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_memory_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_memory_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_sleep_memory_3
#> $structured$assertions$assertion_sleep_memory_3$id
#> [1] "assertion_sleep_memory_3"
#>
#> $structured$assertions$assertion_sleep_memory_3$label
#> [1] "Sleep promotes the consolidation of fear memory in humans."
#>
#> $structured$assertions$assertion_sleep_memory_3$source
#> [1] "source_Menz"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_memory_3$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_memory_3$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_memory_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_memory_3$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_sleep_memory_4
#> $structured$assertions$assertion_sleep_memory_4$id
#> [1] "assertion_sleep_memory_4"
#>
#> $structured$assertions$assertion_sleep_memory_4$label
#> [1] "Sleep promotes the consolidation of emotional memory in humans."
#>
#> $structured$assertions$assertion_sleep_memory_4$source
#> [1] "source_Holland"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_memory_4$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_memory_4$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_memory_4$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_memory_4$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_sleep_memory_5
#> $structured$assertions$assertion_sleep_memory_5$id
#> [1] "assertion_sleep_memory_5"
#>
#> $structured$assertions$assertion_sleep_memory_5$label
#> [1] "Sleep promotes the consolidation of memory in animals after training for a specific task."
#>
#> $structured$assertions$assertion_sleep_memory_5$source
#> [1] "source_Graves"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_memory_5$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_memory_5$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_memory_5$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_memory_5$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_sleep_memory_6
#> $structured$assertions$assertion_sleep_memory_6$id
#> [1] "assertion_sleep_memory_6"
#>
#> $structured$assertions$assertion_sleep_memory_6$label
#> [1] "Memory retention is already noticable after only several minutes of sleep."
#>
#> $structured$assertions$assertion_sleep_memory_6$source
#> [1] "source_Diekelmann"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_memory_6$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_memory_6$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_memory_6$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_memory_6$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_cytokines
#> $structured$assertions$assertion_cytokines$id
#> [1] "assertion_cytokines"
#>
#> $structured$assertions$assertion_cytokines$label
#> [1] "Infection, stress and tissue damage triggers the release of inflammatory cytokines."
#>
#> $structured$assertions$assertion_cytokines$source
#> [1] "source_Frey"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_cytokines$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_cytokines$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_cytokines$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_cytokines_function
#> $structured$assertions$assertion_cytokines_function$id
#> [1] "assertion_cytokines_function"
#>
#> $structured$assertions$assertion_cytokines_function$label
#> [1] "When pro-inflammatory cytokines are injected, these cytokines enhance sleep."
#>
#> $structured$assertions$assertion_cytokines_function$source
#> [1] "source_Krueger"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_cytokines_function$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_cytokines_function$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_cytokines_function$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_cytokines_function$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_sleep_glympathic_system
#> $structured$assertions$assertion_sleep_glympathic_system$id
#> [1] "assertion_sleep_glympathic_system"
#>
#> $structured$assertions$assertion_sleep_glympathic_system$label
#> [1] "Sleep promotes the glympathic system."
#>
#> $structured$assertions$assertion_sleep_glympathic_system$source
#> [1] "source_Xie"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_glympathic_system$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_glympathic_system$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_glympathic_system$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_glympathic_system$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_cytokines
#> $structured$assertions$assertion_SD_cytokines$id
#> [1] "assertion_SD_cytokines"
#>
#> $structured$assertions$assertion_SD_cytokines$label
#> [1] "Sleep deprivation is associated with an increase of pro-inflammatory cytokines, which creates a disbalance of inflammatory cytokines and this induces inflammation."
#>
#> $structured$assertions$assertion_SD_cytokines$source
#> [1] "source_Frey"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_cytokines$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_cytokines$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_cytokines$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_resting_1
#> $structured$assertions$assertion_resting_1$id
#> [1] "assertion_resting_1"
#>
#> $structured$assertions$assertion_resting_1$label
#> [1] "Resting enhances the consolidation of memory."
#>
#> $structured$assertions$assertion_resting_1$source
#> [1] "source_Brokaw"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_resting_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_resting_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_resting_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_resting_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_resting_2
#> $structured$assertions$assertion_resting_2$id
#> [1] "assertion_resting_2"
#>
#> $structured$assertions$assertion_resting_2$label
#> [1] "The neurotransmitter ACh is released during quiet rest, just like during sleep."
#>
#> $structured$assertions$assertion_resting_2$source
#> [1] "source_Marrosu"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_resting_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_resting_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_resting_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_resting_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_nocturnal_1
#> $structured$assertions$assertion_nocturnal_1$id
#> [1] "assertion_nocturnal_1"
#>
#> $structured$assertions$assertion_nocturnal_1$label
#> [1] "Cells like leukocytes show a specific diurnal or nocturnal rhythm."
#>
#> $structured$assertions$assertion_nocturnal_1$source
#> [1] "source_Lange"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_nocturnal_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_nocturnal_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_nocturnal_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_nocturnal_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_nocturnal_2
#> $structured$assertions$assertion_nocturnal_2$id
#> [1] "assertion_nocturnal_2"
#>
#> $structured$assertions$assertion_nocturnal_2$label
#> [1] "During nocturnal sleep cell counts of toxic cells are suppressed."
#>
#> $structured$assertions$assertion_nocturnal_2$source
#> [1] "source_Lange"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_nocturnal_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_nocturnal_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_nocturnal_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_nocturnal_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_sleep_debt
#> $structured$assertions$assertion_sleep_debt$id
#> [1] "assertion_sleep_debt"
#>
#> $structured$assertions$assertion_sleep_debt$label
#> [1] "Sleep deprivation results in a sleep debt, which has unhealthy consequences."
#>
#> $structured$assertions$assertion_sleep_debt$source
#> [1] "source_Burgess"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_sleep_debt$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_sleep_debt$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_sleep_debt$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_sleep_debt$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_glympathic_system_2
#> $structured$assertions$assertion_glympathic_system_2$id
#> [1] "assertion_glympathic_system_2"
#>
#> $structured$assertions$assertion_glympathic_system_2$label
#> [1] "Failure of the glympathic system is associated with Alzheimer's disease."
#>
#> $structured$assertions$assertion_glympathic_system_2$source
#> [1] "source_Plog"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_glympathic_system_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_glympathic_system_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_glympathic_system_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_glympathic_system_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_glympathic_system_1
#> $structured$assertions$assertion_glympathic_system_1$id
#> [1] "assertion_glympathic_system_1"
#>
#> $structured$assertions$assertion_glympathic_system_1$label
#> [1] "The glympathic system is a recently discovered waste clearance pathway that removes metabolites and toxic proteins from the brain."
#>
#> $structured$assertions$assertion_glympathic_system_1$source
#> [1] "source_Jessen"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_glympathic_system_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_glympathic_system_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_glympathic_system_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_glympathic_system_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_performance
#> $structured$assertions$assertion_SD_performance$id
#> [1] "assertion_SD_performance"
#>
#> $structured$assertions$assertion_SD_performance$label
#> [1] "(Cognitive) performance deteriorates after 16 hours of wakefulness."
#>
#> $structured$assertions$assertion_SD_performance$source
#> [1] "source_Killgore13"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_performance$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_performance$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_performance$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_performance$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_sensory_perception
#> $structured$assertions$assertion_SD_sensory_perception$id
#> [1] "assertion_SD_sensory_perception"
#>
#> $structured$assertions$assertion_SD_sensory_perception$label
#> [1] "Sleep loss causes a reduction in visual cortex activity and the reduction is most prominent when an individual experiences an attentional lapse."
#>
#> $structured$assertions$assertion_SD_sensory_perception$source
#> [1] "source_Killgore13"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_sensory_perception$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_sensory_perception$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_sensory_perception$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_sensory_perception$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_inattentive_behaviours
#> $structured$assertions$assertion_inattentive_behaviours$id
#> [1] "assertion_inattentive_behaviours"
#>
#> $structured$assertions$assertion_inattentive_behaviours$label
#> [1] "sleep deprived individuals showed significantly more inattentive behaviours compared to non-sleep deprived individuals while watching a movie."
#>
#> $structured$assertions$assertion_inattentive_behaviours$source
#> [1] "source_Beebe"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_inattentive_behaviours$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_inattentive_behaviours$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_inattentive_behaviours$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_inattentive_behaviours$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_decision
#> $structured$assertions$assertion_SD_decision$id
#> [1] "assertion_SD_decision"
#>
#> $structured$assertions$assertion_SD_decision$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $structured$assertions$assertion_SD_decision$source
#> [1] "source_Harrison"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_decision$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_decision$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_decision$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_decision$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_state_instability
#> $structured$assertions$assertion_state_instability$id
#> [1] "assertion_state_instability"
#>
#> $structured$assertions$assertion_state_instability$label
#> [1] "After sleep deprivation performance becomes instable, which is called state instability."
#>
#> $structured$assertions$assertion_state_instability$source
#> [1] "source_Doran"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_state_instability$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_state_instability$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_state_instability$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_state_instability$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_emotion_1
#> $structured$assertions$assertion_SD_emotion_1$id
#> [1] "assertion_SD_emotion_1"
#>
#> $structured$assertions$assertion_SD_emotion_1$label
#> [1] "Sleep loss enhances the strenth of reactions to negative, but not to positive or neutral stimuli."
#>
#> $structured$assertions$assertion_SD_emotion_1$source
#> [1] "source_Killgore13"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_emotion_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_emotion_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_emotion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_emotion_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_emotion_2
#> $structured$assertions$assertion_SD_emotion_2$id
#> [1] "assertion_SD_emotion_2"
#>
#> $structured$assertions$assertion_SD_emotion_2$label
#> [1] "Humor is negatively evaluated and emotional expression is lost in the voice after sleep deprivation."
#>
#> $structured$assertions$assertion_SD_emotion_2$source
#> [1] "source_Killgore13"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_emotion_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_emotion_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_emotion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_emotion_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_individual_differences_1
#> $structured$assertions$assertion_individual_differences_1$id
#> [1] "assertion_individual_differences_1"
#>
#> $structured$assertions$assertion_individual_differences_1$label
#> [1] "The personality trait extraversion is more related to attentional lapses and more extensive declines in speed response during a task after one night of sleep deprivation."
#>
#> $structured$assertions$assertion_individual_differences_1$source
#> [1] "source_Killgore07"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_individual_differences_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_individual_differences_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_individual_differences_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_individual_differences_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_individual_differences_2
#> $structured$assertions$assertion_individual_differences_2$id
#> [1] "assertion_individual_differences_2"
#>
#> $structured$assertions$assertion_individual_differences_2$label
#> [1] "There is a genetic predisposition for vulnerability to sleep loss."
#>
#> $structured$assertions$assertion_individual_differences_2$source
#> [1] "source_VanDongen"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_individual_differences_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_individual_differences_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_individual_differences_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_individual_differences_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_MDMA_impulse_daytime
#> $structured$assertions$assertion_MDMA_impulse_daytime$id
#> [1] "assertion_MDMA_impulse_daytime"
#>
#> $structured$assertions$assertion_MDMA_impulse_daytime$label
#> [1] "When MDMA is taken during daytime, impulse control is enhanced."
#>
#> $structured$assertions$assertion_MDMA_impulse_daytime$source
#> [1] "source_Kuypers"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_MDMA_impulse_daytime$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_MDMA_impulse_daytime$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_MDMA_impulse_daytime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_MDMA_impulse_daytime$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_MDMA_impulse_nighttime
#> $structured$assertions$assertion_MDMA_impulse_nighttime$id
#> [1] "assertion_MDMA_impulse_nighttime"
#>
#> $structured$assertions$assertion_MDMA_impulse_nighttime$label
#> [1] "When MDMA is taken during nighttime, impulse control is not enhanced."
#>
#> $structured$assertions$assertion_MDMA_impulse_nighttime$source
#> [1] "source_Kuypers"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_MDMA_impulse_nighttime$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_MDMA_impulse_nighttime$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_MDMA_impulse_nighttime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_MDMA_impulse_nighttime$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_MDMA_PMF_daytime
#> $structured$assertions$assertion_MDMA_PMF_daytime$id
#> [1] "assertion_MDMA_PMF_daytime"
#>
#> $structured$assertions$assertion_MDMA_PMF_daytime$label
#> [1] "When MDMA is taken during daytime psychomotor functioning is enhanced."
#>
#> $structured$assertions$assertion_MDMA_PMF_daytime$source
#> [1] "source_Kuypers"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_MDMA_PMF_daytime$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_MDMA_PMF_daytime$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_MDMA_PMF_daytime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_MDMA_PMF_daytime$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_MDMA_PMF_nighttime
#> $structured$assertions$assertion_MDMA_PMF_nighttime$id
#> [1] "assertion_MDMA_PMF_nighttime"
#>
#> $structured$assertions$assertion_MDMA_PMF_nighttime$label
#> [1] "When MDMA is taken during nighttime psychomotor functioning is impaired."
#>
#> $structured$assertions$assertion_MDMA_PMF_nighttime$source
#> [1] "source_Kuypers"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_MDMA_PMF_nighttime$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_MDMA_PMF_nighttime$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_MDMA_PMF_nighttime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_MDMA_PMF_nighttime$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_alcohol
#> $structured$assertions$assertion_SD_alcohol$id
#> [1] "assertion_SD_alcohol"
#>
#> $structured$assertions$assertion_SD_alcohol$label
#> [1] "Sleep deprivation causes cognitive and motor performance impairment similar to alcohol intoxication."
#>
#> $structured$assertions$assertion_SD_alcohol$source
#> [1] "source_Williamson"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_alcohol$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_alcohol$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_alcohol$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_alcohol$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_1
#> $structured$assertions$assertion_interaction_SD_alcohol_1$id
#> [1] "assertion_interaction_SD_alcohol_1"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_1$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived react slower than people who are either are under the influence of alcohol or sleep deprived alone."
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_1$source
#> [1] "source_Peeke"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2
#> $structured$assertions$assertion_interaction_SD_alcohol_2$id
#> [1] "assertion_interaction_SD_alcohol_2"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived show worse driving performance than people who either are under the influence of alcohol or sleep deprived alone."
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2$comment
#> [1] "this source was 'Howard'. But the one that was named 'Howard', I renamed to 'Harrison'. Smth wrong here?"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2$source
#> [1] "source_Harrison"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_interaction_SD_alcohol_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_MDMA_cortisol
#> $structured$assertions$assertion_MDMA_cortisol$id
#> [1] "assertion_MDMA_cortisol"
#>
#> $structured$assertions$assertion_MDMA_cortisol$label
#> [1] "Cortisol levels rise after MDMA is taken."
#>
#> $structured$assertions$assertion_MDMA_cortisol$source
#> [1] "source_Croes"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_MDMA_cortisol$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_MDMA_cortisol$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_MDMA_cortisol$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_MDMA_cortisol$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_resting_3
#> $structured$assertions$assertion_resting_3$id
#> [1] "assertion_resting_3"
#>
#> $structured$assertions$assertion_resting_3$label
#> [1] "Important functions of sleep, like recovery of neural function and synaptic downscaling to save energy and experience benefits for learning and memory, only occur together with slow wave sleep and therefore not while resting."
#>
#> $structured$assertions$assertion_resting_3$source
#> [1] "source_Tononi"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_resting_3$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_resting_3$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_resting_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_resting_3$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_attention
#> $structured$assertions$assertion_attention$id
#> [1] "assertion_attention"
#>
#> $structured$assertions$assertion_attention$label
#> [1] "The most simple form of attention, that is being able to detect a stimulus, is most strongly affected by sleep deprivation."
#>
#> $structured$assertions$assertion_attention$source
#> [1] "source_Lim"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_attention$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_attention$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_attention$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_attention$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_SD_decision
#> $structured$assertions$assertion_SD_decision$id
#> [1] "assertion_SD_decision"
#>
#> $structured$assertions$assertion_SD_decision$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $structured$assertions$assertion_SD_decision$source
#> [1] "source_Harrison"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_SD_decision$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_SD_decision$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_SD_decision$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_SD_decision$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$tryout_determinants_ranking_intention
#> $structured$assertions$tryout_determinants_ranking_intention$id
#> [1] "tryout_determinants_ranking_intention"
#>
#> $structured$assertions$tryout_determinants_ranking_intention$label
#> [1] "Intention to try out XTC is an extremely strong predictor of trying out XTC (Cohen's d > 1, OR > 7)"
#>
#> $structured$assertions$tryout_determinants_ranking_intention$source
#> [1] "src:phd_peters_determinant_xtc_use"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$tryout_determinants_ranking_intention$fromFile
#> [1] "none"
#>
#> $structured$assertions$tryout_determinants_ranking_intention$fromDir
#> [1] "."
#>
#> $structured$assertions$tryout_determinants_ranking_intention$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$tryout_determinants_ranking_intention$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$tryout_determinants_ranking_attitude
#> $structured$assertions$tryout_determinants_ranking_attitude$id
#> [1] "tryout_determinants_ranking_attitude"
#>
#> $structured$assertions$tryout_determinants_ranking_attitude$label
#> [1] "Attitude is the strongest predictor of intention to try out XTC (r = .67)"
#>
#> $structured$assertions$tryout_determinants_ranking_attitude$source
#> [1] "src:phd_peters_determinant_xtc_use"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$tryout_determinants_ranking_attitude$fromFile
#> [1] "none"
#>
#> $structured$assertions$tryout_determinants_ranking_attitude$fromDir
#> [1] "."
#>
#> $structured$assertions$tryout_determinants_ranking_attitude$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$tryout_determinants_ranking_attitude$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$notry_determinants_ranking_othersubstance
#> $structured$assertions$notry_determinants_ranking_othersubstance$id
#> [1] "notry_determinants_ranking_othersubstance"
#>
#> $structured$assertions$notry_determinants_ranking_othersubstance$label
#> [1] "Already using some other substance is the strongest 'deterrent' of trying out ecstasy"
#>
#> $structured$assertions$notry_determinants_ranking_othersubstance$source
#> [1] "src:phd_vervaeke_xtc_social"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$notry_determinants_ranking_othersubstance$fromFile
#> [1] "none"
#>
#> $structured$assertions$notry_determinants_ranking_othersubstance$fromDir
#> [1] "."
#>
#> $structured$assertions$notry_determinants_ranking_othersubstance$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$notry_determinants_ranking_othersubstance$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_1
#> $structured$assertions$assertion_1$id
#> [1] "assertion_1"
#>
#> $structured$assertions$assertion_1$label
#> [1] "Assertion 1"
#>
#> $structured$assertions$assertion_1$description
#> [1] "Description of assertion 1"
#>
#> $structured$assertions$assertion_1$source
#> [1] "source_1" "source_2"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_1$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_1$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$assertion_2
#> $structured$assertions$assertion_2$id
#> [1] "assertion_2"
#>
#> $structured$assertions$assertion_2$label
#> [1] "Assertion 2"
#>
#> $structured$assertions$assertion_2$description
#> [1] "Description of assertion 2"
#>
#> $structured$assertions$assertion_2$source
#> [1] "source_3"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$assertion_2$fromFile
#> [1] "none"
#>
#> $structured$assertions$assertion_2$fromDir
#> [1] "."
#>
#> $structured$assertions$assertion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$assertion_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$some_pills_are_contaminated
#> $structured$assertions$some_pills_are_contaminated$id
#> [1] "some_pills_are_contaminated"
#>
#> $structured$assertions$some_pills_are_contaminated$source
#> [1] "vogels_2009"
#>
#> $structured$assertions$some_pills_are_contaminated$label
#> [1] "Not all produced XTC pills contain only MDMA as active ingredient."
#>
#> $structured$assertions$some_pills_are_contaminated$evidence_type
#> [1] "empirical study"
#>
#> $structured$assertions$some_pills_are_contaminated$fromFile
#> [1] "none"
#>
#> $structured$assertions$some_pills_are_contaminated$fromDir
#> [1] "."
#>
#> $structured$assertions$some_pills_are_contaminated$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$some_pills_are_contaminated$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$dosing_requires_pill_content
#> $structured$assertions$dosing_requires_pill_content$id
#> [1] "dosing_requires_pill_content"
#>
#> $structured$assertions$dosing_requires_pill_content$label
#> [1] "Determining the dose of MDMA one uses requires knowing both one's body weight and the pill dose."
#>
#> $structured$assertions$dosing_requires_pill_content$source
#> [1] "brunt_2012"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$dosing_requires_pill_content$fromFile
#> [1] "none"
#>
#> $structured$assertions$dosing_requires_pill_content$fromDir
#> [1] "."
#>
#> $structured$assertions$dosing_requires_pill_content$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$dosing_requires_pill_content$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$testing_xtc_is_reasoned
#> $structured$assertions$testing_xtc_is_reasoned$id
#> [1] "testing_xtc_is_reasoned"
#>
#> $structured$assertions$testing_xtc_is_reasoned$source
#> [1] "phd_peters_2008"
#>
#> $structured$assertions$testing_xtc_is_reasoned$label
#> [1] "Previous research indicates that getting one's ecstasy tested (or not) is largely a reasoned behavior"
#>
#> $structured$assertions$testing_xtc_is_reasoned$fromFile
#> [1] "none"
#>
#> $structured$assertions$testing_xtc_is_reasoned$fromDir
#> [1] "."
#>
#> $structured$assertions$testing_xtc_is_reasoned$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$testing_xtc_is_reasoned$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$there_is_qualitative_research_about_testing
#> $structured$assertions$there_is_qualitative_research_about_testing$id
#> [1] "there_is_qualitative_research_about_testing"
#>
#> $structured$assertions$there_is_qualitative_research_about_testing$source
#> [1] "phd_peters_2008"
#>
#> $structured$assertions$there_is_qualitative_research_about_testing$label
#> [1] "There exists qualitative research about why people get their ecstasy tested"
#>
#> $structured$assertions$there_is_qualitative_research_about_testing$fromFile
#> [1] "none"
#>
#> $structured$assertions$there_is_qualitative_research_about_testing$fromDir
#> [1] "."
#>
#> $structured$assertions$there_is_qualitative_research_about_testing$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$there_is_qualitative_research_about_testing$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$project_deadline
#> $structured$assertions$project_deadline$id
#> [1] "project_deadline"
#>
#> $structured$assertions$project_deadline$label
#> [1] "The deadline for this project is december 2019."
#>
#> $structured$assertions$project_deadline$source
#> [1] "project_proposal"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$project_deadline$fromFile
#> [1] "none"
#>
#> $structured$assertions$project_deadline$fromDir
#> [1] "."
#>
#> $structured$assertions$project_deadline$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$project_deadline$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$nice_round_number
#> $structured$assertions$nice_round_number$id
#> [1] "nice_round_number"
#>
#> $structured$assertions$nice_round_number$label
#> [1] "We want to recruit a nice round number of participants."
#>
#> $structured$assertions$nice_round_number$source
#> [1] "nice_round_numbers_are_nice"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$nice_round_number$fromFile
#> [1] "none"
#>
#> $structured$assertions$nice_round_number$fromDir
#> [1] "."
#>
#> $structured$assertions$nice_round_number$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$nice_round_number$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$exact_aipe_for_our_study
#> $structured$assertions$exact_aipe_for_our_study$id
#> [1] "exact_aipe_for_our_study"
#>
#> $structured$assertions$exact_aipe_for_our_study$label
#> [1] "Table 1 shows that 383 participants are sufficient even for correlations as low as .05."
#>
#> $structured$assertions$exact_aipe_for_our_study$source
#> [1] "moinester_gottfried_2014"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$exact_aipe_for_our_study$fromFile
#> [1] "none"
#>
#> $structured$assertions$exact_aipe_for_our_study$fromDir
#> [1] "."
#>
#> $structured$assertions$exact_aipe_for_our_study$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$exact_aipe_for_our_study$date
#> [1] "2019-03-06"
#>
#>
#> $structured$assertions$prereg_to_decrease_bias
#> $structured$assertions$prereg_to_decrease_bias$id
#> [1] "prereg_to_decrease_bias"
#>
#> $structured$assertions$prereg_to_decrease_bias$label
#> [1] "Preregistration_decreases_bias"
#>
#> $structured$assertions$prereg_to_decrease_bias$source
#> [1] "example_source"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$assertions$prereg_to_decrease_bias$fromFile
#> [1] "none"
#>
#> $structured$assertions$prereg_to_decrease_bias$fromDir
#> [1] "."
#>
#> $structured$assertions$prereg_to_decrease_bias$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$assertions$prereg_to_decrease_bias$date
#> [1] "2019-03-06"
#>
#>
#>
#> $structured$justifications
#> $structured$justifications$decision1
#> $structured$justifications$decision1$id
#> [1] "decision1"
#>
#> $structured$justifications$decision1$`project-id`
#> [1] "wim"
#>
#> $structured$justifications$decision1$name
#> [1] "last finalization study recruitment"
#>
#> $structured$justifications$decision1$actors
#> [1] "Person, First" "Person, Second"
#>
#> $structured$justifications$decision1$description
#> [1] "We decided to use snowball sampling."
#>
#> $structured$justifications$decision1$alternatives
#> [1] "only invite people we know" "cast a wide net"
#>
#> $structured$justifications$decision1$choice
#> [1] 2
#>
#> $structured$justifications$decision1$justification
#> [1] "more inclusive"
#>
#> $structured$justifications$decision1$specifications
#> NULL
#>
#> $structured$justifications$decision1$fromFile
#> [1] "none"
#>
#> $structured$justifications$decision1$fromDir
#> [1] "."
#>
#> $structured$justifications$decision1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$decision1$date
#> $structured$justifications$decision1$date[[1]]
#> [1] "2018-09-12"
#>
#> $structured$justifications$decision1$date[[2]]
#> [1] "2019-03-06"
#>
#>
#>
#> $structured$justifications$decision2
#> $structured$justifications$decision2$id
#> [1] "decision2"
#>
#> $structured$justifications$decision2$`project-id`
#> [1] "wim"
#>
#> $structured$justifications$decision2$name
#> [1] "do list finalization study"
#>
#> $structured$justifications$decision2$actors
#> [1] "Kok, Gerjo" "Peters, Louk" "Crutzen, Rik"
#> [4] "Peters, Gjalt-Jorn"
#>
#> $structured$justifications$decision2$description
#> [1] "We decided to blablabla"
#>
#> $structured$justifications$decision2$alternatives
#> [1] "no" "yes"
#>
#> $structured$justifications$decision2$choice
#> [1] 2
#>
#> $structured$justifications$decision2$justification
#> [1] "more thorough"
#>
#> $structured$justifications$decision2$specifications
#> NULL
#>
#> $structured$justifications$decision2$fromFile
#> [1] "none"
#>
#> $structured$justifications$decision2$fromDir
#> [1] "."
#>
#> $structured$justifications$decision2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$decision2$date
#> $structured$justifications$decision2$date[[1]]
#> [1] "2018-09-12"
#>
#> $structured$justifications$decision2$date[[2]]
#> [1] "2019-03-06"
#>
#>
#>
#> $structured$justifications$justification_01
#> $structured$justifications$justification_01$id
#> [1] "justification_01"
#>
#> $structured$justifications$justification_01$label
#> [1] "Sleep prevents us from getting sick and (therefore) sleep deprivation causes an impaired immune system. Sleep prevents a disbalance of inflammatory cytokines and therefore health is maintained."
#>
#> $structured$justifications$justification_01$assertion
#> [1] "assertion_cytokines" "assertion_cytokines_function"
#> [3] "assertion_SD_cytokines"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_01$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_01$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_01$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_01$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_02
#> $structured$justifications$justification_02$id
#> [1] "justification_02"
#>
#> $structured$justifications$justification_02$label
#> [1] "Sleep is important for our memory and (therefore) sleep deprivation causes memory impairment."
#>
#> $structured$justifications$justification_02$assertion
#> [1] "assertion_sleep_memory_1" "assertion_sleep_memory_2"
#> [3] "assertion_sleep_memory_3" "assertion_sleep_memory_4"
#> [5] "assertion_sleep_memory_5" "assertion_sleep_memory_6"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_02$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_02$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_02$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_02$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_03
#> $structured$justifications$justification_03$id
#> [1] "justification_03"
#>
#> $structured$justifications$justification_03$label
#> [1] "Sleep protects us from toxicants and (therefore) sleep deprivation is associated with neurological diseases."
#>
#> $structured$justifications$justification_03$assertion
#> [1] "assertion_glympathic_system_1" "assertion_glympathic_system_2"
#> [3] "assertion_sleep_glympathic_system"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_03$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_03$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_03$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_03$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_04
#> $structured$justifications$justification_04$id
#> [1] "justification_04"
#>
#> $structured$justifications$justification_04$label
#> [1] "Resting is not as beneficial as sleep, but is preferred over total sleep deprivation as resting state resembles a sleep state."
#>
#> $structured$justifications$justification_04$assertion
#> [1] "assertion_resting_1" "assertion_resting_2" "assertion_resting_3"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_04$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_04$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_04$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_04$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_05
#> $structured$justifications$justification_05$id
#> [1] "justification_05"
#>
#> $structured$justifications$justification_05$label
#> [1] "Nocturnal sleep is preferred over diurnal sleep, but diurnal sleep is preferred over total sleep deprivation."
#>
#> $structured$justifications$justification_05$assertion
#> [1] "assertion_nocturnal_1" "assertion_nocturnal_2" "assertion_sleep_debt"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_05$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_05$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_05$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_05$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_06
#> $structured$justifications$justification_06$id
#> [1] "justification_06"
#>
#> $structured$justifications$justification_06$label
#> [1] "Sleep deprivation causes impaired cognitive performance."
#>
#> $structured$justifications$justification_06$assertion
#> [1] "assertion_SD_performance" "assertion_attention"
#> [3] "assertion_inattentive_behaviours"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_06$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_06$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_06$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_06$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_07
#> $structured$justifications$justification_07$id
#> [1] "justification_07"
#>
#> $structured$justifications$justification_07$label
#> [1] "Sleep deprivation causes impaired decision making."
#>
#> $structured$justifications$justification_07$assertion
#> [1] "assertion_SD_decision"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_07$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_07$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_07$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_07$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_08
#> $structured$justifications$justification_08$id
#> [1] "justification_08"
#>
#> $structured$justifications$justification_08$label
#> [1] "Sleep deprivation causes state instability."
#>
#> $structured$justifications$justification_08$assertion
#> [1] "assertion_state_instability"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_08$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_08$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_08$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_08$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_09
#> $structured$justifications$justification_09$id
#> [1] "justification_09"
#>
#> $structured$justifications$justification_09$label
#> [1] "Sleep deprivation causes impaired sensory perception."
#>
#> $structured$justifications$justification_09$assertion
#> [1] "assertion_SD_sensory_perception"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_09$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_09$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_09$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_09$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_10
#> $structured$justifications$justification_10$id
#> [1] "justification_10"
#>
#> $structured$justifications$justification_10$label
#> [1] "Sleep deprivation affects emotional perception and experience."
#>
#> $structured$justifications$justification_10$assertion
#> [1] "assertion_SD_emotion_1" "assertion_SD_emotion_2"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_10$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_10$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_10$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_10$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_11
#> $structured$justifications$justification_11$id
#> [1] "justification_11"
#>
#> $structured$justifications$justification_11$label
#> [1] "There are individual differences in the effect sleep deprivation has on people."
#>
#> $structured$justifications$justification_11$assertion
#> [1] "assertion_individual_differences_1" "assertion_individual_differences_2"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_11$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_11$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_11$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_11$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_12
#> $structured$justifications$justification_12$id
#> [1] "justification_12"
#>
#> $structured$justifications$justification_12$label
#> [1] "There is an interaction between sleep deprivation and MDMA."
#>
#> $structured$justifications$justification_12$assertion
#> [1] "assertion_MDMA_PMF_nighttime" "assertion_MDMA_PMF_daytime"
#> [3] "assertion_MDMA_impulse_nighttime" "assertion_MDMA_impulse_daytime"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_12$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_12$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_12$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_12$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_13
#> $structured$justifications$justification_13$id
#> [1] "justification_13"
#>
#> $structured$justifications$justification_13$label
#> [1] "There is an interaction between sleep deprivation and alcohol."
#>
#> $structured$justifications$justification_13$assertion
#> [1] "assertion_SD_alcohol" "assertion_interaction_SD_alcohol_1"
#> [3] "assertion_interaction_SD_alcohol_2"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_13$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_13$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_13$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_1
#> $structured$justifications$justification_1$id
#> [1] "justification_1"
#>
#> $structured$justifications$justification_1$label
#> [1] "Justification 1"
#>
#> $structured$justifications$justification_1$description
#> [1] "Description of justification 1"
#>
#> $structured$justifications$justification_1$assertion
#> [1] "assertion_1" "assertion_2"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_1$justification
#> [1] "justification_1b"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$justification_1$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_1$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_2
#> $structured$justifications$justification_2$id
#> [1] "justification_2"
#>
#> $structured$justifications$justification_2$label
#> [1] "Justification 2"
#>
#> $structured$justifications$justification_2$description
#> [1] "Description of justification 2"
#>
#> $structured$justifications$justification_2$assertion
#> [1] "assertion_2"
#>
#> $structured$justifications$justification_2$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_2$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$without_testing_users_may_ingest_contaminants
#> $structured$justifications$without_testing_users_may_ingest_contaminants$id
#> [1] "without_testing_users_may_ingest_contaminants"
#>
#> $structured$justifications$without_testing_users_may_ingest_contaminants$label
#> [1] "Some XTC pills are contaminated, and one needs to test them to be aware of the pill contents."
#>
#> $structured$justifications$without_testing_users_may_ingest_contaminants$assertion
#> [1] "some_pills_are_contaminated"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$without_testing_users_may_ingest_contaminants$fromFile
#> [1] "none"
#>
#> $structured$justifications$without_testing_users_may_ingest_contaminants$fromDir
#> [1] "."
#>
#> $structured$justifications$without_testing_users_may_ingest_contaminants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$without_testing_users_may_ingest_contaminants$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$proper_dosing_requires_knowing_dose
#> $structured$justifications$proper_dosing_requires_knowing_dose$id
#> [1] "proper_dosing_requires_knowing_dose"
#>
#> $structured$justifications$proper_dosing_requires_knowing_dose$label
#> [1] "Proper dosing (~ 1-1.5 mg of MDMA per kg of body weight) becomes hard if the dose in a pill is unknown."
#>
#> $structured$justifications$proper_dosing_requires_knowing_dose$assertion
#> [1] "dosing_requires_pill_content"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$proper_dosing_requires_knowing_dose$fromFile
#> [1] "none"
#>
#> $structured$justifications$proper_dosing_requires_knowing_dose$fromDir
#> [1] "."
#>
#> $structured$justifications$proper_dosing_requires_knowing_dose$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$proper_dosing_requires_knowing_dose$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$enough_known_about_testing_determinants
#> $structured$justifications$enough_known_about_testing_determinants$id
#> [1] "enough_known_about_testing_determinants"
#>
#> $structured$justifications$enough_known_about_testing_determinants$label
#> [1] "We have enough information available to develop a questionnaire that is likely to measure the most important determinants and sub-determinants of XTC testing."
#>
#> $structured$justifications$enough_known_about_testing_determinants$assertion
#> [1] "testing_xtc_is_reasoned"
#> [2] "there_is_qualitative_research_about_testing"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$enough_known_about_testing_determinants$fromFile
#> [1] "none"
#>
#> $structured$justifications$enough_known_about_testing_determinants$fromDir
#> [1] "."
#>
#> $structured$justifications$enough_known_about_testing_determinants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$enough_known_about_testing_determinants$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$limited_time
#> $structured$justifications$limited_time$id
#> [1] "limited_time"
#>
#> $structured$justifications$limited_time$label
#> [1] "We have to finish this study before the end of 2019."
#>
#> $structured$justifications$limited_time$assertion
#> [1] "project_deadline"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$limited_time$fromFile
#> [1] "none"
#>
#> $structured$justifications$limited_time$fromDir
#> [1] "."
#>
#> $structured$justifications$limited_time$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$limited_time$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$aipe_for_correlations
#> $structured$justifications$aipe_for_correlations$id
#> [1] "aipe_for_correlations"
#>
#> $structured$justifications$aipe_for_correlations$label
#> [1] "To estimate a correlation accurately, you need ~ 400 participants."
#>
#> $structured$justifications$aipe_for_correlations$assertion
#> [1] "nice_round_number" "exact_aipe_for_our_study"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$aipe_for_correlations$fromFile
#> [1] "none"
#>
#> $structured$justifications$aipe_for_correlations$fromDir
#> [1] "."
#>
#> $structured$justifications$aipe_for_correlations$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$aipe_for_correlations$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$low_risk_of_bias
#> $structured$justifications$low_risk_of_bias$id
#> [1] "low_risk_of_bias"
#>
#> $structured$justifications$low_risk_of_bias$label
#> [1] "Because we do not test a specific hypothesis but simply want to know how relevant the different determinants are in this population, the need to preregister is less pressing."
#>
#> $structured$justifications$low_risk_of_bias$assertion
#> [1] "prereg_to_decrease_bias"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$low_risk_of_bias$fromFile
#> [1] "none"
#>
#> $structured$justifications$low_risk_of_bias$fromDir
#> [1] "."
#>
#> $structured$justifications$low_risk_of_bias$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$low_risk_of_bias$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$no_time_for_prereg
#> $structured$justifications$no_time_for_prereg$id
#> [1] "no_time_for_prereg"
#>
#> $structured$justifications$no_time_for_prereg$label
#> [1] "We have no time to complete a preregistration form."
#>
#> $structured$justifications$no_time_for_prereg$assertion
#> [1] "project_deadline"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$justifications$no_time_for_prereg$fromFile
#> [1] "none"
#>
#> $structured$justifications$no_time_for_prereg$fromDir
#> [1] "."
#>
#> $structured$justifications$no_time_for_prereg$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$no_time_for_prereg$date
#> [1] "2019-03-06"
#>
#>
#> $structured$justifications$justification_1b
#> $structured$justifications$justification_1b$id
#> [1] "justification_1b"
#>
#> $structured$justifications$justification_1b$label
#> [1] "Justification 1b"
#>
#> $structured$justifications$justification_1b$description
#> [1] "Description of justification 1b"
#>
#> $structured$justifications$justification_1b$fromFile
#> [1] "none"
#>
#> $structured$justifications$justification_1b$fromDir
#> [1] "."
#>
#> $structured$justifications$justification_1b$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$justifications$justification_1b$date
#> [1] "2019-03-06"
#>
#>
#>
#> $structured$decisions
#> $structured$decisions$decision_1
#> $structured$decisions$decision_1$id
#> [1] "decision_1"
#>
#> $structured$decisions$decision_1$label
#> [1] "Decision 1"
#>
#> $structured$decisions$decision_1$description
#> [1] "Description of decision 1"
#>
#> $structured$decisions$decision_1$justification
#> [1] "justification_1" "justification_2"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$decisions$decision_1$fromFile
#> [1] "none"
#>
#> $structured$decisions$decision_1$fromDir
#> [1] "."
#>
#> $structured$decisions$decision_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$decision_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$decisions$decision_2
#> $structured$decisions$decision_2$id
#> [1] "decision_2"
#>
#> $structured$decisions$decision_2$label
#> [1] "Decision 2"
#>
#> $structured$decisions$decision_2$description
#> [1] "Description of decision 2"
#>
#> $structured$decisions$decision_2$alternatives
#> $structured$decisions$decision_2$alternatives[[1]]
#> $structured$decisions$decision_2$alternatives[[1]]$value
#> [1] 1
#>
#> $structured$decisions$decision_2$alternatives[[1]]$label
#> [1] "Alternative 1"
#>
#> $structured$decisions$decision_2$alternatives[[1]]$description
#> [1] "Description of alternative 1"
#>
#>
#> $structured$decisions$decision_2$alternatives[[2]]
#> $structured$decisions$decision_2$alternatives[[2]]$value
#> [1] 2
#>
#> $structured$decisions$decision_2$alternatives[[2]]$label
#> [1] "Alternative 2"
#>
#> $structured$decisions$decision_2$alternatives[[2]]$description
#> [1] "Description of alternative 2"
#>
#>
#>
#> $structured$decisions$decision_2$fromFile
#> [1] "none"
#>
#> $structured$decisions$decision_2$fromDir
#> [1] "."
#>
#> $structured$decisions$decision_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$decision_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$decisions$decision_to_select_behavior_1
#> $structured$decisions$decision_to_select_behavior_1$id
#> [1] "decision_to_select_behavior_1"
#>
#> $structured$decisions$decision_to_select_behavior_1$label
#> [1] "People should do so and so because of justifications 04,05,07,10 etc."
#>
#> $structured$decisions$decision_to_select_behavior_1$justification
#> [1] "justification_04" "justification_05" "justification_07" "justification_10"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$decisions$decision_to_select_behavior_1$fromFile
#> [1] "none"
#>
#> $structured$decisions$decision_to_select_behavior_1$fromDir
#> [1] "."
#>
#> $structured$decisions$decision_to_select_behavior_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$decision_to_select_behavior_1$date
#> [1] "2019-03-06"
#>
#>
#> $structured$decisions$decision_to_select_behavior_2
#> $structured$decisions$decision_to_select_behavior_2$id
#> [1] "decision_to_select_behavior_2"
#>
#> $structured$decisions$decision_to_select_behavior_2$label
#> [1] "People should do so and so because of"
#>
#> $structured$decisions$decision_to_select_behavior_2$justification
#> [1] "justification_01" "justification_02"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$decisions$decision_to_select_behavior_2$fromFile
#> [1] "none"
#>
#> $structured$decisions$decision_to_select_behavior_2$fromDir
#> [1] "."
#>
#> $structured$decisions$decision_to_select_behavior_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$decision_to_select_behavior_2$date
#> [1] "2019-03-06"
#>
#>
#> $structured$decisions$research_question
#> $structured$decisions$research_question$type
#> [1] "research_question"
#>
#> $structured$decisions$research_question$id
#> [1] "research_question"
#>
#> $structured$decisions$research_question$value
#> [1] "What are the most important determinants of getting one's ecstasy tested?"
#>
#> $structured$decisions$research_question$label
#> [1] "The answer to this research question is required to develop and effective interventions to promote ecstasy pill testing."
#>
#> $structured$decisions$research_question$description
#> [1] "To minimize the likelihood of incidents and accidental intoxication, testing is a required step."
#>
#> $structured$decisions$research_question$justification
#> [1] "without_testing_users_may_ingest_contaminants"
#> [2] "proper_dosing_requires_knowing_dose"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$decisions$research_question$fromFile
#> [1] "none"
#>
#> $structured$decisions$research_question$fromDir
#> [1] "."
#>
#> $structured$decisions$research_question$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$research_question$date
#> [1] "2019-03-06"
#>
#>
#> $structured$decisions$global_study_method
#> $structured$decisions$global_study_method$type
#> [1] "global_study_method"
#>
#> $structured$decisions$global_study_method$id
#> [1] "global_study_method"
#>
#> $structured$decisions$global_study_method$value
#> [1] "quantitative"
#>
#> $structured$decisions$global_study_method$label
#> [1] "We will conduct a quantitative study."
#>
#> $structured$decisions$global_study_method$description
#> [1] "Here, a decision can be explained more in detail (e.g. describing how the justifications relate to each other)."
#>
#> $structured$decisions$global_study_method$justification
#> [1] "enough_known_about_testing_determinants"
#> [2] "limited_time"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$decisions$global_study_method$fromFile
#> [1] "none"
#>
#> $structured$decisions$global_study_method$fromDir
#> [1] "."
#>
#> $structured$decisions$global_study_method$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$global_study_method$date
#> [1] "2019-03-06"
#>
#>
#> $structured$decisions$study_sample_size
#> $structured$decisions$study_sample_size$id
#> [1] "study_sample_size"
#>
#> $structured$decisions$study_sample_size$type
#> [1] "study_sample_size"
#>
#> $structured$decisions$study_sample_size$value
#> [1] 400
#>
#> $structured$decisions$study_sample_size$label
#> [1] "We aim to recruit around 400 participants."
#>
#> $structured$decisions$study_sample_size$justification
#> [1] "aipe_for_correlations"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$decisions$study_sample_size$fromFile
#> [1] "none"
#>
#> $structured$decisions$study_sample_size$fromDir
#> [1] "."
#>
#> $structured$decisions$study_sample_size$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$study_sample_size$date
#> [1] "2019-03-06"
#>
#>
#> $structured$decisions$preregistration
#> $structured$decisions$preregistration$id
#> [1] "preregistration"
#>
#> $structured$decisions$preregistration$type
#> [1] "preregistration"
#>
#> $structured$decisions$preregistration$value
#> [1] FALSE
#>
#> $structured$decisions$preregistration$label
#> [1] "We will not preregister this study."
#>
#> $structured$decisions$preregistration$justification
#> [1] "low_risk_of_bias" "no_time_for_prereg"
#> attr(,"class")
#> [1] "justifierRef"
#>
#> $structured$decisions$preregistration$fromFile
#> [1] "none"
#>
#> $structured$decisions$preregistration$fromDir
#> [1] "."
#>
#> $structured$decisions$preregistration$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $structured$decisions$preregistration$date
#> [1] "2019-03-06"
#>
#>
#>
#> $structured$justifier
#> $structured$justifier$justifier_example_study_framework_specification
#> $id
#> [1] "justifier_example_study_framework_specification"
#>
#> $label
#> [1] "Justifier example: Study framework specification"
#>
#> $description
#> [1] "This framework is an example of how different decisions in the planning of a psychological study could be contextualized."
#>
#> $context
#> $context[[1]]
#> $context[[1]]$id
#> [1] "global_planning"
#>
#> $context[[1]]$importedContextFramework
#> [1] "some_other_framework_specification"
#>
#> $context[[1]]$importedContextId
#> [1] "global_planning"
#>
#> $context[[1]]$sequence
#> [1] 1
#>
#>
#> $context[[2]]
#> $context[[2]]$id
#> [1] "design"
#>
#> $context[[2]]$sequence
#> [1] 2
#>
#> $context[[2]]$label
#> [1] "Decisions related to design"
#>
#>
#> $context[[3]]
#> $context[[3]]$id
#> [1] "sample_size"
#>
#> $context[[3]]$sequence
#> [1] 3
#>
#>
#> $context[[4]]
#> $context[[4]]$id
#> [1] "operationalizations"
#>
#> $context[[4]]$sequence
#> [1] 4
#>
#>
#>
#> $condition
#> $condition[[1]]
#> $condition[[1]]$id
#> [1] "global_method_specification"
#>
#> $condition[[1]]$contextId
#> [1] "global_planning"
#>
#> $condition[[1]]$type
#> [1] "global_method_specification"
#>
#> $condition[[1]]$element
#> [1] "decision"
#>
#> $condition[[1]]$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $condition[[1]]$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#> $condition[[2]]
#> $condition[[2]]$id
#> [1] "study_sample_size"
#>
#> $condition[[2]]$contextId
#> [1] "sample_size"
#>
#> $condition[[2]]$type
#> [1] "study_sample_size"
#>
#> $condition[[2]]$element
#> [1] "decision"
#>
#> $condition[[2]]$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#> $condition[[3]]
#> $condition[[3]]$id
#> [1] "included_variables"
#>
#> $condition[[3]]$contextid
#> [1] "design"
#>
#> $condition[[3]]$type
#> [1] "included_variables"
#>
#> $condition[[3]]$element
#> [1] "decision"
#>
#>
#> $condition[[4]]
#> $condition[[4]]$id
#> [1] "source_types"
#>
#> $condition[[4]]$element
#> [1] "source"
#>
#> $condition[[4]]$field
#> [1] "evidence_type"
#>
#> $condition[[4]]$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $condition[[4]]$scores
#> [1] 1 2 3 4 5 6
#>
#> $condition[[4]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#>
#> $condition[[5]]
#> $condition[[5]]$id
#> [1] "source_year"
#>
#> $condition[[5]]$element
#> [1] "source"
#>
#> $condition[[5]]$field
#> [1] "year"
#>
#> $condition[[5]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $condition[[5]]$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#>
#>
#> $fromFile
#> [1] "none"
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#> $structured$justifier[[2]]
#> $scope
#> [1] "global"
#>
#> $framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $fromFile
#> [1] "none"
#>
#> $id
#> [1] ""
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#> $structured$justifier[[3]]
#> $scope
#> [1] "local"
#>
#> $date
#> [1] "2019-03-06"
#>
#> $fromFile
#> [1] "none"
#>
#> $id
#> [1] ""
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#>
#>
#> $logging
#> $logging$n_firstSweep
#> sources assertions justifications decisions justifier
#> 31 40 15 8 3
#>
#>
#> $frameworks
#> $frameworks$specs
#> $frameworks$specs[[1]]
#> framework
#> "justifier-example-study-framework-specification.jmd"
#> scope
#> "global"
#> fromFile
#> "none"
#>
#>
#> $frameworks$loaded
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$id
#> [1] "justifier_example_study_framework_specification"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$label
#> [1] "Justifier example: Study framework specification"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$description
#> [1] "This framework is an example of how different decisions in the planning of a psychological study could be contextualized."
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[1]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[1]]$id
#> [1] "global_planning"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[1]]$importedContextFramework
#> [1] "some_other_framework_specification"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[1]]$importedContextId
#> [1] "global_planning"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[1]]$sequence
#> [1] 1
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[2]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[2]]$id
#> [1] "design"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[2]]$sequence
#> [1] 2
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[2]]$label
#> [1] "Decisions related to design"
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[3]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[3]]$id
#> [1] "sample_size"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[3]]$sequence
#> [1] 3
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[4]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[4]]$id
#> [1] "operationalizations"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$context[[4]]$sequence
#> [1] 4
#>
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[1]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[1]]$id
#> [1] "global_method_specification"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[1]]$contextId
#> [1] "global_planning"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[1]]$type
#> [1] "global_method_specification"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[1]]$element
#> [1] "decision"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[1]]$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[1]]$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[2]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[2]]$id
#> [1] "study_sample_size"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[2]]$contextId
#> [1] "sample_size"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[2]]$type
#> [1] "study_sample_size"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[2]]$element
#> [1] "decision"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[2]]$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[3]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[3]]$id
#> [1] "included_variables"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[3]]$contextid
#> [1] "design"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[3]]$type
#> [1] "included_variables"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[3]]$element
#> [1] "decision"
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[4]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[4]]$id
#> [1] "source_types"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[4]]$element
#> [1] "source"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[4]]$field
#> [1] "evidence_type"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[4]]$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[4]]$scores
#> [1] 1 2 3 4 5 6
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[4]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[5]]
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[5]]$id
#> [1] "source_year"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[5]]$element
#> [1] "source"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[5]]$field
#> [1] "year"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[5]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $frameworks$loaded$`justifier-example-study-framework-specification.jmd`$justifier$condition[[5]]$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#>
#>
#>
#>
#> $frameworks$loaded[[2]]
#> $frameworks$loaded[[2]]$justifier
#> $id
#> [1] "justifier_example_study_framework_specification"
#>
#> $label
#> [1] "Justifier example: Study framework specification"
#>
#> $description
#> [1] "This framework is an example of how different decisions in the planning of a psychological study could be contextualized."
#>
#> $context
#> $context[[1]]
#> $context[[1]]$id
#> [1] "global_planning"
#>
#> $context[[1]]$importedContextFramework
#> [1] "some_other_framework_specification"
#>
#> $context[[1]]$importedContextId
#> [1] "global_planning"
#>
#> $context[[1]]$sequence
#> [1] 1
#>
#>
#> $context[[2]]
#> $context[[2]]$id
#> [1] "design"
#>
#> $context[[2]]$sequence
#> [1] 2
#>
#> $context[[2]]$label
#> [1] "Decisions related to design"
#>
#>
#> $context[[3]]
#> $context[[3]]$id
#> [1] "sample_size"
#>
#> $context[[3]]$sequence
#> [1] 3
#>
#>
#> $context[[4]]
#> $context[[4]]$id
#> [1] "operationalizations"
#>
#> $context[[4]]$sequence
#> [1] 4
#>
#>
#>
#> $condition
#> $condition[[1]]
#> $condition[[1]]$id
#> [1] "global_method_specification"
#>
#> $condition[[1]]$contextId
#> [1] "global_planning"
#>
#> $condition[[1]]$type
#> [1] "global_method_specification"
#>
#> $condition[[1]]$element
#> [1] "decision"
#>
#> $condition[[1]]$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $condition[[1]]$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#> $condition[[2]]
#> $condition[[2]]$id
#> [1] "study_sample_size"
#>
#> $condition[[2]]$contextId
#> [1] "sample_size"
#>
#> $condition[[2]]$type
#> [1] "study_sample_size"
#>
#> $condition[[2]]$element
#> [1] "decision"
#>
#> $condition[[2]]$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#> $condition[[3]]
#> $condition[[3]]$id
#> [1] "included_variables"
#>
#> $condition[[3]]$contextid
#> [1] "design"
#>
#> $condition[[3]]$type
#> [1] "included_variables"
#>
#> $condition[[3]]$element
#> [1] "decision"
#>
#>
#> $condition[[4]]
#> $condition[[4]]$id
#> [1] "source_types"
#>
#> $condition[[4]]$element
#> [1] "source"
#>
#> $condition[[4]]$field
#> [1] "evidence_type"
#>
#> $condition[[4]]$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $condition[[4]]$scores
#> [1] 1 2 3 4 5 6
#>
#> $condition[[4]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#>
#> $condition[[5]]
#> $condition[[5]]$id
#> [1] "source_year"
#>
#> $condition[[5]]$element
#> [1] "source"
#>
#> $condition[[5]]$field
#> [1] "year"
#>
#> $condition[[5]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $condition[[5]]$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#>
#>
#> $fromFile
#> [1] "none"
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#>
#> $frameworks$loaded[[3]]
#> $frameworks$loaded[[3]]$justifier
#> $scope
#> [1] "global"
#>
#> $framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $fromFile
#> [1] "none"
#>
#> $id
#> [1] ""
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#>
#> $frameworks$loaded[[4]]
#> $frameworks$loaded[[4]]$justifier
#> $scope
#> [1] "local"
#>
#> $date
#> [1] "2019-03-06"
#>
#> $fromFile
#> [1] "none"
#>
#> $id
#> [1] ""
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#>
#>
#> $frameworks$parsed
#> $frameworks$parsed$justifier_example_study_framework_specification
#> $id
#> [1] "justifier_example_study_framework_specification"
#>
#> $label
#> [1] "Justifier example: Study framework specification"
#>
#> $description
#> [1] "This framework is an example of how different decisions in the planning of a psychological study could be contextualized."
#>
#> $contextSpecifications
#> $contextSpecifications[[1]]
#> $contextSpecifications[[1]]$id
#> [1] "global_planning"
#>
#> $contextSpecifications[[1]]$importedContextFramework
#> [1] "some_other_framework_specification"
#>
#> $contextSpecifications[[1]]$importedContextId
#> [1] "global_planning"
#>
#> $contextSpecifications[[1]]$sequence
#> [1] 1
#>
#>
#> $contextSpecifications[[2]]
#> $contextSpecifications[[2]]$id
#> [1] "design"
#>
#> $contextSpecifications[[2]]$sequence
#> [1] 2
#>
#> $contextSpecifications[[2]]$label
#> [1] "Decisions related to design"
#>
#>
#> $contextSpecifications[[3]]
#> $contextSpecifications[[3]]$id
#> [1] "sample_size"
#>
#> $contextSpecifications[[3]]$sequence
#> [1] 3
#>
#>
#> $contextSpecifications[[4]]
#> $contextSpecifications[[4]]$id
#> [1] "operationalizations"
#>
#> $contextSpecifications[[4]]$sequence
#> [1] 4
#>
#>
#>
#> $conditionSpecifications
#> $conditionSpecifications[[1]]
#> $conditionSpecifications[[1]]$id
#> [1] "global_method_specification"
#>
#> $conditionSpecifications[[1]]$contextId
#> [1] "global_planning"
#>
#> $conditionSpecifications[[1]]$type
#> [1] "global_method_specification"
#>
#> $conditionSpecifications[[1]]$element
#> [1] "decision"
#>
#> $conditionSpecifications[[1]]$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $conditionSpecifications[[1]]$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#> $conditionSpecifications[[2]]
#> $conditionSpecifications[[2]]$id
#> [1] "study_sample_size"
#>
#> $conditionSpecifications[[2]]$contextId
#> [1] "sample_size"
#>
#> $conditionSpecifications[[2]]$type
#> [1] "study_sample_size"
#>
#> $conditionSpecifications[[2]]$element
#> [1] "decision"
#>
#> $conditionSpecifications[[2]]$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#> $conditionSpecifications[[3]]
#> $conditionSpecifications[[3]]$id
#> [1] "included_variables"
#>
#> $conditionSpecifications[[3]]$contextid
#> [1] "design"
#>
#> $conditionSpecifications[[3]]$type
#> [1] "included_variables"
#>
#> $conditionSpecifications[[3]]$element
#> [1] "decision"
#>
#>
#> $conditionSpecifications[[4]]
#> $conditionSpecifications[[4]]$id
#> [1] "source_types"
#>
#> $conditionSpecifications[[4]]$element
#> [1] "source"
#>
#> $conditionSpecifications[[4]]$field
#> [1] "evidence_type"
#>
#> $conditionSpecifications[[4]]$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $conditionSpecifications[[4]]$scores
#> [1] 1 2 3 4 5 6
#>
#> $conditionSpecifications[[4]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#> $conditionSpecifications[[4]]$type
#> [1] ".*"
#>
#>
#> $conditionSpecifications[[5]]
#> $conditionSpecifications[[5]]$id
#> [1] "source_year"
#>
#> $conditionSpecifications[[5]]$element
#> [1] "source"
#>
#> $conditionSpecifications[[5]]$field
#> [1] "year"
#>
#> $conditionSpecifications[[5]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $conditionSpecifications[[5]]$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#> $conditionSpecifications[[5]]$type
#> [1] ".*"
#>
#>
#>
#> $contexts
#> id sequence label
#> 1 global_planning 1 <NA>
#> 2 design 2 Decisions related to design
#> 3 sample_size 3 <NA>
#> 4 operationalizations 4 <NA>
#>
#> $conditions
#> $conditions$decision
#> $conditions$decision$global_method_specification
#> $conditions$decision$global_method_specification$global_method_specification
#> $conditions$decision$global_method_specification$global_method_specification$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $conditions$decision$global_method_specification$global_method_specification$contextId
#> [1] "global_planning"
#>
#> $conditions$decision$global_method_specification$global_method_specification$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#>
#> $conditions$decision$study_sample_size
#> $conditions$decision$study_sample_size$study_sample_size
#> $conditions$decision$study_sample_size$study_sample_size$contextId
#> [1] "sample_size"
#>
#> $conditions$decision$study_sample_size$study_sample_size$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#>
#> $conditions$decision$included_variables
#> $conditions$decision$included_variables$included_variables
#> list()
#>
#>
#>
#> $conditions$justification
#> list()
#>
#> $conditions$assertion
#> list()
#>
#> $conditions$source
#> $conditions$source$`.*`
#> $conditions$source$`.*`$source_types
#> $conditions$source$`.*`$source_types$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $conditions$source$`.*`$source_types$scores
#> [1] 1 2 3 4 5 6
#>
#> $conditions$source$`.*`$source_types$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#> $conditions$source$`.*`$source_types$field
#> [1] "evidence_type"
#>
#>
#> $conditions$source$`.*`$source_year
#> $conditions$source$`.*`$source_year$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $conditions$source$`.*`$source_year$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#> $conditions$source$`.*`$source_year$field
#> [1] "year"
#>
#>
#>
#>
#>
#> attr(,"class")
#> [1] "justifierFramework" "list"
#>
#> $frameworks$parsed$justifier_example_study_framework_specification
#> $id
#> [1] "justifier_example_study_framework_specification"
#>
#> $label
#> [1] "Justifier example: Study framework specification"
#>
#> $description
#> [1] "This framework is an example of how different decisions in the planning of a psychological study could be contextualized."
#>
#> $contextSpecifications
#> $contextSpecifications[[1]]
#> $contextSpecifications[[1]]$id
#> [1] "global_planning"
#>
#> $contextSpecifications[[1]]$importedContextFramework
#> [1] "some_other_framework_specification"
#>
#> $contextSpecifications[[1]]$importedContextId
#> [1] "global_planning"
#>
#> $contextSpecifications[[1]]$sequence
#> [1] 1
#>
#>
#> $contextSpecifications[[2]]
#> $contextSpecifications[[2]]$id
#> [1] "design"
#>
#> $contextSpecifications[[2]]$sequence
#> [1] 2
#>
#> $contextSpecifications[[2]]$label
#> [1] "Decisions related to design"
#>
#>
#> $contextSpecifications[[3]]
#> $contextSpecifications[[3]]$id
#> [1] "sample_size"
#>
#> $contextSpecifications[[3]]$sequence
#> [1] 3
#>
#>
#> $contextSpecifications[[4]]
#> $contextSpecifications[[4]]$id
#> [1] "operationalizations"
#>
#> $contextSpecifications[[4]]$sequence
#> [1] 4
#>
#>
#>
#> $conditionSpecifications
#> $conditionSpecifications[[1]]
#> $conditionSpecifications[[1]]$id
#> [1] "global_method_specification"
#>
#> $conditionSpecifications[[1]]$contextId
#> [1] "global_planning"
#>
#> $conditionSpecifications[[1]]$type
#> [1] "global_method_specification"
#>
#> $conditionSpecifications[[1]]$element
#> [1] "decision"
#>
#> $conditionSpecifications[[1]]$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $conditionSpecifications[[1]]$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#> $conditionSpecifications[[2]]
#> $conditionSpecifications[[2]]$id
#> [1] "study_sample_size"
#>
#> $conditionSpecifications[[2]]$contextId
#> [1] "sample_size"
#>
#> $conditionSpecifications[[2]]$type
#> [1] "study_sample_size"
#>
#> $conditionSpecifications[[2]]$element
#> [1] "decision"
#>
#> $conditionSpecifications[[2]]$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#> $conditionSpecifications[[3]]
#> $conditionSpecifications[[3]]$id
#> [1] "included_variables"
#>
#> $conditionSpecifications[[3]]$contextid
#> [1] "design"
#>
#> $conditionSpecifications[[3]]$type
#> [1] "included_variables"
#>
#> $conditionSpecifications[[3]]$element
#> [1] "decision"
#>
#>
#> $conditionSpecifications[[4]]
#> $conditionSpecifications[[4]]$id
#> [1] "source_types"
#>
#> $conditionSpecifications[[4]]$element
#> [1] "source"
#>
#> $conditionSpecifications[[4]]$field
#> [1] "evidence_type"
#>
#> $conditionSpecifications[[4]]$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $conditionSpecifications[[4]]$scores
#> [1] 1 2 3 4 5 6
#>
#> $conditionSpecifications[[4]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#> $conditionSpecifications[[4]]$type
#> [1] ".*"
#>
#>
#> $conditionSpecifications[[5]]
#> $conditionSpecifications[[5]]$id
#> [1] "source_year"
#>
#> $conditionSpecifications[[5]]$element
#> [1] "source"
#>
#> $conditionSpecifications[[5]]$field
#> [1] "year"
#>
#> $conditionSpecifications[[5]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $conditionSpecifications[[5]]$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#> $conditionSpecifications[[5]]$type
#> [1] ".*"
#>
#>
#>
#> $contexts
#> id sequence label
#> 1 global_planning 1 <NA>
#> 2 design 2 Decisions related to design
#> 3 sample_size 3 <NA>
#> 4 operationalizations 4 <NA>
#>
#> $conditions
#> $conditions$decision
#> $conditions$decision$global_method_specification
#> $conditions$decision$global_method_specification$global_method_specification
#> $conditions$decision$global_method_specification$global_method_specification$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $conditions$decision$global_method_specification$global_method_specification$contextId
#> [1] "global_planning"
#>
#> $conditions$decision$global_method_specification$global_method_specification$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#>
#> $conditions$decision$study_sample_size
#> $conditions$decision$study_sample_size$study_sample_size
#> $conditions$decision$study_sample_size$study_sample_size$contextId
#> [1] "sample_size"
#>
#> $conditions$decision$study_sample_size$study_sample_size$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#>
#> $conditions$decision$included_variables
#> $conditions$decision$included_variables$included_variables
#> list()
#>
#>
#>
#> $conditions$justification
#> list()
#>
#> $conditions$assertion
#> list()
#>
#> $conditions$source
#> $conditions$source$`.*`
#> $conditions$source$`.*`$source_types
#> $conditions$source$`.*`$source_types$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $conditions$source$`.*`$source_types$scores
#> [1] 1 2 3 4 5 6
#>
#> $conditions$source$`.*`$source_types$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#> $conditions$source$`.*`$source_types$field
#> [1] "evidence_type"
#>
#>
#> $conditions$source$`.*`$source_year
#> $conditions$source$`.*`$source_year$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $conditions$source$`.*`$source_year$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#> $conditions$source$`.*`$source_year$field
#> [1] "year"
#>
#>
#>
#>
#>
#> attr(,"class")
#> [1] "justifierFramework" "list"
#>
#> $frameworks$parsed[[3]]
#> $id
#> [1] ""
#>
#> $label
#> character(0)
#>
#> $description
#> character(0)
#>
#> $contextSpecifications
#> list()
#>
#> $conditionSpecifications
#> list()
#>
#> $contexts
#> data frame with 0 columns and 0 rows
#>
#> $conditions
#> $conditions$decision
#> list()
#>
#> $conditions$justification
#> list()
#>
#> $conditions$assertion
#> list()
#>
#> $conditions$source
#> list()
#>
#>
#> attr(,"class")
#> [1] "justifierFramework" "list"
#>
#> $frameworks$parsed[[4]]
#> $id
#> [1] ""
#>
#> $label
#> character(0)
#>
#> $description
#> character(0)
#>
#> $contextSpecifications
#> list()
#>
#> $conditionSpecifications
#> list()
#>
#> $contexts
#> data frame with 0 columns and 0 rows
#>
#> $conditions
#> $conditions$decision
#> list()
#>
#> $conditions$justification
#> list()
#>
#> $conditions$assertion
#> list()
#>
#> $conditions$source
#> list()
#>
#>
#> attr(,"class")
#> [1] "justifierFramework" "list"
#>
#>
#>
#> $fwApplications
#> $fwApplications$justifier_example_study_framework_specification
#> $fwApplications$justifier_example_study_framework_specification$verifications
#> element id type
#> 1 decision study_sample_size study_sample_size
#> 2 source source_Diekelmann .*
#> 3 source source_Gais .*
#> 4 source source_Menz .*
#> 5 source source_Holland .*
#> 6 source source_Graves .*
#> 7 source source_Frey .*
#> 8 source source_Xie .*
#> 9 source source_Brokaw .*
#> 10 source source_Marrosu .*
#> 11 source source_Lange .*
#> 12 source source_Burgess .*
#> 13 source source_Plog .*
#> 14 source source_Killgore13 .*
#> 15 source source_Harrison .*
#> 16 source source_Doran .*
#> 17 source source_Killgore07 .*
#> 18 source source_VanDongen .*
#> 19 source source_Kuypers .*
#> 20 source source_Williamson .*
#> 21 source source_Peeke .*
#> 22 source source_Croes .*
#> 23 source source_Berger .*
#> 24 source source_Fairclough .*
#> 25 source source_Krueger .*
#> 26 source source_Jessen .*
#> 27 source source_Lim .*
#> 28 source source_Tononi .*
#> 29 source source_Beebe .*
#> 30 source source_Harrison .*
#> 31 source phd_peters_xtc_determinants .*
#> 32 source phd_vervaeke_xtc_social .*
#> 33 source src:phd_peters_determinant_xtc_use .*
#> 34 source src:phd_vervaeke_xtc_social .*
#> 35 source source_1 .*
#> 36 source source_2 .*
#> 37 source source_3 .*
#> 38 source brunt_2012 .*
#> 39 source project_proposal .*
#> 40 source nice_round_numbers_are_nice .*
#> 41 source moinester_gottfried_2014 .*
#> 42 source example_source .*
#> 43 source source_Diekelmann .*
#> 44 source source_Gais .*
#> 45 source source_Menz .*
#> 46 source source_Holland .*
#> 47 source source_Graves .*
#> 48 source source_Frey .*
#> 49 source source_Xie .*
#> 50 source source_Brokaw .*
#> 51 source source_Marrosu .*
#> 52 source source_Lange .*
#> 53 source source_Burgess .*
#> 54 source source_Plog .*
#> 55 source source_Killgore13 .*
#> 56 source source_Harrison .*
#> 57 source source_Doran .*
#> 58 source source_Killgore07 .*
#> 59 source source_VanDongen .*
#> 60 source source_Kuypers .*
#> 61 source source_Williamson .*
#> 62 source source_Peeke .*
#> 63 source source_Croes .*
#> 64 source source_Berger .*
#> 65 source source_Fairclough .*
#> 66 source source_Krueger .*
#> 67 source source_Jessen .*
#> 68 source source_Lim .*
#> 69 source source_Tononi .*
#> 70 source source_Beebe .*
#> 71 source source_Harrison .*
#> 72 source phd_peters_xtc_determinants .*
#> 73 source phd_vervaeke_xtc_social .*
#> 74 source src:phd_peters_determinant_xtc_use .*
#> 75 source src:phd_vervaeke_xtc_social .*
#> 76 source source_1 .*
#> 77 source source_2 .*
#> 78 source source_3 .*
#> 79 source brunt_2012 .*
#> 80 source project_proposal .*
#> 81 source nice_round_numbers_are_nice .*
#> 82 source moinester_gottfried_2014 .*
#> 83 source example_source .*
#> condition
#> 1 study_sample_size
#> 2 source_types
#> 3 source_types
#> 4 source_types
#> 5 source_types
#> 6 source_types
#> 7 source_types
#> 8 source_types
#> 9 source_types
#> 10 source_types
#> 11 source_types
#> 12 source_types
#> 13 source_types
#> 14 source_types
#> 15 source_types
#> 16 source_types
#> 17 source_types
#> 18 source_types
#> 19 source_types
#> 20 source_types
#> 21 source_types
#> 22 source_types
#> 23 source_types
#> 24 source_types
#> 25 source_types
#> 26 source_types
#> 27 source_types
#> 28 source_types
#> 29 source_types
#> 30 source_types
#> 31 source_types
#> 32 source_types
#> 33 source_types
#> 34 source_types
#> 35 source_types
#> 36 source_types
#> 37 source_types
#> 38 source_types
#> 39 source_types
#> 40 source_types
#> 41 source_types
#> 42 source_types
#> 43 source_year
#> 44 source_year
#> 45 source_year
#> 46 source_year
#> 47 source_year
#> 48 source_year
#> 49 source_year
#> 50 source_year
#> 51 source_year
#> 52 source_year
#> 53 source_year
#> 54 source_year
#> 55 source_year
#> 56 source_year
#> 57 source_year
#> 58 source_year
#> 59 source_year
#> 60 source_year
#> 61 source_year
#> 62 source_year
#> 63 source_year
#> 64 source_year
#> 65 source_year
#> 66 source_year
#> 67 source_year
#> 68 source_year
#> 69 source_year
#> 70 source_year
#> 71 source_year
#> 72 source_year
#> 73 source_year
#> 74 source_year
#> 75 source_year
#> 76 source_year
#> 77 source_year
#> 78 source_year
#> 79 source_year
#> 80 source_year
#> 81 source_year
#> 82 source_year
#> 83 source_year
#> verification
#> 1 is.numeric(THIS_SPECIFICATION$value)
#> 2 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 3 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 4 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 5 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 6 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 7 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 8 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 9 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 10 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 11 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 12 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 13 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 14 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 15 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 16 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 17 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 18 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 19 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 20 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 21 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 22 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 23 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 24 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 25 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 26 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 27 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 28 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 29 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 30 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 31 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 32 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 33 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 34 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 35 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 36 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 37 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 38 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 39 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 40 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 41 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 42 is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)
#> 43 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 44 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 45 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 46 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 47 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 48 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 49 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 50 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 51 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 52 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 53 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 54 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 55 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 56 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 57 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 58 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 59 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 60 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 61 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 62 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 63 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 64 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 65 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 66 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 67 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 68 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 69 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 70 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 71 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 72 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 73 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 74 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 75 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 76 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 77 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 78 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 79 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 80 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 81 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 82 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> 83 is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))
#> result
#> 1 OK
#> 2 OK
#> 3 OK
#> 4 OK
#> 5 OK
#> 6 OK
#> 7 OK
#> 8 OK
#> 9 OK
#> 10 OK
#> 11 OK
#> 12 OK
#> 13 OK
#> 14 OK
#> 15 OK
#> 16 OK
#> 17 OK
#> 18 OK
#> 19 OK
#> 20 OK
#> 21 OK
#> 22 OK
#> 23 OK
#> 24 OK
#> 25 OK
#> 26 OK
#> 27 OK
#> 28 OK
#> 29 OK
#> 30 OK
#> 31 OK
#> 32 OK
#> 33 OK
#> 34 OK
#> 35 OK
#> 36 OK
#> 37 OK
#> 38 OK
#> 39 OK
#> 40 OK
#> 41 OK
#> 42 OK
#> 43 OK
#> 44 OK
#> 45 OK
#> 46 OK
#> 47 OK
#> 48 OK
#> 49 OK
#> 50 OK
#> 51 OK
#> 52 OK
#> 53 OK
#> 54 OK
#> 55 OK
#> 56 OK
#> 57 OK
#> 58 OK
#> 59 OK
#> 60 OK
#> 61 OK
#> 62 OK
#> 63 OK
#> 64 OK
#> 65 OK
#> 66 OK
#> 67 OK
#> 68 OK
#> 69 OK
#> 70 OK
#> 71 OK
#> 72 OK
#> 73 OK
#> 74 OK
#> 75 OK
#> 76 OK
#> 77 OK
#> 78 OK
#> 79 OK
#> 80 OK
#> 81 The year you specify in a source must consist of exactly four digits.
#> 82 OK
#> 83 OK
#>
#> $fwApplications$justifier_example_study_framework_specification$fieldScoring
#> element id type condition field
#> 1 source brunt_2012 .* source_types evidence_type
#> 2 source nice_round_numbers_are_nice .* source_types evidence_type
#> 3 source moinester_gottfried_2014 .* source_types evidence_type
#> 4 source example_source .* source_types evidence_type
#> value score
#> 1 empirical study 5
#> 2 team opinion 1
#> 3 theory 4
#> 4 empirical study 5
#>
#> $fwApplications$justifier_example_study_framework_specification$scoreDf
#> decision field score
#> 1 decision_1 evidence_type NA
#> 2 decision_2 evidence_type NA
#> 3 decision_to_select_behavior_1 evidence_type NA
#> 4 decision_to_select_behavior_2 evidence_type NA
#> 5 research_question evidence_type 5.0
#> 6 global_study_method evidence_type NA
#> 7 study_sample_size evidence_type 2.5
#> 8 preregistration evidence_type 5.0
#>
#>
#> $fwApplications[[2]]
#> $fwApplications[[2]]$verifications
#> data frame with 0 columns and 0 rows
#>
#> $fwApplications[[2]]$fieldScoring
#> data frame with 0 columns and 0 rows
#>
#>
#> $fwApplications[[3]]
#> $fwApplications[[3]]$verifications
#> data frame with 0 columns and 0 rows
#>
#> $fwApplications[[3]]$fieldScoring
#> data frame with 0 columns and 0 rows
#>
#>
#> $fwApplications[[4]]
#> $fwApplications[[4]]$scoreDf
#> data frame with 0 columns and 0 rows
#>
#>
#> $fwApplications[[5]]
#> $fwApplications[[5]]$scoreDf
#> data frame with 0 columns and 0 rows
#>
#>
#>
#> $supplemented
#> $supplemented$sources
#> $supplemented$sources$source_Diekelmann
#> $supplemented$sources$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $supplemented$sources$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $supplemented$sources$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $supplemented$sources$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $supplemented$sources$source_Diekelmann$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Gais
#> $supplemented$sources$source_Gais$id
#> [1] "source_Gais"
#>
#> $supplemented$sources$source_Gais$label
#> [1] "Gais (2003) Sleep after learning aids memory recall."
#>
#> $supplemented$sources$source_Gais$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $supplemented$sources$source_Gais$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Gais$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Gais$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Gais$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Gais$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Menz
#> $supplemented$sources$source_Menz$id
#> [1] "source_Menz"
#>
#> $supplemented$sources$source_Menz$label
#> [1] "Menz et al (2013) The role of sleep and sleep deprivation in consolidating fear memories."
#>
#> $supplemented$sources$source_Menz$xdoi
#> [1] "doi:10.1016/j.neuroimage.2013.03.001"
#>
#> $supplemented$sources$source_Menz$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Menz$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Menz$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Menz$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Menz$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Holland
#> $supplemented$sources$source_Holland$id
#> [1] "source_Holland"
#>
#> $supplemented$sources$source_Holland$label
#> [1] "Holland & Lewis (2007) Emotional memory: selective enhancement by sleep."
#>
#> $supplemented$sources$source_Holland$xdoi
#> [1] "doi:10.1016/j.cub.2006.12.033"
#>
#> $supplemented$sources$source_Holland$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Holland$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Holland$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Holland$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Holland$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Graves
#> $supplemented$sources$source_Graves$id
#> [1] "source_Graves"
#>
#> $supplemented$sources$source_Graves$label
#> [1] "Graves (2003) Sleep deprivation selectively impairs memory consolidation for contextual fear conditioning."
#>
#> $supplemented$sources$source_Graves$xdoi
#> [1] "doi:10.1101/lm.48803"
#>
#> $supplemented$sources$source_Graves$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Graves$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Graves$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Graves$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Graves$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Frey
#> $supplemented$sources$source_Frey$id
#> [1] "source_Frey"
#>
#> $supplemented$sources$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $supplemented$sources$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $supplemented$sources$source_Frey$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Frey$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Frey$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Xie
#> $supplemented$sources$source_Xie$id
#> [1] "source_Xie"
#>
#> $supplemented$sources$source_Xie$label
#> [1] "Zie et al (2013) ). Sleep drives metabolite clearance from the adult brain."
#>
#> $supplemented$sources$source_Xie$xdoi
#> [1] "doi:10.1126/science.1241224"
#>
#> $supplemented$sources$source_Xie$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Xie$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Xie$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Xie$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Xie$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Brokaw
#> $supplemented$sources$source_Brokaw$id
#> [1] "source_Brokaw"
#>
#> $supplemented$sources$source_Brokaw$label
#> [1] "Brokaw et al (2016) Resting state EEG correlates of memory consolidation."
#>
#> $supplemented$sources$source_Brokaw$xdoi
#> [1] "doi:10.1016/j.nlm.2016.01.008"
#>
#> $supplemented$sources$source_Brokaw$pp
#> [1] "17-25"
#>
#> $supplemented$sources$source_Brokaw$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Brokaw$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Brokaw$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Brokaw$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Brokaw$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Marrosu
#> $supplemented$sources$source_Marrosu$id
#> [1] "source_Marrosu"
#>
#> $supplemented$sources$source_Marrosu$label
#> [1] "Marrosu et al (1995) Microdialysis measurement of cortical and hippocampal acetylcholine release during sleep-wake cycle in freely moving cats."
#>
#> $supplemented$sources$source_Marrosu$xdoi
#> [1] "No DOI found yet, located in: Brain Research, vol 671, pp 329–332."
#>
#> $supplemented$sources$source_Marrosu$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Marrosu$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Marrosu$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Marrosu$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Marrosu$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Lange
#> $supplemented$sources$source_Lange$id
#> [1] "source_Lange"
#>
#> $supplemented$sources$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $supplemented$sources$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $supplemented$sources$source_Lange$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Lange$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Lange$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Burgess
#> $supplemented$sources$source_Burgess$id
#> [1] "source_Burgess"
#>
#> $supplemented$sources$source_Burgess$label
#> [1] "Burgess et al (2002) ). Bright light, dark and melatonin can promote circadian adaptation in night shift workers."
#>
#> $supplemented$sources$source_Burgess$xdoi
#> [1] "doi:10.1053/smrv.2001.0215"
#>
#> $supplemented$sources$source_Burgess$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Burgess$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Burgess$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Burgess$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Burgess$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Plog
#> $supplemented$sources$source_Plog$id
#> [1] "source_Plog"
#>
#> $supplemented$sources$source_Plog$label
#> [1] "Plog & Nedergaard (2018) The glymphatic system in central nervous system health and disease: past, present, and future"
#>
#> $supplemented$sources$source_Plog$xdoi
#> [1] "doi:10.1146/annurev-pathol-051217-111018"
#>
#> $supplemented$sources$source_Plog$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Plog$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Plog$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Plog$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Plog$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Killgore13
#> $supplemented$sources$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$sources$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$sources$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$sources$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Harrison
#> $supplemented$sources$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$sources$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$sources$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$sources$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Doran
#> $supplemented$sources$source_Doran$id
#> [1] "source_Doran"
#>
#> $supplemented$sources$source_Doran$label
#> [1] "Doran et al (2001) Sustained attention performance during sleep deprivation: evidence of state instability."
#>
#> $supplemented$sources$source_Doran$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $supplemented$sources$source_Doran$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Doran$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Doran$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Doran$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Doran$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Killgore07
#> $supplemented$sources$source_Killgore07$id
#> [1] "source_Killgore07"
#>
#> $supplemented$sources$source_Killgore07$label
#> [1] "Killgore et al (2007) The trait of Introversion–Extraversion predicts vulnerability to sleep deprivation."
#>
#> $supplemented$sources$source_Killgore07$xdoi
#> [1] "doi:10.1111/j.1365-2869.2007.00611.x"
#>
#> $supplemented$sources$source_Killgore07$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Killgore07$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Killgore07$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Killgore07$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Killgore07$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_VanDongen
#> $supplemented$sources$source_VanDongen$id
#> [1] "source_VanDongen"
#>
#> $supplemented$sources$source_VanDongen$label
#> [1] "Van Dongen et al (2003) Sleep debt: Theoretical and empirical issues."
#>
#> $supplemented$sources$source_VanDongen$comment
#> [1] "No DOI found yet; located in Sleep and Biological Rhythms, vol 1, pp 5–13"
#>
#> $supplemented$sources$source_VanDongen$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_VanDongen$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_VanDongen$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_VanDongen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_VanDongen$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Kuypers
#> $supplemented$sources$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$sources$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$sources$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$sources$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Williamson
#> $supplemented$sources$source_Williamson$id
#> [1] "source_Williamson"
#>
#> $supplemented$sources$source_Williamson$label
#> [1] "Williamson & Feyer (2000) Moderate sleep deprivation produces impairments in cognitive and motor performance equivalent to legally prescribed levels of alcohol intoxication."
#>
#> $supplemented$sources$source_Williamson$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Williamson$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Williamson$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Williamson$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Williamson$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Peeke
#> $supplemented$sources$source_Peeke$id
#> [1] "source_Peeke"
#>
#> $supplemented$sources$source_Peeke$label
#> [1] "Peeke et al (1980) Combined effects of alcohol and sleep deprivation in normal young adults."
#>
#> $supplemented$sources$source_Peeke$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Peeke$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Peeke$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Peeke$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Croes
#> $supplemented$sources$source_Croes$id
#> [1] "source_Croes"
#>
#> $supplemented$sources$source_Croes$label
#> [1] "Croes et al (2017) Langdurige klachten na ecstasygebruik."
#>
#> $supplemented$sources$source_Croes$xdoi
#> [1] "url:www.trimbos.nl/docs/2c1748e6-93d5-481b-8fad-013f91a9e1df.pdf"
#>
#> $supplemented$sources$source_Croes$type
#> [1] "Report"
#>
#> $supplemented$sources$source_Croes$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Croes$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Croes$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Croes$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Berger
#> $supplemented$sources$source_Berger$id
#> [1] "source_Berger"
#>
#> $supplemented$sources$source_Berger$label
#> [1] "Energy conservation and sleep."
#>
#> $supplemented$sources$source_Berger$xdoi
#> [1] "doi:10.1016/0166-4328(95)00002-b"
#>
#> $supplemented$sources$source_Berger$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Berger$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Berger$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Berger$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Berger$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Fairclough
#> $supplemented$sources$source_Fairclough$id
#> [1] "source_Fairclough"
#>
#> $supplemented$sources$source_Fairclough$label
#> [1] "Fairclough & Graham (1999) Impairment of driving performance caused by sleep deprivation or alcohol: A comparative Study. "
#>
#> $supplemented$sources$source_Fairclough$xdoi
#> [1] "doi:10.1518/001872099779577336"
#>
#> $supplemented$sources$source_Fairclough$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Fairclough$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Fairclough$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Fairclough$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Fairclough$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Krueger
#> $supplemented$sources$source_Krueger$id
#> [1] "source_Krueger"
#>
#> $supplemented$sources$source_Krueger$label
#> [1] "Kreueger et al (2006) The role of cytokines in physiological sleep regulation"
#>
#> $supplemented$sources$source_Krueger$xdoi
#> [1] "doi:10.1111/j.1749-6632.2001.tb05826.x"
#>
#> $supplemented$sources$source_Krueger$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Krueger$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Krueger$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Krueger$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Krueger$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Jessen
#> $supplemented$sources$source_Jessen$id
#> [1] "source_Jessen"
#>
#> $supplemented$sources$source_Jessen$label
#> [1] "Jessen et al (2015) The Glymphatic System: A Beginner’s Guide."
#>
#> $supplemented$sources$source_Jessen$xdoi
#> [1] "doi:10.1007/s11064-015-1581-6"
#>
#> $supplemented$sources$source_Jessen$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Jessen$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Jessen$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Jessen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Jessen$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Lim
#> $supplemented$sources$source_Lim$id
#> [1] "source_Lim"
#>
#> $supplemented$sources$source_Lim$label
#> [1] "Lim & Dinges (2010) A meta-analysis of the impact of short-term sleep deprivation on cognitive variables."
#>
#> $supplemented$sources$source_Lim$xdoi
#> [1] "doi:10.1037/a0018883"
#>
#> $supplemented$sources$source_Lim$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Lim$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Lim$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Lim$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Lim$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Tononi
#> $supplemented$sources$source_Tononi$id
#> [1] "source_Tononi"
#>
#> $supplemented$sources$source_Tononi$label
#> [1] "Tononi & Cirelli (2006) ). Sleep function and synaptic homeostasis."
#>
#> $supplemented$sources$source_Tononi$xdoi
#> [1] "doi:10.1016/j.smrv.2005.05.002"
#>
#> $supplemented$sources$source_Tononi$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Tononi$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Tononi$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Tononi$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Tononi$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Beebe
#> $supplemented$sources$source_Beebe$id
#> [1] "source_Beebe"
#>
#> $supplemented$sources$source_Beebe$label
#> [1] "Beebe et al (2010). Attention, learning, and arousal of experimentally sleep-restricted adolescents in a simulated classroom"
#>
#> $supplemented$sources$source_Beebe$xdoi
#> [1] "doi:10.1016/j.jadohealth.2010.03.005"
#>
#> $supplemented$sources$source_Beebe$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Beebe$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Beebe$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Beebe$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Beebe$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_Harrison
#> $supplemented$sources$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$sources$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$sources$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$sources$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$phd_peters_xtc_determinants
#> $supplemented$sources$phd_peters_xtc_determinants$id
#> [1] "phd_peters_xtc_determinants"
#>
#> $supplemented$sources$phd_peters_xtc_determinants$label
#> [1] "Peters, G. J. Y. (2008) Determinants of ecstasy use and harm reduction strategies: informing evidence-based intervention development. https://phdthesis.nl"
#>
#> $supplemented$sources$phd_peters_xtc_determinants$xdoi
#> [1] "isbn:9781409240716"
#>
#> $supplemented$sources$phd_peters_xtc_determinants$fromFile
#> [1] "none"
#>
#> $supplemented$sources$phd_peters_xtc_determinants$fromDir
#> [1] "."
#>
#> $supplemented$sources$phd_peters_xtc_determinants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$phd_peters_xtc_determinants$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$phd_vervaeke_xtc_social
#> $supplemented$sources$phd_vervaeke_xtc_social$id
#> [1] "phd_vervaeke_xtc_social"
#>
#> $supplemented$sources$phd_vervaeke_xtc_social$label
#> [1] "Vervaeke, H. K. E. (2009) Initiation and continuation: social context and behavioural aspects of ecstasy use"
#>
#> $supplemented$sources$phd_vervaeke_xtc_social$fromFile
#> [1] "none"
#>
#> $supplemented$sources$phd_vervaeke_xtc_social$fromDir
#> [1] "."
#>
#> $supplemented$sources$phd_vervaeke_xtc_social$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$phd_vervaeke_xtc_social$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$`src:phd_peters_determinant_xtc_use`
#> $supplemented$sources$`src:phd_peters_determinant_xtc_use`$id
#> [1] "src:phd_peters_determinant_xtc_use"
#>
#> $supplemented$sources$`src:phd_peters_determinant_xtc_use`$pp
#> [1] 91
#>
#> $supplemented$sources$`src:phd_peters_determinant_xtc_use`$fromFile
#> [1] "none"
#>
#> $supplemented$sources$`src:phd_peters_determinant_xtc_use`$fromDir
#> [1] "."
#>
#> $supplemented$sources$`src:phd_peters_determinant_xtc_use`$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$`src:phd_peters_determinant_xtc_use`$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$`src:phd_vervaeke_xtc_social`
#> $supplemented$sources$`src:phd_vervaeke_xtc_social`$id
#> [1] "src:phd_vervaeke_xtc_social"
#>
#> $supplemented$sources$`src:phd_vervaeke_xtc_social`$pp
#> [1] 36
#>
#> $supplemented$sources$`src:phd_vervaeke_xtc_social`$fromFile
#> [1] "none"
#>
#> $supplemented$sources$`src:phd_vervaeke_xtc_social`$fromDir
#> [1] "."
#>
#> $supplemented$sources$`src:phd_vervaeke_xtc_social`$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$`src:phd_vervaeke_xtc_social`$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_1
#> $supplemented$sources$source_1$id
#> [1] "source_1"
#>
#> $supplemented$sources$source_1$label
#> [1] "Source 1"
#>
#> $supplemented$sources$source_1$xdoi
#> [1] "doi:10.0001/1000-0001.1.0.1"
#>
#> $supplemented$sources$source_1$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_1$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_1$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_2
#> $supplemented$sources$source_2$id
#> [1] "source_2"
#>
#> $supplemented$sources$source_2$label
#> [1] "Source 2"
#>
#> $supplemented$sources$source_2$xdoi
#> [1] "doi:10.0002/2000-0002.2.0.2"
#>
#> $supplemented$sources$source_2$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_2$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_2$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$source_3
#> $supplemented$sources$source_3$id
#> [1] "source_3"
#>
#> $supplemented$sources$source_3$label
#> [1] "Source 3"
#>
#> $supplemented$sources$source_3$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $supplemented$sources$source_3$type
#> [1] "Journal article"
#>
#> $supplemented$sources$source_3$fromFile
#> [1] "none"
#>
#> $supplemented$sources$source_3$fromDir
#> [1] "."
#>
#> $supplemented$sources$source_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$source_3$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$brunt_2012
#> $supplemented$sources$brunt_2012$id
#> [1] "brunt_2012"
#>
#> $supplemented$sources$brunt_2012$label
#> [1] "Brunt, Koeter, Niesink & van den Brink (2012)"
#>
#> $supplemented$sources$brunt_2012$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$sources$brunt_2012$fromFile
#> [1] "none"
#>
#> $supplemented$sources$brunt_2012$fromDir
#> [1] "."
#>
#> $supplemented$sources$brunt_2012$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$brunt_2012$date
#> [1] "2019-03-06"
#>
#> $supplemented$sources$brunt_2012$scores
#> $supplemented$sources$brunt_2012$scores$evidence_type
#> [1] 5
#>
#>
#>
#> $supplemented$sources$project_proposal
#> $supplemented$sources$project_proposal$id
#> [1] "project_proposal"
#>
#> $supplemented$sources$project_proposal$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $supplemented$sources$project_proposal$year
#> [1] 2017
#>
#> $supplemented$sources$project_proposal$fromFile
#> [1] "none"
#>
#> $supplemented$sources$project_proposal$fromDir
#> [1] "."
#>
#> $supplemented$sources$project_proposal$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$project_proposal$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$sources$nice_round_numbers_are_nice
#> $supplemented$sources$nice_round_numbers_are_nice$id
#> [1] "nice_round_numbers_are_nice"
#>
#> $supplemented$sources$nice_round_numbers_are_nice$year
#> [1] 219
#>
#> $supplemented$sources$nice_round_numbers_are_nice$label
#> [1] "In our meeting of 2019-07-05, we agreed that all team members like nice round numbers."
#>
#> $supplemented$sources$nice_round_numbers_are_nice$evidence_type
#> [1] "team opinion"
#>
#> $supplemented$sources$nice_round_numbers_are_nice$fromFile
#> [1] "none"
#>
#> $supplemented$sources$nice_round_numbers_are_nice$fromDir
#> [1] "."
#>
#> $supplemented$sources$nice_round_numbers_are_nice$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$nice_round_numbers_are_nice$date
#> [1] "2019-03-06"
#>
#> $supplemented$sources$nice_round_numbers_are_nice$scores
#> $supplemented$sources$nice_round_numbers_are_nice$scores$evidence_type
#> [1] 1
#>
#>
#>
#> $supplemented$sources$moinester_gottfried_2014
#> $supplemented$sources$moinester_gottfried_2014$id
#> [1] "moinester_gottfried_2014"
#>
#> $supplemented$sources$moinester_gottfried_2014$year
#> [1] 2014
#>
#> $supplemented$sources$moinester_gottfried_2014$label
#> [1] "Moinester, M., & Gottfried, R. (2014). Sample size estimation for correlations with pre-specified confidence interval. The Quantitative Methods of Psychology, 10(2), 124–130."
#>
#> $supplemented$sources$moinester_gottfried_2014$evidence_type
#> [1] "theory"
#>
#> $supplemented$sources$moinester_gottfried_2014$fromFile
#> [1] "none"
#>
#> $supplemented$sources$moinester_gottfried_2014$fromDir
#> [1] "."
#>
#> $supplemented$sources$moinester_gottfried_2014$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$moinester_gottfried_2014$date
#> [1] "2019-03-06"
#>
#> $supplemented$sources$moinester_gottfried_2014$scores
#> $supplemented$sources$moinester_gottfried_2014$scores$evidence_type
#> [1] 4
#>
#>
#>
#> $supplemented$sources$example_source
#> $supplemented$sources$example_source$id
#> [1] "example_source"
#>
#> $supplemented$sources$example_source$label
#> [1] "Not online now, but a study making this point would be nice here"
#>
#> $supplemented$sources$example_source$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$sources$example_source$fromFile
#> [1] "none"
#>
#> $supplemented$sources$example_source$fromDir
#> [1] "."
#>
#> $supplemented$sources$example_source$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$sources$example_source$date
#> [1] "2019-03-06"
#>
#> $supplemented$sources$example_source$scores
#> $supplemented$sources$example_source$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $supplemented$assertions
#> $supplemented$assertions$assertion_sleep_memory_1
#> $supplemented$assertions$assertion_sleep_memory_1$id
#> [1] "assertion_sleep_memory_1"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$source$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_2
#> $supplemented$assertions$assertion_sleep_memory_2$id
#> [1] "assertion_sleep_memory_2"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$id
#> [1] "source_Gais"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$label
#> [1] "Gais (2003) Sleep after learning aids memory recall."
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$source$source_Gais$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_3
#> $supplemented$assertions$assertion_sleep_memory_3$id
#> [1] "assertion_sleep_memory_3"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$label
#> [1] "Sleep promotes the consolidation of fear memory in humans."
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$id
#> [1] "source_Menz"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$label
#> [1] "Menz et al (2013) The role of sleep and sleep deprivation in consolidating fear memories."
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$xdoi
#> [1] "doi:10.1016/j.neuroimage.2013.03.001"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$source$source_Menz$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_3$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_3$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_4
#> $supplemented$assertions$assertion_sleep_memory_4$id
#> [1] "assertion_sleep_memory_4"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$label
#> [1] "Sleep promotes the consolidation of emotional memory in humans."
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$id
#> [1] "source_Holland"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$label
#> [1] "Holland & Lewis (2007) Emotional memory: selective enhancement by sleep."
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$xdoi
#> [1] "doi:10.1016/j.cub.2006.12.033"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$source$source_Holland$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_4$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_4$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_4$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_5
#> $supplemented$assertions$assertion_sleep_memory_5$id
#> [1] "assertion_sleep_memory_5"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$label
#> [1] "Sleep promotes the consolidation of memory in animals after training for a specific task."
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$id
#> [1] "source_Graves"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$label
#> [1] "Graves (2003) Sleep deprivation selectively impairs memory consolidation for contextual fear conditioning."
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$xdoi
#> [1] "doi:10.1101/lm.48803"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$source$source_Graves$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_5$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_5$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_5$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_6
#> $supplemented$assertions$assertion_sleep_memory_6$id
#> [1] "assertion_sleep_memory_6"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$label
#> [1] "Memory retention is already noticable after only several minutes of sleep."
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$source$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_memory_6$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_memory_6$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_memory_6$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_cytokines
#> $supplemented$assertions$assertion_cytokines$id
#> [1] "assertion_cytokines"
#>
#> $supplemented$assertions$assertion_cytokines$label
#> [1] "Infection, stress and tissue damage triggers the release of inflammatory cytokines."
#>
#> $supplemented$assertions$assertion_cytokines$source
#> $supplemented$assertions$assertion_cytokines$source$source_Frey
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$id
#> [1] "source_Frey"
#>
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_cytokines$source$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_cytokines$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_cytokines$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_cytokines$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_cytokines_function
#> $supplemented$assertions$assertion_cytokines_function$id
#> [1] "assertion_cytokines_function"
#>
#> $supplemented$assertions$assertion_cytokines_function$label
#> [1] "When pro-inflammatory cytokines are injected, these cytokines enhance sleep."
#>
#> $supplemented$assertions$assertion_cytokines_function$source
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$id
#> [1] "source_Krueger"
#>
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$label
#> [1] "Kreueger et al (2006) The role of cytokines in physiological sleep regulation"
#>
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$xdoi
#> [1] "doi:10.1111/j.1749-6632.2001.tb05826.x"
#>
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_cytokines_function$source$source_Krueger$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_cytokines_function$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_cytokines_function$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_cytokines_function$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_cytokines_function$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system
#> $supplemented$assertions$assertion_sleep_glympathic_system$id
#> [1] "assertion_sleep_glympathic_system"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$label
#> [1] "Sleep promotes the glympathic system."
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$id
#> [1] "source_Xie"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$label
#> [1] "Zie et al (2013) ). Sleep drives metabolite clearance from the adult brain."
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$xdoi
#> [1] "doi:10.1126/science.1241224"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$source$source_Xie$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_glympathic_system$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_cytokines
#> $supplemented$assertions$assertion_SD_cytokines$id
#> [1] "assertion_SD_cytokines"
#>
#> $supplemented$assertions$assertion_SD_cytokines$label
#> [1] "Sleep deprivation is associated with an increase of pro-inflammatory cytokines, which creates a disbalance of inflammatory cytokines and this induces inflammation."
#>
#> $supplemented$assertions$assertion_SD_cytokines$source
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$id
#> [1] "source_Frey"
#>
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_cytokines$source$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_cytokines$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_cytokines$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_cytokines$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_resting_1
#> $supplemented$assertions$assertion_resting_1$id
#> [1] "assertion_resting_1"
#>
#> $supplemented$assertions$assertion_resting_1$label
#> [1] "Resting enhances the consolidation of memory."
#>
#> $supplemented$assertions$assertion_resting_1$source
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$id
#> [1] "source_Brokaw"
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$label
#> [1] "Brokaw et al (2016) Resting state EEG correlates of memory consolidation."
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$xdoi
#> [1] "doi:10.1016/j.nlm.2016.01.008"
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$pp
#> [1] "17-25"
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_resting_1$source$source_Brokaw$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_resting_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_resting_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_resting_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_resting_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_resting_2
#> $supplemented$assertions$assertion_resting_2$id
#> [1] "assertion_resting_2"
#>
#> $supplemented$assertions$assertion_resting_2$label
#> [1] "The neurotransmitter ACh is released during quiet rest, just like during sleep."
#>
#> $supplemented$assertions$assertion_resting_2$source
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$id
#> [1] "source_Marrosu"
#>
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$label
#> [1] "Marrosu et al (1995) Microdialysis measurement of cortical and hippocampal acetylcholine release during sleep-wake cycle in freely moving cats."
#>
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$xdoi
#> [1] "No DOI found yet, located in: Brain Research, vol 671, pp 329–332."
#>
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_resting_2$source$source_Marrosu$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_resting_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_resting_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_resting_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_resting_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_nocturnal_1
#> $supplemented$assertions$assertion_nocturnal_1$id
#> [1] "assertion_nocturnal_1"
#>
#> $supplemented$assertions$assertion_nocturnal_1$label
#> [1] "Cells like leukocytes show a specific diurnal or nocturnal rhythm."
#>
#> $supplemented$assertions$assertion_nocturnal_1$source
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$id
#> [1] "source_Lange"
#>
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_nocturnal_1$source$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_nocturnal_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_nocturnal_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_nocturnal_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_nocturnal_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_nocturnal_2
#> $supplemented$assertions$assertion_nocturnal_2$id
#> [1] "assertion_nocturnal_2"
#>
#> $supplemented$assertions$assertion_nocturnal_2$label
#> [1] "During nocturnal sleep cell counts of toxic cells are suppressed."
#>
#> $supplemented$assertions$assertion_nocturnal_2$source
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$id
#> [1] "source_Lange"
#>
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_nocturnal_2$source$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_nocturnal_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_nocturnal_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_nocturnal_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_nocturnal_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_sleep_debt
#> $supplemented$assertions$assertion_sleep_debt$id
#> [1] "assertion_sleep_debt"
#>
#> $supplemented$assertions$assertion_sleep_debt$label
#> [1] "Sleep deprivation results in a sleep debt, which has unhealthy consequences."
#>
#> $supplemented$assertions$assertion_sleep_debt$source
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$id
#> [1] "source_Burgess"
#>
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$label
#> [1] "Burgess et al (2002) ). Bright light, dark and melatonin can promote circadian adaptation in night shift workers."
#>
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$xdoi
#> [1] "doi:10.1053/smrv.2001.0215"
#>
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_debt$source$source_Burgess$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_sleep_debt$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_sleep_debt$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_sleep_debt$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_sleep_debt$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_glympathic_system_2
#> $supplemented$assertions$assertion_glympathic_system_2$id
#> [1] "assertion_glympathic_system_2"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$label
#> [1] "Failure of the glympathic system is associated with Alzheimer's disease."
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$id
#> [1] "source_Plog"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$label
#> [1] "Plog & Nedergaard (2018) The glymphatic system in central nervous system health and disease: past, present, and future"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$xdoi
#> [1] "doi:10.1146/annurev-pathol-051217-111018"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$source$source_Plog$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_glympathic_system_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_glympathic_system_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_glympathic_system_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_glympathic_system_1
#> $supplemented$assertions$assertion_glympathic_system_1$id
#> [1] "assertion_glympathic_system_1"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$label
#> [1] "The glympathic system is a recently discovered waste clearance pathway that removes metabolites and toxic proteins from the brain."
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$id
#> [1] "source_Jessen"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$label
#> [1] "Jessen et al (2015) The Glymphatic System: A Beginner’s Guide."
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$xdoi
#> [1] "doi:10.1007/s11064-015-1581-6"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$source$source_Jessen$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_glympathic_system_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_glympathic_system_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_glympathic_system_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_performance
#> $supplemented$assertions$assertion_SD_performance$id
#> [1] "assertion_SD_performance"
#>
#> $supplemented$assertions$assertion_SD_performance$label
#> [1] "(Cognitive) performance deteriorates after 16 hours of wakefulness."
#>
#> $supplemented$assertions$assertion_SD_performance$source
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_performance$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_performance$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_performance$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_performance$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_performance$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_sensory_perception
#> $supplemented$assertions$assertion_SD_sensory_perception$id
#> [1] "assertion_SD_sensory_perception"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$label
#> [1] "Sleep loss causes a reduction in visual cortex activity and the reduction is most prominent when an individual experiences an attentional lapse."
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_sensory_perception$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_inattentive_behaviours
#> $supplemented$assertions$assertion_inattentive_behaviours$id
#> [1] "assertion_inattentive_behaviours"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$label
#> [1] "sleep deprived individuals showed significantly more inattentive behaviours compared to non-sleep deprived individuals while watching a movie."
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$id
#> [1] "source_Beebe"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$label
#> [1] "Beebe et al (2010). Attention, learning, and arousal of experimentally sleep-restricted adolescents in a simulated classroom"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$xdoi
#> [1] "doi:10.1016/j.jadohealth.2010.03.005"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$source$source_Beebe$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_inattentive_behaviours$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_decision
#> $supplemented$assertions$assertion_SD_decision$id
#> [1] "assertion_SD_decision"
#>
#> $supplemented$assertions$assertion_SD_decision$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $supplemented$assertions$assertion_SD_decision$source
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_decision$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_decision$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_decision$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_decision$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_state_instability
#> $supplemented$assertions$assertion_state_instability$id
#> [1] "assertion_state_instability"
#>
#> $supplemented$assertions$assertion_state_instability$label
#> [1] "After sleep deprivation performance becomes instable, which is called state instability."
#>
#> $supplemented$assertions$assertion_state_instability$source
#> $supplemented$assertions$assertion_state_instability$source$source_Doran
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$id
#> [1] "source_Doran"
#>
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$label
#> [1] "Doran et al (2001) Sustained attention performance during sleep deprivation: evidence of state instability."
#>
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_state_instability$source$source_Doran$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_state_instability$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_state_instability$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_state_instability$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_state_instability$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_emotion_1
#> $supplemented$assertions$assertion_SD_emotion_1$id
#> [1] "assertion_SD_emotion_1"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$label
#> [1] "Sleep loss enhances the strenth of reactions to negative, but not to positive or neutral stimuli."
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_emotion_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_emotion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_emotion_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_emotion_2
#> $supplemented$assertions$assertion_SD_emotion_2$id
#> [1] "assertion_SD_emotion_2"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$label
#> [1] "Humor is negatively evaluated and emotional expression is lost in the voice after sleep deprivation."
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_emotion_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_emotion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_emotion_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_individual_differences_1
#> $supplemented$assertions$assertion_individual_differences_1$id
#> [1] "assertion_individual_differences_1"
#>
#> $supplemented$assertions$assertion_individual_differences_1$label
#> [1] "The personality trait extraversion is more related to attentional lapses and more extensive declines in speed response during a task after one night of sleep deprivation."
#>
#> $supplemented$assertions$assertion_individual_differences_1$source
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$id
#> [1] "source_Killgore07"
#>
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$label
#> [1] "Killgore et al (2007) The trait of Introversion–Extraversion predicts vulnerability to sleep deprivation."
#>
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$xdoi
#> [1] "doi:10.1111/j.1365-2869.2007.00611.x"
#>
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_individual_differences_1$source$source_Killgore07$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_individual_differences_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_individual_differences_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_individual_differences_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_individual_differences_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_individual_differences_2
#> $supplemented$assertions$assertion_individual_differences_2$id
#> [1] "assertion_individual_differences_2"
#>
#> $supplemented$assertions$assertion_individual_differences_2$label
#> [1] "There is a genetic predisposition for vulnerability to sleep loss."
#>
#> $supplemented$assertions$assertion_individual_differences_2$source
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$id
#> [1] "source_VanDongen"
#>
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$label
#> [1] "Van Dongen et al (2003) Sleep debt: Theoretical and empirical issues."
#>
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$comment
#> [1] "No DOI found yet; located in Sleep and Biological Rhythms, vol 1, pp 5–13"
#>
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_individual_differences_2$source$source_VanDongen$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_individual_differences_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_individual_differences_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_individual_differences_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_individual_differences_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$id
#> [1] "assertion_MDMA_impulse_daytime"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$label
#> [1] "When MDMA is taken during daytime, impulse control is enhanced."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_daytime$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$id
#> [1] "assertion_MDMA_impulse_nighttime"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$label
#> [1] "When MDMA is taken during nighttime, impulse control is not enhanced."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_impulse_nighttime$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$id
#> [1] "assertion_MDMA_PMF_daytime"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$label
#> [1] "When MDMA is taken during daytime psychomotor functioning is enhanced."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_daytime$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$id
#> [1] "assertion_MDMA_PMF_nighttime"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$label
#> [1] "When MDMA is taken during nighttime psychomotor functioning is impaired."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_PMF_nighttime$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_alcohol
#> $supplemented$assertions$assertion_SD_alcohol$id
#> [1] "assertion_SD_alcohol"
#>
#> $supplemented$assertions$assertion_SD_alcohol$label
#> [1] "Sleep deprivation causes cognitive and motor performance impairment similar to alcohol intoxication."
#>
#> $supplemented$assertions$assertion_SD_alcohol$source
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson$id
#> [1] "source_Williamson"
#>
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson$label
#> [1] "Williamson & Feyer (2000) Moderate sleep deprivation produces impairments in cognitive and motor performance equivalent to legally prescribed levels of alcohol intoxication."
#>
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_alcohol$source$source_Williamson$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_alcohol$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_alcohol$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_alcohol$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_alcohol$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$id
#> [1] "assertion_interaction_SD_alcohol_1"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived react slower than people who are either are under the influence of alcohol or sleep deprived alone."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source$source_Peeke
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source$source_Peeke$id
#> [1] "source_Peeke"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source$source_Peeke$label
#> [1] "Peeke et al (1980) Combined effects of alcohol and sleep deprivation in normal young adults."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source$source_Peeke$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source$source_Peeke$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source$source_Peeke$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$source$source_Peeke$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$id
#> [1] "assertion_interaction_SD_alcohol_2"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived show worse driving performance than people who either are under the influence of alcohol or sleep deprived alone."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$comment
#> [1] "this source was 'Howard'. But the one that was named 'Howard', I renamed to 'Harrison'. Smth wrong here?"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$source$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_interaction_SD_alcohol_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_MDMA_cortisol
#> $supplemented$assertions$assertion_MDMA_cortisol$id
#> [1] "assertion_MDMA_cortisol"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$label
#> [1] "Cortisol levels rise after MDMA is taken."
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$id
#> [1] "source_Croes"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$label
#> [1] "Croes et al (2017) Langdurige klachten na ecstasygebruik."
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$xdoi
#> [1] "url:www.trimbos.nl/docs/2c1748e6-93d5-481b-8fad-013f91a9e1df.pdf"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$type
#> [1] "Report"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$source$source_Croes$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_MDMA_cortisol$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_resting_3
#> $supplemented$assertions$assertion_resting_3$id
#> [1] "assertion_resting_3"
#>
#> $supplemented$assertions$assertion_resting_3$label
#> [1] "Important functions of sleep, like recovery of neural function and synaptic downscaling to save energy and experience benefits for learning and memory, only occur together with slow wave sleep and therefore not while resting."
#>
#> $supplemented$assertions$assertion_resting_3$source
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$id
#> [1] "source_Tononi"
#>
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$label
#> [1] "Tononi & Cirelli (2006) ). Sleep function and synaptic homeostasis."
#>
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$xdoi
#> [1] "doi:10.1016/j.smrv.2005.05.002"
#>
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_resting_3$source$source_Tononi$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_resting_3$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_resting_3$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_resting_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_resting_3$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_attention
#> $supplemented$assertions$assertion_attention$id
#> [1] "assertion_attention"
#>
#> $supplemented$assertions$assertion_attention$label
#> [1] "The most simple form of attention, that is being able to detect a stimulus, is most strongly affected by sleep deprivation."
#>
#> $supplemented$assertions$assertion_attention$source
#> $supplemented$assertions$assertion_attention$source$source_Lim
#> $supplemented$assertions$assertion_attention$source$source_Lim$id
#> [1] "source_Lim"
#>
#> $supplemented$assertions$assertion_attention$source$source_Lim$label
#> [1] "Lim & Dinges (2010) A meta-analysis of the impact of short-term sleep deprivation on cognitive variables."
#>
#> $supplemented$assertions$assertion_attention$source$source_Lim$xdoi
#> [1] "doi:10.1037/a0018883"
#>
#> $supplemented$assertions$assertion_attention$source$source_Lim$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_attention$source$source_Lim$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_attention$source$source_Lim$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_attention$source$source_Lim$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_attention$source$source_Lim$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_attention$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_attention$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_attention$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_attention$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_SD_decision
#> $supplemented$assertions$assertion_SD_decision$id
#> [1] "assertion_SD_decision"
#>
#> $supplemented$assertions$assertion_SD_decision$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $supplemented$assertions$assertion_SD_decision$source
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_decision$source$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_SD_decision$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_SD_decision$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_SD_decision$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_SD_decision$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention
#> $supplemented$assertions$tryout_determinants_ranking_intention$id
#> [1] "tryout_determinants_ranking_intention"
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$label
#> [1] "Intention to try out XTC is an extremely strong predictor of trying out XTC (Cohen's d > 1, OR > 7)"
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$source
#> $supplemented$assertions$tryout_determinants_ranking_intention$source$`src:phd_peters_determinant_xtc_use`
#> $supplemented$assertions$tryout_determinants_ranking_intention$source$`src:phd_peters_determinant_xtc_use`$id
#> [1] "src:phd_peters_determinant_xtc_use"
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$source$`src:phd_peters_determinant_xtc_use`$pp
#> [1] 91
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$source$`src:phd_peters_determinant_xtc_use`$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$source$`src:phd_peters_determinant_xtc_use`$fromDir
#> [1] "."
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$source$`src:phd_peters_determinant_xtc_use`$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$source$`src:phd_peters_determinant_xtc_use`$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$fromDir
#> [1] "."
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$tryout_determinants_ranking_intention$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude
#> $supplemented$assertions$tryout_determinants_ranking_attitude$id
#> [1] "tryout_determinants_ranking_attitude"
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$label
#> [1] "Attitude is the strongest predictor of intention to try out XTC (r = .67)"
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source$`src:phd_peters_determinant_xtc_use`
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source$`src:phd_peters_determinant_xtc_use`$id
#> [1] "src:phd_peters_determinant_xtc_use"
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source$`src:phd_peters_determinant_xtc_use`$pp
#> [1] 91
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source$`src:phd_peters_determinant_xtc_use`$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source$`src:phd_peters_determinant_xtc_use`$fromDir
#> [1] "."
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source$`src:phd_peters_determinant_xtc_use`$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$source$`src:phd_peters_determinant_xtc_use`$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$fromDir
#> [1] "."
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$tryout_determinants_ranking_attitude$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$id
#> [1] "notry_determinants_ranking_othersubstance"
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$label
#> [1] "Already using some other substance is the strongest 'deterrent' of trying out ecstasy"
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source$`src:phd_vervaeke_xtc_social`
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source$`src:phd_vervaeke_xtc_social`$id
#> [1] "src:phd_vervaeke_xtc_social"
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source$`src:phd_vervaeke_xtc_social`$pp
#> [1] 36
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source$`src:phd_vervaeke_xtc_social`$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source$`src:phd_vervaeke_xtc_social`$fromDir
#> [1] "."
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source$`src:phd_vervaeke_xtc_social`$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$source$`src:phd_vervaeke_xtc_social`$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$fromDir
#> [1] "."
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$notry_determinants_ranking_othersubstance$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_1
#> $supplemented$assertions$assertion_1$id
#> [1] "assertion_1"
#>
#> $supplemented$assertions$assertion_1$label
#> [1] "Assertion 1"
#>
#> $supplemented$assertions$assertion_1$description
#> [1] "Description of assertion 1"
#>
#> $supplemented$assertions$assertion_1$source
#> $supplemented$assertions$assertion_1$source$source_1
#> $supplemented$assertions$assertion_1$source$source_1$id
#> [1] "source_1"
#>
#> $supplemented$assertions$assertion_1$source$source_1$label
#> [1] "Source 1"
#>
#> $supplemented$assertions$assertion_1$source$source_1$xdoi
#> [1] "doi:10.0001/1000-0001.1.0.1"
#>
#> $supplemented$assertions$assertion_1$source$source_1$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_1$source$source_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_1$source$source_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_1$source$source_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_1$source$source_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_1$source$source_2
#> $supplemented$assertions$assertion_1$source$source_2$id
#> [1] "source_2"
#>
#> $supplemented$assertions$assertion_1$source$source_2$label
#> [1] "Source 2"
#>
#> $supplemented$assertions$assertion_1$source$source_2$xdoi
#> [1] "doi:10.0002/2000-0002.2.0.2"
#>
#> $supplemented$assertions$assertion_1$source$source_2$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_1$source$source_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_1$source$source_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_1$source$source_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_1$source$source_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_1$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_1$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$assertion_2
#> $supplemented$assertions$assertion_2$id
#> [1] "assertion_2"
#>
#> $supplemented$assertions$assertion_2$label
#> [1] "Assertion 2"
#>
#> $supplemented$assertions$assertion_2$description
#> [1] "Description of assertion 2"
#>
#> $supplemented$assertions$assertion_2$source
#> $supplemented$assertions$assertion_2$source$source_3
#> $supplemented$assertions$assertion_2$source$source_3$id
#> [1] "source_3"
#>
#> $supplemented$assertions$assertion_2$source$source_3$label
#> [1] "Source 3"
#>
#> $supplemented$assertions$assertion_2$source$source_3$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $supplemented$assertions$assertion_2$source$source_3$type
#> [1] "Journal article"
#>
#> $supplemented$assertions$assertion_2$source$source_3$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_2$source$source_3$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_2$source$source_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_2$source$source_3$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$assertion_2$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$assertion_2$fromDir
#> [1] "."
#>
#> $supplemented$assertions$assertion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$assertion_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$some_pills_are_contaminated
#> $supplemented$assertions$some_pills_are_contaminated$id
#> [1] "some_pills_are_contaminated"
#>
#> $supplemented$assertions$some_pills_are_contaminated$source
#> $supplemented$assertions$some_pills_are_contaminated$source$vogels_2009
#> NULL
#>
#>
#> $supplemented$assertions$some_pills_are_contaminated$label
#> [1] "Not all produced XTC pills contain only MDMA as active ingredient."
#>
#> $supplemented$assertions$some_pills_are_contaminated$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$assertions$some_pills_are_contaminated$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$some_pills_are_contaminated$fromDir
#> [1] "."
#>
#> $supplemented$assertions$some_pills_are_contaminated$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$some_pills_are_contaminated$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$dosing_requires_pill_content
#> $supplemented$assertions$dosing_requires_pill_content$id
#> [1] "dosing_requires_pill_content"
#>
#> $supplemented$assertions$dosing_requires_pill_content$label
#> [1] "Determining the dose of MDMA one uses requires knowing both one's body weight and the pill dose."
#>
#> $supplemented$assertions$dosing_requires_pill_content$source
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$id
#> [1] "brunt_2012"
#>
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$label
#> [1] "Brunt, Koeter, Niesink & van den Brink (2012)"
#>
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$fromDir
#> [1] "."
#>
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$date
#> [1] "2019-03-06"
#>
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$scores
#> $supplemented$assertions$dosing_requires_pill_content$source$brunt_2012$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $supplemented$assertions$dosing_requires_pill_content$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$dosing_requires_pill_content$fromDir
#> [1] "."
#>
#> $supplemented$assertions$dosing_requires_pill_content$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$dosing_requires_pill_content$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$testing_xtc_is_reasoned
#> $supplemented$assertions$testing_xtc_is_reasoned$id
#> [1] "testing_xtc_is_reasoned"
#>
#> $supplemented$assertions$testing_xtc_is_reasoned$source
#> $supplemented$assertions$testing_xtc_is_reasoned$source$phd_peters_2008
#> NULL
#>
#>
#> $supplemented$assertions$testing_xtc_is_reasoned$label
#> [1] "Previous research indicates that getting one's ecstasy tested (or not) is largely a reasoned behavior"
#>
#> $supplemented$assertions$testing_xtc_is_reasoned$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$testing_xtc_is_reasoned$fromDir
#> [1] "."
#>
#> $supplemented$assertions$testing_xtc_is_reasoned$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$testing_xtc_is_reasoned$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$there_is_qualitative_research_about_testing
#> $supplemented$assertions$there_is_qualitative_research_about_testing$id
#> [1] "there_is_qualitative_research_about_testing"
#>
#> $supplemented$assertions$there_is_qualitative_research_about_testing$source
#> $supplemented$assertions$there_is_qualitative_research_about_testing$source$phd_peters_2008
#> NULL
#>
#>
#> $supplemented$assertions$there_is_qualitative_research_about_testing$label
#> [1] "There exists qualitative research about why people get their ecstasy tested"
#>
#> $supplemented$assertions$there_is_qualitative_research_about_testing$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$there_is_qualitative_research_about_testing$fromDir
#> [1] "."
#>
#> $supplemented$assertions$there_is_qualitative_research_about_testing$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$there_is_qualitative_research_about_testing$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$project_deadline
#> $supplemented$assertions$project_deadline$id
#> [1] "project_deadline"
#>
#> $supplemented$assertions$project_deadline$label
#> [1] "The deadline for this project is december 2019."
#>
#> $supplemented$assertions$project_deadline$source
#> $supplemented$assertions$project_deadline$source$project_proposal
#> $supplemented$assertions$project_deadline$source$project_proposal$id
#> [1] "project_proposal"
#>
#> $supplemented$assertions$project_deadline$source$project_proposal$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $supplemented$assertions$project_deadline$source$project_proposal$year
#> [1] 2017
#>
#> $supplemented$assertions$project_deadline$source$project_proposal$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$project_deadline$source$project_proposal$fromDir
#> [1] "."
#>
#> $supplemented$assertions$project_deadline$source$project_proposal$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$project_deadline$source$project_proposal$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$assertions$project_deadline$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$project_deadline$fromDir
#> [1] "."
#>
#> $supplemented$assertions$project_deadline$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$project_deadline$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$nice_round_number
#> $supplemented$assertions$nice_round_number$id
#> [1] "nice_round_number"
#>
#> $supplemented$assertions$nice_round_number$label
#> [1] "We want to recruit a nice round number of participants."
#>
#> $supplemented$assertions$nice_round_number$source
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$id
#> [1] "nice_round_numbers_are_nice"
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$year
#> [1] 219
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$label
#> [1] "In our meeting of 2019-07-05, we agreed that all team members like nice round numbers."
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$evidence_type
#> [1] "team opinion"
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$fromDir
#> [1] "."
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$date
#> [1] "2019-03-06"
#>
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$scores
#> $supplemented$assertions$nice_round_number$source$nice_round_numbers_are_nice$scores$evidence_type
#> [1] 1
#>
#>
#>
#>
#> $supplemented$assertions$nice_round_number$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$nice_round_number$fromDir
#> [1] "."
#>
#> $supplemented$assertions$nice_round_number$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$nice_round_number$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$exact_aipe_for_our_study
#> $supplemented$assertions$exact_aipe_for_our_study$id
#> [1] "exact_aipe_for_our_study"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$label
#> [1] "Table 1 shows that 383 participants are sufficient even for correlations as low as .05."
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$id
#> [1] "moinester_gottfried_2014"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$year
#> [1] 2014
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$label
#> [1] "Moinester, M., & Gottfried, R. (2014). Sample size estimation for correlations with pre-specified confidence interval. The Quantitative Methods of Psychology, 10(2), 124–130."
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$evidence_type
#> [1] "theory"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$fromDir
#> [1] "."
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$date
#> [1] "2019-03-06"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$scores
#> $supplemented$assertions$exact_aipe_for_our_study$source$moinester_gottfried_2014$scores$evidence_type
#> [1] 4
#>
#>
#>
#>
#> $supplemented$assertions$exact_aipe_for_our_study$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$fromDir
#> [1] "."
#>
#> $supplemented$assertions$exact_aipe_for_our_study$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$exact_aipe_for_our_study$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$assertions$prereg_to_decrease_bias
#> $supplemented$assertions$prereg_to_decrease_bias$id
#> [1] "prereg_to_decrease_bias"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$label
#> [1] "Preregistration_decreases_bias"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$id
#> [1] "example_source"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$label
#> [1] "Not online now, but a study making this point would be nice here"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$fromDir
#> [1] "."
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$date
#> [1] "2019-03-06"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$scores
#> $supplemented$assertions$prereg_to_decrease_bias$source$example_source$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $supplemented$assertions$prereg_to_decrease_bias$fromFile
#> [1] "none"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$fromDir
#> [1] "."
#>
#> $supplemented$assertions$prereg_to_decrease_bias$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$assertions$prereg_to_decrease_bias$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications
#> $supplemented$justifications$decision1
#> $supplemented$justifications$decision1$id
#> [1] "decision1"
#>
#> $supplemented$justifications$decision1$`project-id`
#> [1] "wim"
#>
#> $supplemented$justifications$decision1$name
#> [1] "last finalization study recruitment"
#>
#> $supplemented$justifications$decision1$actors
#> [1] "Person, First" "Person, Second"
#>
#> $supplemented$justifications$decision1$description
#> [1] "We decided to use snowball sampling."
#>
#> $supplemented$justifications$decision1$alternatives
#> [1] "only invite people we know" "cast a wide net"
#>
#> $supplemented$justifications$decision1$choice
#> [1] 2
#>
#> $supplemented$justifications$decision1$justification
#> $supplemented$justifications$decision1$justification$`more inclusive`
#> NULL
#>
#>
#> $supplemented$justifications$decision1$specifications
#> NULL
#>
#> $supplemented$justifications$decision1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$decision1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$decision1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$decision1$date
#> $supplemented$justifications$decision1$date[[1]]
#> [1] "2018-09-12"
#>
#> $supplemented$justifications$decision1$date[[2]]
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$decision2
#> $supplemented$justifications$decision2$id
#> [1] "decision2"
#>
#> $supplemented$justifications$decision2$`project-id`
#> [1] "wim"
#>
#> $supplemented$justifications$decision2$name
#> [1] "do list finalization study"
#>
#> $supplemented$justifications$decision2$actors
#> [1] "Kok, Gerjo" "Peters, Louk" "Crutzen, Rik"
#> [4] "Peters, Gjalt-Jorn"
#>
#> $supplemented$justifications$decision2$description
#> [1] "We decided to blablabla"
#>
#> $supplemented$justifications$decision2$alternatives
#> [1] "no" "yes"
#>
#> $supplemented$justifications$decision2$choice
#> [1] 2
#>
#> $supplemented$justifications$decision2$justification
#> $supplemented$justifications$decision2$justification$`more thorough`
#> NULL
#>
#>
#> $supplemented$justifications$decision2$specifications
#> NULL
#>
#> $supplemented$justifications$decision2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$decision2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$decision2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$decision2$date
#> $supplemented$justifications$decision2$date[[1]]
#> [1] "2018-09-12"
#>
#> $supplemented$justifications$decision2$date[[2]]
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_01
#> $supplemented$justifications$justification_01$id
#> [1] "justification_01"
#>
#> $supplemented$justifications$justification_01$label
#> [1] "Sleep prevents us from getting sick and (therefore) sleep deprivation causes an impaired immune system. Sleep prevents a disbalance of inflammatory cytokines and therefore health is maintained."
#>
#> $supplemented$justifications$justification_01$assertion
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$id
#> [1] "assertion_cytokines"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$label
#> [1] "Infection, stress and tissue damage triggers the release of inflammatory cytokines."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$id
#> [1] "source_Frey"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$source$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$id
#> [1] "assertion_cytokines_function"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$label
#> [1] "When pro-inflammatory cytokines are injected, these cytokines enhance sleep."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$id
#> [1] "source_Krueger"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$label
#> [1] "Kreueger et al (2006) The role of cytokines in physiological sleep regulation"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$xdoi
#> [1] "doi:10.1111/j.1749-6632.2001.tb05826.x"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_cytokines_function$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$id
#> [1] "assertion_SD_cytokines"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$label
#> [1] "Sleep deprivation is associated with an increase of pro-inflammatory cytokines, which creates a disbalance of inflammatory cytokines and this induces inflammation."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$id
#> [1] "source_Frey"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_01$assertion$assertion_SD_cytokines$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_01$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_01$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_01$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_01$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_02
#> $supplemented$justifications$justification_02$id
#> [1] "justification_02"
#>
#> $supplemented$justifications$justification_02$label
#> [1] "Sleep is important for our memory and (therefore) sleep deprivation causes memory impairment."
#>
#> $supplemented$justifications$justification_02$assertion
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$id
#> [1] "assertion_sleep_memory_1"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$id
#> [1] "assertion_sleep_memory_2"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$id
#> [1] "source_Gais"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$label
#> [1] "Gais (2003) Sleep after learning aids memory recall."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$id
#> [1] "assertion_sleep_memory_3"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$label
#> [1] "Sleep promotes the consolidation of fear memory in humans."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$id
#> [1] "source_Menz"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$label
#> [1] "Menz et al (2013) The role of sleep and sleep deprivation in consolidating fear memories."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$xdoi
#> [1] "doi:10.1016/j.neuroimage.2013.03.001"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_3$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$id
#> [1] "assertion_sleep_memory_4"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$label
#> [1] "Sleep promotes the consolidation of emotional memory in humans."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$id
#> [1] "source_Holland"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$label
#> [1] "Holland & Lewis (2007) Emotional memory: selective enhancement by sleep."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$xdoi
#> [1] "doi:10.1016/j.cub.2006.12.033"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_4$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$id
#> [1] "assertion_sleep_memory_5"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$label
#> [1] "Sleep promotes the consolidation of memory in animals after training for a specific task."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$id
#> [1] "source_Graves"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$label
#> [1] "Graves (2003) Sleep deprivation selectively impairs memory consolidation for contextual fear conditioning."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$xdoi
#> [1] "doi:10.1101/lm.48803"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_5$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$id
#> [1] "assertion_sleep_memory_6"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$label
#> [1] "Memory retention is already noticable after only several minutes of sleep."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$assertion$assertion_sleep_memory_6$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_02$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_02$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_02$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_02$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_03
#> $supplemented$justifications$justification_03$id
#> [1] "justification_03"
#>
#> $supplemented$justifications$justification_03$label
#> [1] "Sleep protects us from toxicants and (therefore) sleep deprivation is associated with neurological diseases."
#>
#> $supplemented$justifications$justification_03$assertion
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$id
#> [1] "assertion_glympathic_system_1"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$label
#> [1] "The glympathic system is a recently discovered waste clearance pathway that removes metabolites and toxic proteins from the brain."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$id
#> [1] "source_Jessen"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$label
#> [1] "Jessen et al (2015) The Glymphatic System: A Beginner’s Guide."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$xdoi
#> [1] "doi:10.1007/s11064-015-1581-6"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$source$source_Jessen$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$id
#> [1] "assertion_glympathic_system_2"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$label
#> [1] "Failure of the glympathic system is associated with Alzheimer's disease."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$id
#> [1] "source_Plog"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$label
#> [1] "Plog & Nedergaard (2018) The glymphatic system in central nervous system health and disease: past, present, and future"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$xdoi
#> [1] "doi:10.1146/annurev-pathol-051217-111018"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$source$source_Plog$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_glympathic_system_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$id
#> [1] "assertion_sleep_glympathic_system"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$label
#> [1] "Sleep promotes the glympathic system."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$id
#> [1] "source_Xie"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$label
#> [1] "Zie et al (2013) ). Sleep drives metabolite clearance from the adult brain."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$xdoi
#> [1] "doi:10.1126/science.1241224"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$source$source_Xie$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_03$assertion$assertion_sleep_glympathic_system$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_03$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_03$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_03$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_03$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_04
#> $supplemented$justifications$justification_04$id
#> [1] "justification_04"
#>
#> $supplemented$justifications$justification_04$label
#> [1] "Resting is not as beneficial as sleep, but is preferred over total sleep deprivation as resting state resembles a sleep state."
#>
#> $supplemented$justifications$justification_04$assertion
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$id
#> [1] "assertion_resting_1"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$label
#> [1] "Resting enhances the consolidation of memory."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$id
#> [1] "source_Brokaw"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$label
#> [1] "Brokaw et al (2016) Resting state EEG correlates of memory consolidation."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$xdoi
#> [1] "doi:10.1016/j.nlm.2016.01.008"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$pp
#> [1] "17-25"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$source$source_Brokaw$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$id
#> [1] "assertion_resting_2"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$label
#> [1] "The neurotransmitter ACh is released during quiet rest, just like during sleep."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$id
#> [1] "source_Marrosu"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$label
#> [1] "Marrosu et al (1995) Microdialysis measurement of cortical and hippocampal acetylcholine release during sleep-wake cycle in freely moving cats."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$xdoi
#> [1] "No DOI found yet, located in: Brain Research, vol 671, pp 329–332."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$source$source_Marrosu$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$id
#> [1] "assertion_resting_3"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$label
#> [1] "Important functions of sleep, like recovery of neural function and synaptic downscaling to save energy and experience benefits for learning and memory, only occur together with slow wave sleep and therefore not while resting."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$id
#> [1] "source_Tononi"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$label
#> [1] "Tononi & Cirelli (2006) ). Sleep function and synaptic homeostasis."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$xdoi
#> [1] "doi:10.1016/j.smrv.2005.05.002"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$source$source_Tononi$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_04$assertion$assertion_resting_3$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_04$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_04$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_04$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_04$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_05
#> $supplemented$justifications$justification_05$id
#> [1] "justification_05"
#>
#> $supplemented$justifications$justification_05$label
#> [1] "Nocturnal sleep is preferred over diurnal sleep, but diurnal sleep is preferred over total sleep deprivation."
#>
#> $supplemented$justifications$justification_05$assertion
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$id
#> [1] "assertion_nocturnal_1"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$label
#> [1] "Cells like leukocytes show a specific diurnal or nocturnal rhythm."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$id
#> [1] "source_Lange"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$id
#> [1] "assertion_nocturnal_2"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$label
#> [1] "During nocturnal sleep cell counts of toxic cells are suppressed."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$id
#> [1] "source_Lange"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_nocturnal_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$id
#> [1] "assertion_sleep_debt"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$label
#> [1] "Sleep deprivation results in a sleep debt, which has unhealthy consequences."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$id
#> [1] "source_Burgess"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$label
#> [1] "Burgess et al (2002) ). Bright light, dark and melatonin can promote circadian adaptation in night shift workers."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$xdoi
#> [1] "doi:10.1053/smrv.2001.0215"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_05$assertion$assertion_sleep_debt$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_05$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_05$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_05$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_05$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_06
#> $supplemented$justifications$justification_06$id
#> [1] "justification_06"
#>
#> $supplemented$justifications$justification_06$label
#> [1] "Sleep deprivation causes impaired cognitive performance."
#>
#> $supplemented$justifications$justification_06$assertion
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$id
#> [1] "assertion_SD_performance"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$label
#> [1] "(Cognitive) performance deteriorates after 16 hours of wakefulness."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_SD_performance$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention
#> $supplemented$justifications$justification_06$assertion$assertion_attention$id
#> [1] "assertion_attention"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$label
#> [1] "The most simple form of attention, that is being able to detect a stimulus, is most strongly affected by sleep deprivation."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$id
#> [1] "source_Lim"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$label
#> [1] "Lim & Dinges (2010) A meta-analysis of the impact of short-term sleep deprivation on cognitive variables."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$xdoi
#> [1] "doi:10.1037/a0018883"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$source$source_Lim$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_attention$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$id
#> [1] "assertion_inattentive_behaviours"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$label
#> [1] "sleep deprived individuals showed significantly more inattentive behaviours compared to non-sleep deprived individuals while watching a movie."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$id
#> [1] "source_Beebe"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$label
#> [1] "Beebe et al (2010). Attention, learning, and arousal of experimentally sleep-restricted adolescents in a simulated classroom"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$xdoi
#> [1] "doi:10.1016/j.jadohealth.2010.03.005"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$source$source_Beebe$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_06$assertion$assertion_inattentive_behaviours$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_06$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_06$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_06$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_06$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_07
#> $supplemented$justifications$justification_07$id
#> [1] "justification_07"
#>
#> $supplemented$justifications$justification_07$label
#> [1] "Sleep deprivation causes impaired decision making."
#>
#> $supplemented$justifications$justification_07$assertion
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$id
#> [1] "assertion_SD_decision"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$source$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_07$assertion$assertion_SD_decision$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_07$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_07$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_07$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_07$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_08
#> $supplemented$justifications$justification_08$id
#> [1] "justification_08"
#>
#> $supplemented$justifications$justification_08$label
#> [1] "Sleep deprivation causes state instability."
#>
#> $supplemented$justifications$justification_08$assertion
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$id
#> [1] "assertion_state_instability"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$label
#> [1] "After sleep deprivation performance becomes instable, which is called state instability."
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$id
#> [1] "source_Doran"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$label
#> [1] "Doran et al (2001) Sustained attention performance during sleep deprivation: evidence of state instability."
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$source$source_Doran$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_08$assertion$assertion_state_instability$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_08$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_08$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_08$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_08$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_09
#> $supplemented$justifications$justification_09$id
#> [1] "justification_09"
#>
#> $supplemented$justifications$justification_09$label
#> [1] "Sleep deprivation causes impaired sensory perception."
#>
#> $supplemented$justifications$justification_09$assertion
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$id
#> [1] "assertion_SD_sensory_perception"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$label
#> [1] "Sleep loss causes a reduction in visual cortex activity and the reduction is most prominent when an individual experiences an attentional lapse."
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_09$assertion$assertion_SD_sensory_perception$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_09$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_09$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_09$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_09$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_10
#> $supplemented$justifications$justification_10$id
#> [1] "justification_10"
#>
#> $supplemented$justifications$justification_10$label
#> [1] "Sleep deprivation affects emotional perception and experience."
#>
#> $supplemented$justifications$justification_10$assertion
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$id
#> [1] "assertion_SD_emotion_1"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$label
#> [1] "Sleep loss enhances the strenth of reactions to negative, but not to positive or neutral stimuli."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$id
#> [1] "assertion_SD_emotion_2"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$label
#> [1] "Humor is negatively evaluated and emotional expression is lost in the voice after sleep deprivation."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_10$assertion$assertion_SD_emotion_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_10$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_10$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_10$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_10$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_11
#> $supplemented$justifications$justification_11$id
#> [1] "justification_11"
#>
#> $supplemented$justifications$justification_11$label
#> [1] "There are individual differences in the effect sleep deprivation has on people."
#>
#> $supplemented$justifications$justification_11$assertion
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$id
#> [1] "assertion_individual_differences_1"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$label
#> [1] "The personality trait extraversion is more related to attentional lapses and more extensive declines in speed response during a task after one night of sleep deprivation."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$id
#> [1] "source_Killgore07"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$label
#> [1] "Killgore et al (2007) The trait of Introversion–Extraversion predicts vulnerability to sleep deprivation."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$xdoi
#> [1] "doi:10.1111/j.1365-2869.2007.00611.x"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$source$source_Killgore07$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$id
#> [1] "assertion_individual_differences_2"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$label
#> [1] "There is a genetic predisposition for vulnerability to sleep loss."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$id
#> [1] "source_VanDongen"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$label
#> [1] "Van Dongen et al (2003) Sleep debt: Theoretical and empirical issues."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$comment
#> [1] "No DOI found yet; located in Sleep and Biological Rhythms, vol 1, pp 5–13"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$source$source_VanDongen$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_11$assertion$assertion_individual_differences_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_11$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_11$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_11$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_11$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_12
#> $supplemented$justifications$justification_12$id
#> [1] "justification_12"
#>
#> $supplemented$justifications$justification_12$label
#> [1] "There is an interaction between sleep deprivation and MDMA."
#>
#> $supplemented$justifications$justification_12$assertion
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$id
#> [1] "assertion_MDMA_PMF_nighttime"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$label
#> [1] "When MDMA is taken during nighttime psychomotor functioning is impaired."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_nighttime$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$id
#> [1] "assertion_MDMA_PMF_daytime"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$label
#> [1] "When MDMA is taken during daytime psychomotor functioning is enhanced."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_PMF_daytime$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$id
#> [1] "assertion_MDMA_impulse_nighttime"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$label
#> [1] "When MDMA is taken during nighttime, impulse control is not enhanced."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_nighttime$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$id
#> [1] "assertion_MDMA_impulse_daytime"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$label
#> [1] "When MDMA is taken during daytime, impulse control is enhanced."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$id
#> [1] "source_Kuypers"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$label
#> [1] "Kuypers (2007) psychedelic bliss: memory and risk taking during MDMA intoxication."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$xdoi
#> [1] "ISBN:978-90-9021656-0"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$source$source_Kuypers$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$assertion$assertion_MDMA_impulse_daytime$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_12$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_12$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_12$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_12$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_13
#> $supplemented$justifications$justification_13$id
#> [1] "justification_13"
#>
#> $supplemented$justifications$justification_13$label
#> [1] "There is an interaction between sleep deprivation and alcohol."
#>
#> $supplemented$justifications$justification_13$assertion
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$id
#> [1] "assertion_SD_alcohol"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$label
#> [1] "Sleep deprivation causes cognitive and motor performance impairment similar to alcohol intoxication."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson$id
#> [1] "source_Williamson"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson$label
#> [1] "Williamson & Feyer (2000) Moderate sleep deprivation produces impairments in cognitive and motor performance equivalent to legally prescribed levels of alcohol intoxication."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$source$source_Williamson$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_SD_alcohol$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$id
#> [1] "assertion_interaction_SD_alcohol_1"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived react slower than people who are either are under the influence of alcohol or sleep deprived alone."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source$source_Peeke
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source$source_Peeke$id
#> [1] "source_Peeke"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source$source_Peeke$label
#> [1] "Peeke et al (1980) Combined effects of alcohol and sleep deprivation in normal young adults."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source$source_Peeke$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source$source_Peeke$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source$source_Peeke$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$source$source_Peeke$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$id
#> [1] "assertion_interaction_SD_alcohol_2"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$label
#> [1] "People who are under the influence of alcohol and who are sleep deprived show worse driving performance than people who either are under the influence of alcohol or sleep deprived alone."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$comment
#> [1] "this source was 'Howard'. But the one that was named 'Howard', I renamed to 'Harrison'. Smth wrong here?"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$source$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_13$assertion$assertion_interaction_SD_alcohol_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_13$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_13$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_13$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_1
#> $supplemented$justifications$justification_1$id
#> [1] "justification_1"
#>
#> $supplemented$justifications$justification_1$label
#> [1] "Justification 1"
#>
#> $supplemented$justifications$justification_1$description
#> [1] "Description of justification 1"
#>
#> $supplemented$justifications$justification_1$assertion
#> $supplemented$justifications$justification_1$assertion$assertion_1
#> $supplemented$justifications$justification_1$assertion$assertion_1$id
#> [1] "assertion_1"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$label
#> [1] "Assertion 1"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$description
#> [1] "Description of assertion 1"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$id
#> [1] "source_1"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$label
#> [1] "Source 1"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$xdoi
#> [1] "doi:10.0001/1000-0001.1.0.1"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$id
#> [1] "source_2"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$label
#> [1] "Source 2"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$xdoi
#> [1] "doi:10.0002/2000-0002.2.0.2"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$source$source_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2
#> $supplemented$justifications$justification_1$assertion$assertion_2$id
#> [1] "assertion_2"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$label
#> [1] "Assertion 2"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$description
#> [1] "Description of assertion 2"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$id
#> [1] "source_3"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$label
#> [1] "Source 3"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$source$source_3$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1$assertion$assertion_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_1$justification
#> $supplemented$justifications$justification_1$justification$justification_1b
#> $supplemented$justifications$justification_1$justification$justification_1b$id
#> [1] "justification_1b"
#>
#> $supplemented$justifications$justification_1$justification$justification_1b$label
#> [1] "Justification 1b"
#>
#> $supplemented$justifications$justification_1$justification$justification_1b$description
#> [1] "Description of justification 1b"
#>
#> $supplemented$justifications$justification_1$justification$justification_1b$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1$justification$justification_1b$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1$justification$justification_1b$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1$justification$justification_1b$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_1$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_2
#> $supplemented$justifications$justification_2$id
#> [1] "justification_2"
#>
#> $supplemented$justifications$justification_2$label
#> [1] "Justification 2"
#>
#> $supplemented$justifications$justification_2$description
#> [1] "Description of justification 2"
#>
#> $supplemented$justifications$justification_2$assertion
#> $supplemented$justifications$justification_2$assertion$assertion_2
#> $supplemented$justifications$justification_2$assertion$assertion_2$id
#> [1] "assertion_2"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$label
#> [1] "Assertion 2"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$description
#> [1] "Description of assertion 2"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$id
#> [1] "source_3"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$label
#> [1] "Source 3"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$type
#> [1] "Journal article"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$source$source_3$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_2$assertion$assertion_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$justification_2$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_2$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$id
#> [1] "without_testing_users_may_ingest_contaminants"
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$label
#> [1] "Some XTC pills are contaminated, and one needs to test them to be aware of the pill contents."
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$id
#> [1] "some_pills_are_contaminated"
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$source
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$source$vogels_2009
#> NULL
#>
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$label
#> [1] "Not all produced XTC pills contain only MDMA as active ingredient."
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$fromDir
#> [1] "."
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$fromDir
#> [1] "."
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$without_testing_users_may_ingest_contaminants$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$id
#> [1] "proper_dosing_requires_knowing_dose"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$label
#> [1] "Proper dosing (~ 1-1.5 mg of MDMA per kg of body weight) becomes hard if the dose in a pill is unknown."
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$id
#> [1] "dosing_requires_pill_content"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$label
#> [1] "Determining the dose of MDMA one uses requires knowing both one's body weight and the pill dose."
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$id
#> [1] "brunt_2012"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$label
#> [1] "Brunt, Koeter, Niesink & van den Brink (2012)"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$fromDir
#> [1] "."
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$date
#> [1] "2019-03-06"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$scores
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$fromDir
#> [1] "."
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$fromDir
#> [1] "."
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$proper_dosing_requires_knowing_dose$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$enough_known_about_testing_determinants
#> $supplemented$justifications$enough_known_about_testing_determinants$id
#> [1] "enough_known_about_testing_determinants"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$label
#> [1] "We have enough information available to develop a questionnaire that is likely to measure the most important determinants and sub-determinants of XTC testing."
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$id
#> [1] "testing_xtc_is_reasoned"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$source
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$source$phd_peters_2008
#> NULL
#>
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$label
#> [1] "Previous research indicates that getting one's ecstasy tested (or not) is largely a reasoned behavior"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$fromDir
#> [1] "."
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$id
#> [1] "there_is_qualitative_research_about_testing"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$source
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$source$phd_peters_2008
#> NULL
#>
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$label
#> [1] "There exists qualitative research about why people get their ecstasy tested"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$fromDir
#> [1] "."
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$fromDir
#> [1] "."
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$enough_known_about_testing_determinants$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$limited_time
#> $supplemented$justifications$limited_time$id
#> [1] "limited_time"
#>
#> $supplemented$justifications$limited_time$label
#> [1] "We have to finish this study before the end of 2019."
#>
#> $supplemented$justifications$limited_time$assertion
#> $supplemented$justifications$limited_time$assertion$project_deadline
#> $supplemented$justifications$limited_time$assertion$project_deadline$id
#> [1] "project_deadline"
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$label
#> [1] "The deadline for this project is december 2019."
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$source
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal$id
#> [1] "project_proposal"
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal$year
#> [1] 2017
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal$fromDir
#> [1] "."
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$source$project_proposal$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$fromDir
#> [1] "."
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$limited_time$assertion$project_deadline$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$limited_time$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$limited_time$fromDir
#> [1] "."
#>
#> $supplemented$justifications$limited_time$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$limited_time$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$aipe_for_correlations
#> $supplemented$justifications$aipe_for_correlations$id
#> [1] "aipe_for_correlations"
#>
#> $supplemented$justifications$aipe_for_correlations$label
#> [1] "To estimate a correlation accurately, you need ~ 400 participants."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$id
#> [1] "nice_round_number"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$label
#> [1] "We want to recruit a nice round number of participants."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$id
#> [1] "nice_round_numbers_are_nice"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$year
#> [1] 219
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$label
#> [1] "In our meeting of 2019-07-05, we agreed that all team members like nice round numbers."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$evidence_type
#> [1] "team opinion"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$fromDir
#> [1] "."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$date
#> [1] "2019-03-06"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$scores
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$scores$evidence_type
#> [1] 1
#>
#>
#>
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$fromDir
#> [1] "."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$nice_round_number$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$id
#> [1] "exact_aipe_for_our_study"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$label
#> [1] "Table 1 shows that 383 participants are sufficient even for correlations as low as .05."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$id
#> [1] "moinester_gottfried_2014"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$year
#> [1] 2014
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$label
#> [1] "Moinester, M., & Gottfried, R. (2014). Sample size estimation for correlations with pre-specified confidence interval. The Quantitative Methods of Psychology, 10(2), 124–130."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$evidence_type
#> [1] "theory"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$fromDir
#> [1] "."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$date
#> [1] "2019-03-06"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$scores
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$scores$evidence_type
#> [1] 4
#>
#>
#>
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$fromDir
#> [1] "."
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$aipe_for_correlations$assertion$exact_aipe_for_our_study$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$aipe_for_correlations$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$aipe_for_correlations$fromDir
#> [1] "."
#>
#> $supplemented$justifications$aipe_for_correlations$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$aipe_for_correlations$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$low_risk_of_bias
#> $supplemented$justifications$low_risk_of_bias$id
#> [1] "low_risk_of_bias"
#>
#> $supplemented$justifications$low_risk_of_bias$label
#> [1] "Because we do not test a specific hypothesis but simply want to know how relevant the different determinants are in this population, the need to preregister is less pressing."
#>
#> $supplemented$justifications$low_risk_of_bias$assertion
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$id
#> [1] "prereg_to_decrease_bias"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$label
#> [1] "Preregistration_decreases_bias"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$id
#> [1] "example_source"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$label
#> [1] "Not online now, but a study making this point would be nice here"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$fromDir
#> [1] "."
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$date
#> [1] "2019-03-06"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$scores
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$fromDir
#> [1] "."
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$low_risk_of_bias$assertion$prereg_to_decrease_bias$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$low_risk_of_bias$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$low_risk_of_bias$fromDir
#> [1] "."
#>
#> $supplemented$justifications$low_risk_of_bias$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$low_risk_of_bias$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$no_time_for_prereg
#> $supplemented$justifications$no_time_for_prereg$id
#> [1] "no_time_for_prereg"
#>
#> $supplemented$justifications$no_time_for_prereg$label
#> [1] "We have no time to complete a preregistration form."
#>
#> $supplemented$justifications$no_time_for_prereg$assertion
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$id
#> [1] "project_deadline"
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$label
#> [1] "The deadline for this project is december 2019."
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal$id
#> [1] "project_proposal"
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal$year
#> [1] 2017
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal$fromDir
#> [1] "."
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$source$project_proposal$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$fromDir
#> [1] "."
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$no_time_for_prereg$assertion$project_deadline$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifications$no_time_for_prereg$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$no_time_for_prereg$fromDir
#> [1] "."
#>
#> $supplemented$justifications$no_time_for_prereg$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$no_time_for_prereg$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$justifications$justification_1b
#> $supplemented$justifications$justification_1b$id
#> [1] "justification_1b"
#>
#> $supplemented$justifications$justification_1b$label
#> [1] "Justification 1b"
#>
#> $supplemented$justifications$justification_1b$description
#> [1] "Description of justification 1b"
#>
#> $supplemented$justifications$justification_1b$fromFile
#> [1] "none"
#>
#> $supplemented$justifications$justification_1b$fromDir
#> [1] "."
#>
#> $supplemented$justifications$justification_1b$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$justifications$justification_1b$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions
#> $supplemented$decisions$decision_1
#> $supplemented$decisions$decision_1$id
#> [1] "decision_1"
#>
#> $supplemented$decisions$decision_1$label
#> [1] "Decision 1"
#>
#> $supplemented$decisions$decision_1$description
#> [1] "Description of decision 1"
#>
#> $supplemented$decisions$decision_1$justification
#> $supplemented$decisions$decision_1$justification$justification_1
#> $supplemented$decisions$decision_1$justification$justification_1$id
#> [1] "justification_1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$label
#> [1] "Justification 1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$description
#> [1] "Description of justification 1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$id
#> [1] "assertion_1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$label
#> [1] "Assertion 1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$description
#> [1] "Description of assertion 1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$id
#> [1] "source_1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$label
#> [1] "Source 1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$xdoi
#> [1] "doi:10.0001/1000-0001.1.0.1"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$id
#> [1] "source_2"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$label
#> [1] "Source 2"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$xdoi
#> [1] "doi:10.0002/2000-0002.2.0.2"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$source$source_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$id
#> [1] "assertion_2"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$label
#> [1] "Assertion 2"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$description
#> [1] "Description of assertion 2"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$id
#> [1] "source_3"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$label
#> [1] "Source 3"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$source$source_3$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$assertion$assertion_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_1$justification
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b$id
#> [1] "justification_1b"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b$label
#> [1] "Justification 1b"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b$description
#> [1] "Description of justification 1b"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$justification$justification_1b$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_2
#> $supplemented$decisions$decision_1$justification$justification_2$id
#> [1] "justification_2"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$label
#> [1] "Justification 2"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$description
#> [1] "Description of justification 2"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$id
#> [1] "assertion_2"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$label
#> [1] "Assertion 2"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$description
#> [1] "Description of assertion 2"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$id
#> [1] "source_3"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$label
#> [1] "Source 3"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$xdoi
#> [1] "doi:10.0003/3000-0003.3.0.3"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$source$source_3$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$assertion$assertion_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_1$justification$justification_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$justification$justification_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$justification$justification_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_2
#> $supplemented$decisions$decision_2$id
#> [1] "decision_2"
#>
#> $supplemented$decisions$decision_2$label
#> [1] "Decision 2"
#>
#> $supplemented$decisions$decision_2$description
#> [1] "Description of decision 2"
#>
#> $supplemented$decisions$decision_2$alternatives
#> $supplemented$decisions$decision_2$alternatives[[1]]
#> $supplemented$decisions$decision_2$alternatives[[1]]$value
#> [1] 1
#>
#> $supplemented$decisions$decision_2$alternatives[[1]]$label
#> [1] "Alternative 1"
#>
#> $supplemented$decisions$decision_2$alternatives[[1]]$description
#> [1] "Description of alternative 1"
#>
#>
#> $supplemented$decisions$decision_2$alternatives[[2]]
#> $supplemented$decisions$decision_2$alternatives[[2]]$value
#> [1] 2
#>
#> $supplemented$decisions$decision_2$alternatives[[2]]$label
#> [1] "Alternative 2"
#>
#> $supplemented$decisions$decision_2$alternatives[[2]]$description
#> [1] "Description of alternative 2"
#>
#>
#>
#> $supplemented$decisions$decision_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1
#> $supplemented$decisions$decision_to_select_behavior_1$id
#> [1] "decision_to_select_behavior_1"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$label
#> [1] "People should do so and so because of justifications 04,05,07,10 etc."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$id
#> [1] "justification_04"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$label
#> [1] "Resting is not as beneficial as sleep, but is preferred over total sleep deprivation as resting state resembles a sleep state."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$id
#> [1] "assertion_resting_1"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$label
#> [1] "Resting enhances the consolidation of memory."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$id
#> [1] "source_Brokaw"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$label
#> [1] "Brokaw et al (2016) Resting state EEG correlates of memory consolidation."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$xdoi
#> [1] "doi:10.1016/j.nlm.2016.01.008"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$pp
#> [1] "17-25"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$source$source_Brokaw$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$id
#> [1] "assertion_resting_2"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$label
#> [1] "The neurotransmitter ACh is released during quiet rest, just like during sleep."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$id
#> [1] "source_Marrosu"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$label
#> [1] "Marrosu et al (1995) Microdialysis measurement of cortical and hippocampal acetylcholine release during sleep-wake cycle in freely moving cats."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$xdoi
#> [1] "No DOI found yet, located in: Brain Research, vol 671, pp 329–332."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$source$source_Marrosu$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$id
#> [1] "assertion_resting_3"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$label
#> [1] "Important functions of sleep, like recovery of neural function and synaptic downscaling to save energy and experience benefits for learning and memory, only occur together with slow wave sleep and therefore not while resting."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$id
#> [1] "source_Tononi"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$label
#> [1] "Tononi & Cirelli (2006) ). Sleep function and synaptic homeostasis."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$xdoi
#> [1] "doi:10.1016/j.smrv.2005.05.002"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$source$source_Tononi$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$assertion$assertion_resting_3$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_04$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$id
#> [1] "justification_05"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$label
#> [1] "Nocturnal sleep is preferred over diurnal sleep, but diurnal sleep is preferred over total sleep deprivation."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$id
#> [1] "assertion_nocturnal_1"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$label
#> [1] "Cells like leukocytes show a specific diurnal or nocturnal rhythm."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$id
#> [1] "source_Lange"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$source$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$id
#> [1] "assertion_nocturnal_2"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$label
#> [1] "During nocturnal sleep cell counts of toxic cells are suppressed."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$id
#> [1] "source_Lange"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$label
#> [1] "Lange et al (2010) Effects of sleep and circadian rhythm on the human immune system."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$xdoi
#> [1] "doi:10.1111/j.1749-6632.2009.05300.x"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$source$source_Lange$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_nocturnal_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$id
#> [1] "assertion_sleep_debt"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$label
#> [1] "Sleep deprivation results in a sleep debt, which has unhealthy consequences."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$id
#> [1] "source_Burgess"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$label
#> [1] "Burgess et al (2002) ). Bright light, dark and melatonin can promote circadian adaptation in night shift workers."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$xdoi
#> [1] "doi:10.1053/smrv.2001.0215"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$source$source_Burgess$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$assertion$assertion_sleep_debt$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_05$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$id
#> [1] "justification_07"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$label
#> [1] "Sleep deprivation causes impaired decision making."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$id
#> [1] "assertion_SD_decision"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$label
#> [1] "Sleep deprivation affects the decision making proces."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$id
#> [1] "source_Harrison"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$label
#> [1] "Harrison & Horne (2000) The impact of sleep deprivation on decision making: A review."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$xdoi
#> [1] "doi:10.1037/1076-898x.6.3.236"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$source$source_Harrison$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$assertion$assertion_SD_decision$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_07$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$id
#> [1] "justification_10"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$label
#> [1] "Sleep deprivation affects emotional perception and experience."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$id
#> [1] "assertion_SD_emotion_1"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$label
#> [1] "Sleep loss enhances the strenth of reactions to negative, but not to positive or neutral stimuli."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$id
#> [1] "assertion_SD_emotion_2"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$label
#> [1] "Humor is negatively evaluated and emotional expression is lost in the voice after sleep deprivation."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$id
#> [1] "source_Killgore13"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$label
#> [1] "Klilgore & Weber (2013) Sleep deprivation and cognitive performance."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$xdoi
#> [1] "doi:10.1007/978-1-4614-9087-6_16"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$source$source_Killgore13$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$assertion$assertion_SD_emotion_2$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$justification$justification_10$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2
#> $supplemented$decisions$decision_to_select_behavior_2$id
#> [1] "decision_to_select_behavior_2"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$label
#> [1] "People should do so and so because of"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$id
#> [1] "justification_01"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$label
#> [1] "Sleep prevents us from getting sick and (therefore) sleep deprivation causes an impaired immune system. Sleep prevents a disbalance of inflammatory cytokines and therefore health is maintained."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$id
#> [1] "assertion_cytokines"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$label
#> [1] "Infection, stress and tissue damage triggers the release of inflammatory cytokines."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$id
#> [1] "source_Frey"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$source$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$id
#> [1] "assertion_cytokines_function"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$label
#> [1] "When pro-inflammatory cytokines are injected, these cytokines enhance sleep."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$id
#> [1] "source_Krueger"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$label
#> [1] "Kreueger et al (2006) The role of cytokines in physiological sleep regulation"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$xdoi
#> [1] "doi:10.1111/j.1749-6632.2001.tb05826.x"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$source$source_Krueger$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_cytokines_function$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$id
#> [1] "assertion_SD_cytokines"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$label
#> [1] "Sleep deprivation is associated with an increase of pro-inflammatory cytokines, which creates a disbalance of inflammatory cytokines and this induces inflammation."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$id
#> [1] "source_Frey"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$label
#> [1] "Frey et al (2007) The effects of 40 hours of total sleep deprivation on inflammatory markers in healthy young adults."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$xdoi
#> [1] "doi:10.1016/j.bbi.2007.04.003"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$source$source_Frey$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$assertion$assertion_SD_cytokines$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_01$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$id
#> [1] "justification_02"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$label
#> [1] "Sleep is important for our memory and (therefore) sleep deprivation causes memory impairment."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$id
#> [1] "assertion_sleep_memory_1"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$source$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_1$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$id
#> [1] "assertion_sleep_memory_2"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$label
#> [1] "Sleep promotes the consolidation of memory in humans."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$id
#> [1] "source_Gais"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$label
#> [1] "Gais (2003) Sleep after learning aids memory recall."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$xdoi
#> [1] "doi:10.1101/lm.132106"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$source$source_Gais$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$id
#> [1] "assertion_sleep_memory_3"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$label
#> [1] "Sleep promotes the consolidation of fear memory in humans."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$id
#> [1] "source_Menz"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$label
#> [1] "Menz et al (2013) The role of sleep and sleep deprivation in consolidating fear memories."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$xdoi
#> [1] "doi:10.1016/j.neuroimage.2013.03.001"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$source$source_Menz$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_3$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$id
#> [1] "assertion_sleep_memory_4"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$label
#> [1] "Sleep promotes the consolidation of emotional memory in humans."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$id
#> [1] "source_Holland"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$label
#> [1] "Holland & Lewis (2007) Emotional memory: selective enhancement by sleep."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$xdoi
#> [1] "doi:10.1016/j.cub.2006.12.033"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$source$source_Holland$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_4$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$id
#> [1] "assertion_sleep_memory_5"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$label
#> [1] "Sleep promotes the consolidation of memory in animals after training for a specific task."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$id
#> [1] "source_Graves"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$label
#> [1] "Graves (2003) Sleep deprivation selectively impairs memory consolidation for contextual fear conditioning."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$xdoi
#> [1] "doi:10.1101/lm.48803"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$source$source_Graves$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_5$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$id
#> [1] "assertion_sleep_memory_6"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$label
#> [1] "Memory retention is already noticable after only several minutes of sleep."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$id
#> [1] "source_Diekelmann"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$label
#> [1] "Diekelmann & Born (2010) The memory function of sleep."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$xdoi
#> [1] "doi:10.1038/nrn2762"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$type
#> [1] "Journal article"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$comment
#> [1] "test of a comment"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$source$source_Diekelmann$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$assertion$assertion_sleep_memory_6$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$justification$justification_02$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$decision_to_select_behavior_2$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$fromDir
#> [1] "."
#>
#> $supplemented$decisions$decision_to_select_behavior_2$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$decision_to_select_behavior_2$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$research_question
#> $supplemented$decisions$research_question$type
#> [1] "research_question"
#>
#> $supplemented$decisions$research_question$id
#> [1] "research_question"
#>
#> $supplemented$decisions$research_question$value
#> [1] "What are the most important determinants of getting one's ecstasy tested?"
#>
#> $supplemented$decisions$research_question$label
#> [1] "The answer to this research question is required to develop and effective interventions to promote ecstasy pill testing."
#>
#> $supplemented$decisions$research_question$description
#> [1] "To minimize the likelihood of incidents and accidental intoxication, testing is a required step."
#>
#> $supplemented$decisions$research_question$justification
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$id
#> [1] "without_testing_users_may_ingest_contaminants"
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$label
#> [1] "Some XTC pills are contaminated, and one needs to test them to be aware of the pill contents."
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$id
#> [1] "some_pills_are_contaminated"
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$source
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$source$vogels_2009
#> NULL
#>
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$label
#> [1] "Not all produced XTC pills contain only MDMA as active ingredient."
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$fromDir
#> [1] "."
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$assertion$some_pills_are_contaminated$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$fromDir
#> [1] "."
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$research_question$justification$without_testing_users_may_ingest_contaminants$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$id
#> [1] "proper_dosing_requires_knowing_dose"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$label
#> [1] "Proper dosing (~ 1-1.5 mg of MDMA per kg of body weight) becomes hard if the dose in a pill is unknown."
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$id
#> [1] "dosing_requires_pill_content"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$label
#> [1] "Determining the dose of MDMA one uses requires knowing both one's body weight and the pill dose."
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$id
#> [1] "brunt_2012"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$label
#> [1] "Brunt, Koeter, Niesink & van den Brink (2012)"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$fromDir
#> [1] "."
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$date
#> [1] "2019-03-06"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$scores
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$source$brunt_2012$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$fromDir
#> [1] "."
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$assertion$dosing_requires_pill_content$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$fromDir
#> [1] "."
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$research_question$justification$proper_dosing_requires_knowing_dose$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$research_question$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$research_question$fromDir
#> [1] "."
#>
#> $supplemented$decisions$research_question$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$research_question$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$global_study_method
#> $supplemented$decisions$global_study_method$type
#> [1] "global_study_method"
#>
#> $supplemented$decisions$global_study_method$id
#> [1] "global_study_method"
#>
#> $supplemented$decisions$global_study_method$value
#> [1] "quantitative"
#>
#> $supplemented$decisions$global_study_method$label
#> [1] "We will conduct a quantitative study."
#>
#> $supplemented$decisions$global_study_method$description
#> [1] "Here, a decision can be explained more in detail (e.g. describing how the justifications relate to each other)."
#>
#> $supplemented$decisions$global_study_method$justification
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$id
#> [1] "enough_known_about_testing_determinants"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$label
#> [1] "We have enough information available to develop a questionnaire that is likely to measure the most important determinants and sub-determinants of XTC testing."
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$id
#> [1] "testing_xtc_is_reasoned"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$source
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$source$phd_peters_2008
#> NULL
#>
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$label
#> [1] "Previous research indicates that getting one's ecstasy tested (or not) is largely a reasoned behavior"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$fromDir
#> [1] "."
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$testing_xtc_is_reasoned$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$id
#> [1] "there_is_qualitative_research_about_testing"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$source
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$source$phd_peters_2008
#> NULL
#>
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$label
#> [1] "There exists qualitative research about why people get their ecstasy tested"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$fromDir
#> [1] "."
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$assertion$there_is_qualitative_research_about_testing$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$fromDir
#> [1] "."
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$global_study_method$justification$enough_known_about_testing_determinants$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$global_study_method$justification$limited_time
#> $supplemented$decisions$global_study_method$justification$limited_time$id
#> [1] "limited_time"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$label
#> [1] "We have to finish this study before the end of 2019."
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$id
#> [1] "project_deadline"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$label
#> [1] "The deadline for this project is december 2019."
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal$id
#> [1] "project_proposal"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal$year
#> [1] 2017
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal$fromDir
#> [1] "."
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$source$project_proposal$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$fromDir
#> [1] "."
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$assertion$project_deadline$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$fromDir
#> [1] "."
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$global_study_method$justification$limited_time$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$global_study_method$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$global_study_method$fromDir
#> [1] "."
#>
#> $supplemented$decisions$global_study_method$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$global_study_method$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$study_sample_size
#> $supplemented$decisions$study_sample_size$id
#> [1] "study_sample_size"
#>
#> $supplemented$decisions$study_sample_size$type
#> [1] "study_sample_size"
#>
#> $supplemented$decisions$study_sample_size$value
#> [1] 400
#>
#> $supplemented$decisions$study_sample_size$label
#> [1] "We aim to recruit around 400 participants."
#>
#> $supplemented$decisions$study_sample_size$justification
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$id
#> [1] "aipe_for_correlations"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$label
#> [1] "To estimate a correlation accurately, you need ~ 400 participants."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$id
#> [1] "nice_round_number"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$label
#> [1] "We want to recruit a nice round number of participants."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$id
#> [1] "nice_round_numbers_are_nice"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$year
#> [1] 219
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$label
#> [1] "In our meeting of 2019-07-05, we agreed that all team members like nice round numbers."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$evidence_type
#> [1] "team opinion"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$fromDir
#> [1] "."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$date
#> [1] "2019-03-06"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$scores
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$source$nice_round_numbers_are_nice$scores$evidence_type
#> [1] 1
#>
#>
#>
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$fromDir
#> [1] "."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$nice_round_number$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$id
#> [1] "exact_aipe_for_our_study"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$label
#> [1] "Table 1 shows that 383 participants are sufficient even for correlations as low as .05."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$id
#> [1] "moinester_gottfried_2014"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$year
#> [1] 2014
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$label
#> [1] "Moinester, M., & Gottfried, R. (2014). Sample size estimation for correlations with pre-specified confidence interval. The Quantitative Methods of Psychology, 10(2), 124–130."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$evidence_type
#> [1] "theory"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$fromDir
#> [1] "."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$date
#> [1] "2019-03-06"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$scores
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$source$moinester_gottfried_2014$scores$evidence_type
#> [1] 4
#>
#>
#>
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$fromDir
#> [1] "."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$assertion$exact_aipe_for_our_study$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$fromDir
#> [1] "."
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$study_sample_size$justification$aipe_for_correlations$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$study_sample_size$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$study_sample_size$fromDir
#> [1] "."
#>
#> $supplemented$decisions$study_sample_size$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$study_sample_size$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$preregistration
#> $supplemented$decisions$preregistration$id
#> [1] "preregistration"
#>
#> $supplemented$decisions$preregistration$type
#> [1] "preregistration"
#>
#> $supplemented$decisions$preregistration$value
#> [1] FALSE
#>
#> $supplemented$decisions$preregistration$label
#> [1] "We will not preregister this study."
#>
#> $supplemented$decisions$preregistration$justification
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$id
#> [1] "low_risk_of_bias"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$label
#> [1] "Because we do not test a specific hypothesis but simply want to know how relevant the different determinants are in this population, the need to preregister is less pressing."
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$id
#> [1] "prereg_to_decrease_bias"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$label
#> [1] "Preregistration_decreases_bias"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$id
#> [1] "example_source"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$label
#> [1] "Not online now, but a study making this point would be nice here"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$evidence_type
#> [1] "empirical study"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$fromDir
#> [1] "."
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$date
#> [1] "2019-03-06"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$scores
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$source$example_source$scores$evidence_type
#> [1] 5
#>
#>
#>
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$fromDir
#> [1] "."
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$assertion$prereg_to_decrease_bias$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$fromDir
#> [1] "."
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$preregistration$justification$low_risk_of_bias$date
#> [1] "2019-03-06"
#>
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$id
#> [1] "no_time_for_prereg"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$label
#> [1] "We have no time to complete a preregistration form."
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$id
#> [1] "project_deadline"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$label
#> [1] "The deadline for this project is december 2019."
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal$id
#> [1] "project_proposal"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal$label
#> [1] "The original proposal for this project as funded by our funder, see the document."
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal$year
#> [1] 2017
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal$fromDir
#> [1] "."
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$source$project_proposal$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$fromDir
#> [1] "."
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$assertion$project_deadline$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$fromDir
#> [1] "."
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$preregistration$justification$no_time_for_prereg$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$decisions$preregistration$fromFile
#> [1] "none"
#>
#> $supplemented$decisions$preregistration$fromDir
#> [1] "."
#>
#> $supplemented$decisions$preregistration$framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $supplemented$decisions$preregistration$date
#> [1] "2019-03-06"
#>
#>
#>
#> $supplemented$justifier
#> $supplemented$justifier$justifier_example_study_framework_specification
#> $id
#> [1] "justifier_example_study_framework_specification"
#>
#> $label
#> [1] "Justifier example: Study framework specification"
#>
#> $description
#> [1] "This framework is an example of how different decisions in the planning of a psychological study could be contextualized."
#>
#> $context
#> $context[[1]]
#> $context[[1]]$id
#> [1] "global_planning"
#>
#> $context[[1]]$importedContextFramework
#> [1] "some_other_framework_specification"
#>
#> $context[[1]]$importedContextId
#> [1] "global_planning"
#>
#> $context[[1]]$sequence
#> [1] 1
#>
#>
#> $context[[2]]
#> $context[[2]]$id
#> [1] "design"
#>
#> $context[[2]]$sequence
#> [1] 2
#>
#> $context[[2]]$label
#> [1] "Decisions related to design"
#>
#>
#> $context[[3]]
#> $context[[3]]$id
#> [1] "sample_size"
#>
#> $context[[3]]$sequence
#> [1] 3
#>
#>
#> $context[[4]]
#> $context[[4]]$id
#> [1] "operationalizations"
#>
#> $context[[4]]$sequence
#> [1] 4
#>
#>
#>
#> $condition
#> $condition[[1]]
#> $condition[[1]]$id
#> [1] "global_method_specification"
#>
#> $condition[[1]]$contextId
#> [1] "global_planning"
#>
#> $condition[[1]]$type
#> [1] "global_method_specification"
#>
#> $condition[[1]]$element
#> [1] "decision"
#>
#> $condition[[1]]$label
#> [1] "Any psychological study is either empirical, in which case it is a qualitative study, a quantitative study, or a mixed methods study, or it is a literature synthesis."
#>
#> $condition[[1]]$values
#> [1] "synthesis" "qualitative" "quantitative" "mixed"
#>
#>
#> $condition[[2]]
#> $condition[[2]]$id
#> [1] "study_sample_size"
#>
#> $condition[[2]]$contextId
#> [1] "sample_size"
#>
#> $condition[[2]]$type
#> [1] "study_sample_size"
#>
#> $condition[[2]]$element
#> [1] "decision"
#>
#> $condition[[2]]$verifications
#> [1] "is.numeric(THIS_SPECIFICATION$value)"
#>
#>
#> $condition[[3]]
#> $condition[[3]]$id
#> [1] "included_variables"
#>
#> $condition[[3]]$contextid
#> [1] "design"
#>
#> $condition[[3]]$type
#> [1] "included_variables"
#>
#> $condition[[3]]$element
#> [1] "decision"
#>
#>
#> $condition[[4]]
#> $condition[[4]]$id
#> [1] "source_types"
#>
#> $condition[[4]]$element
#> [1] "source"
#>
#> $condition[[4]]$field
#> [1] "evidence_type"
#>
#> $condition[[4]]$values
#> [1] "team opinion" "external expert opinion"
#> [3] "external expert consensus" "theory"
#> [5] "empirical study" "evidence synthesis"
#>
#> $condition[[4]]$scores
#> [1] 1 2 3 4 5 6
#>
#> $condition[[4]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$evidence_type) || (THIS_SPECIFICATION$evidence_type %in% THIS_CONDITION$values)"
#>
#>
#> $condition[[5]]
#> $condition[[5]]$id
#> [1] "source_year"
#>
#> $condition[[5]]$element
#> [1] "source"
#>
#> $condition[[5]]$field
#> [1] "year"
#>
#> $condition[[5]]$verifications
#> [1] "is.null(THIS_SPECIFICATION$year) || all(grepl('^[0-9]{4}$', THIS_SPECIFICATION$year) & (nchar(THIS_SPECIFICATION$year)==4))"
#>
#> $condition[[5]]$verificationMsgs
#> [1] "The year you specify in a source must consist of exactly four digits."
#>
#>
#>
#> $fromFile
#> [1] "none"
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#> $supplemented$justifier[[2]]
#> $scope
#> [1] "global"
#>
#> $framework
#> [1] "justifier-example-study-framework-specification.jmd"
#>
#> $fromFile
#> [1] "none"
#>
#> $id
#> [1] ""
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#> $supplemented$justifier[[3]]
#> $scope
#> [1] "local"
#>
#> $date
#> [1] "2019-03-06"
#>
#> $fromFile
#> [1] "none"
#>
#> $id
#> [1] ""
#>
#> $fromDir
#> [1] "."
#>
#> attr(,"class")
#> [1] "justifierSpec" "justifier"
#>
#>
#>
#> $decisionTrees
#> $decisionTrees$decision_1
#> levelName
#> 1 decision_1
#> 2 ¦--justification_1
#> 3 ¦ ¦--assertion_1
#> 4 ¦ ¦ ¦--source_1
#> 5 ¦ ¦ °--source_2
#> 6 ¦ °--assertion_2
#> 7 ¦ °--source_3
#> 8 °--justification_2
#> 9 °--assertion_2
#> 10 °--source_3
#>
#> $decisionTrees$decision_2
#> levelName
#> 1 decision_2
#>
#> $decisionTrees$decision_to_select_behavior_1
#> levelName
#> 1 decision_to_select_behavior_1
#> 2 ¦--justification_04
#> 3 ¦ ¦--assertion_resting_1
#> 4 ¦ ¦ °--source_Brokaw
#> 5 ¦ ¦--assertion_resting_2
#> 6 ¦ ¦ °--source_Marrosu
#> 7 ¦ °--assertion_resting_3
#> 8 ¦ °--source_Tononi
#> 9 ¦--justification_05
#> 10 ¦ ¦--assertion_nocturnal_1
#> 11 ¦ ¦ °--source_Lange
#> 12 ¦ ¦--assertion_nocturnal_2
#> 13 ¦ ¦ °--source_Lange
#> 14 ¦ °--assertion_sleep_debt
#> 15 ¦ °--source_Burgess
#> 16 ¦--justification_07
#> 17 ¦ °--assertion_SD_decision
#> 18 ¦ °--source_Harrison
#> 19 °--justification_10
#> 20 ¦--assertion_SD_emotion_1
#> 21 ¦ °--source_Killgore13
#> 22 °--assertion_SD_emotion_2
#> 23 °--source_Killgore13
#>
#> $decisionTrees$decision_to_select_behavior_2
#> levelName
#> 1 decision_to_select_behavior_2
#> 2 ¦--justification_01
#> 3 ¦ ¦--assertion_cytokines
#> 4 ¦ ¦ °--source_Frey
#> 5 ¦ ¦--assertion_cytokines_function
#> 6 ¦ ¦ °--source_Krueger
#> 7 ¦ °--assertion_SD_cytokines
#> 8 ¦ °--source_Frey
#> 9 °--justification_02
#> 10 ¦--assertion_sleep_memory_1
#> 11 ¦ °--source_Diekelmann
#> 12 ¦--assertion_sleep_memory_2
#> 13 ¦ °--source_Gais
#> 14 ¦--assertion_sleep_memory_3
#> 15 ¦ °--source_Menz
#> 16 ¦--assertion_sleep_memory_4
#> 17 ¦ °--source_Holland
#> 18 ¦--assertion_sleep_memory_5
#> 19 ¦ °--source_Graves
#> 20 °--assertion_sleep_memory_6
#> 21 °--source_Diekelmann
#>
#> $decisionTrees$research_question
#> levelName
#> 1 research_question
#> 2 ¦--without_testing_users_may_ingest_contaminants
#> 3 ¦ °--some_pills_are_contaminated
#> 4 ¦ °--1
#> 5 °--proper_dosing_requires_knowing_dose
#> 6 °--dosing_requires_pill_content
#> 7 °--brunt_2012
#>
#> $decisionTrees$global_study_method
#> levelName
#> 1 global_study_method
#> 2 ¦--enough_known_about_testing_determinants
#> 3 ¦ ¦--testing_xtc_is_reasoned
#> 4 ¦ ¦ °--1
#> 5 ¦ °--there_is_qualitative_research_about_testing
#> 6 ¦ °--1
#> 7 °--limited_time
#> 8 °--project_deadline
#> 9 °--project_proposal
#>
#> $decisionTrees$study_sample_size
#> levelName
#> 1 study_sample_size
#> 2 °--aipe_for_correlations
#> 3 ¦--nice_round_number
#> 4 ¦ °--nice_round_numbers_are_nice
#> 5 °--exact_aipe_for_our_study
#> 6 °--moinester_gottfried_2014
#>
#> $decisionTrees$preregistration
#> levelName
#> 1 preregistration
#> 2 ¦--low_risk_of_bias
#> 3 ¦ °--prereg_to_decrease_bias
#> 4 ¦ °--example_source
#> 5 °--no_time_for_prereg
#> 6 °--project_deadline
#> 7 °--project_proposal
#>
#>
#> $decisionGraphs
#> $decisionGraphs$decision_1
#>
#> $decisionGraphs$decision_2
#>
#> $decisionGraphs$decision_to_select_behavior_1
#>
#> $decisionGraphs$decision_to_select_behavior_2
#>
#> $decisionGraphs$research_question
#>
#> $decisionGraphs$global_study_method
#>
#> $decisionGraphs$study_sample_size
#>
#> $decisionGraphs$preregistration
#>
#>
#> attr(,"class")
#> [1] "justifications"